Mastering Conditional Validation in Laravel with the "sometimes" Rule
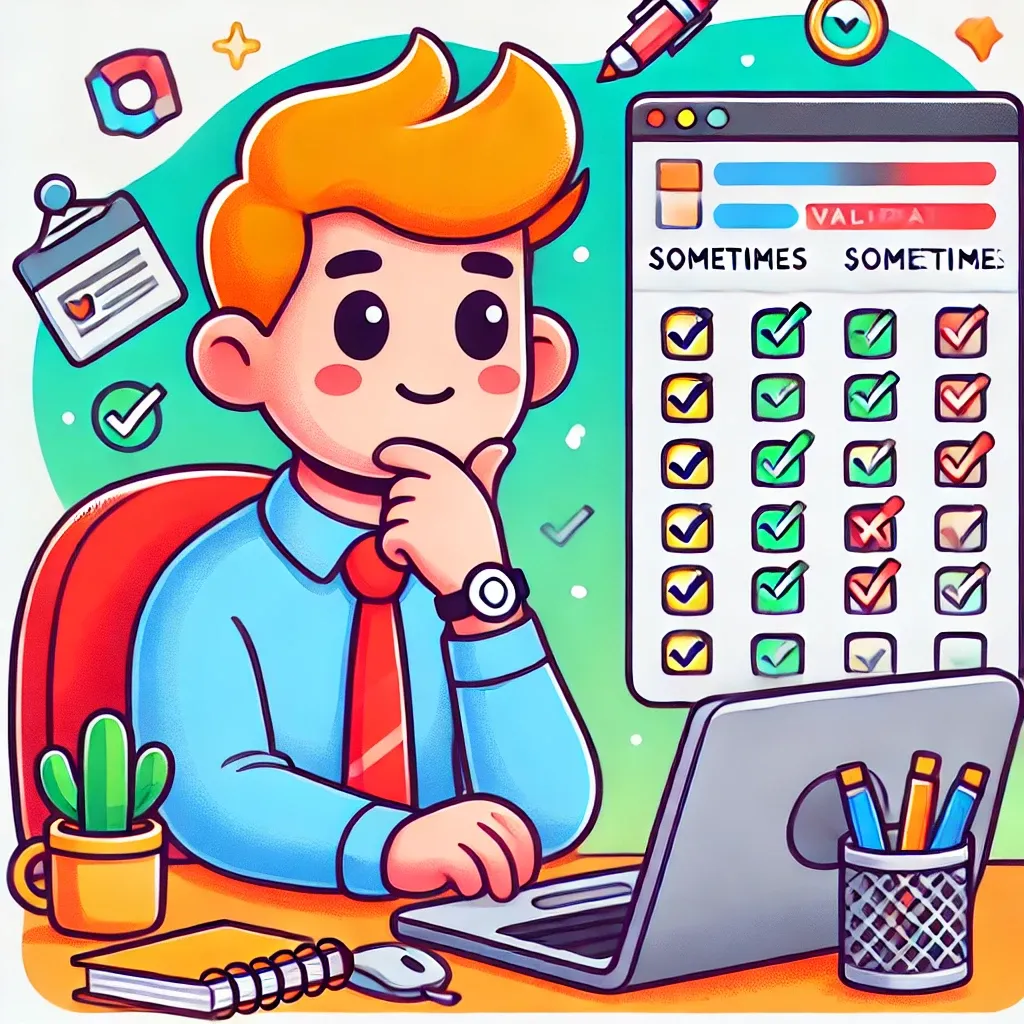
As Laravel developers, we often encounter complex forms where the validation requirements for certain fields depend on the values of others. This is where Laravel's "sometimes" validation rule comes into play. In this post, we'll explore how to use this powerful feature to create more flexible and dynamic form validations.
Understanding the "sometimes" Rule
The "sometimes" rule in Laravel allows you to conditionally apply validation rules to a field. It's particularly useful when a field should only be validated if it's present in the input data or if certain conditions are met.
Basic Usage
Let's start with a simple example:
$validator = Validator::make($request->all(), [
'email' => 'required|email',
'phone' => 'sometimes|required|digits:10',
]);
In this case, the 'phone' field will only be validated if it's present in the input data. If it is present, it must be required and have exactly 10 digits.
Advanced Techniques
Using Closures for Complex Conditions
The real power of "sometimes" comes when you combine it with closures for more complex conditional logic:
$validator = Validator::make($request->all(), [
'games' => 'required|in:yes,no',
'reason' => 'sometimes|required|max:500',
]);
$validator->sometimes('reason', 'required|max:500', function ($input) {
return $input->games === 'no';
});
Here, the 'reason' field is only required if the user selects 'no' for the 'games' field.
Multiple Fields and Rules
You can apply "sometimes" to multiple fields and rules:
$validator->sometimes(['address', 'city', 'state'], 'required', function ($input) {
return $input->country === 'US';
});
This makes all address fields required only if the country is set to 'US'.
Real-World Example: Event Registration Form
Let's consider a more complex scenario of an event registration form:
use Illuminate\Support\Facades\Validator;
class EventRegistrationController extends Controller
{
public function store(Request $request)
{
$validator = Validator::make($request->all(), [
'name' => 'required|string|max:255',
'email' => 'required|email',
'event_type' => 'required|in:conference,workshop,webinar',
'dietary_requirements' => 'sometimes|required|string|max:255',
'workshop_choice' => 'sometimes|required|exists:workshops,id',
'company' => 'sometimes|required|string|max:255',
'job_title' => 'sometimes|required|string|max:255',
]);
$validator->sometimes('dietary_requirements', 'required', function ($input) {
return in_array($input->event_type, ['conference', 'workshop']);
});
$validator->sometimes('workshop_choice', 'required', function ($input) {
return $input->event_type === 'workshop';
});
$validator->sometimes(['company', 'job_title'], 'required', function ($input) {
return $input->event_type === 'conference';
});
if ($validator->fails()) {
return redirect()->back()->withErrors($validator)->withInput();
}
// Registration logic here...
}
}
In this example:
- Dietary requirements are only required for in-person events (conference or workshop).
- Workshop choice is only required if the event type is a workshop.
- Company and job title are only required for conference registrations.
Best Practices
- Keep It Readable: While powerful, complex conditional validations can become hard to read. Consider breaking them into smaller, more manageable pieces.
- Use Form Requests: For very complex validations, consider moving the logic into a Form Request class to keep your controllers clean.
- Combine with Other Rules: "sometimes" works well with other Laravel validation features, allowing for highly customized validation logic.
- Error Messages: Remember to provide clear error messages for conditional fields to avoid user confusion.
- Testing: Thoroughly test your conditional validations to ensure they behave correctly under all scenarios.
Conclusion
The "sometimes" validation rule in Laravel provides a powerful way to implement conditional validation logic in your forms. By leveraging this feature, you can create more dynamic and user-friendly forms that adapt to user input and specific scenarios.
Whether you're building a complex registration system, a multi-step form, or any application that requires contextual data validation, mastering the "sometimes" rule will significantly enhance your ability to create robust and flexible Laravel applications. Remember, the goal is not just to validate data, but to create a smooth and logical user experience that guides users through your forms effectively.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!