Mastering Conditional Logic with Laravel's unless Method
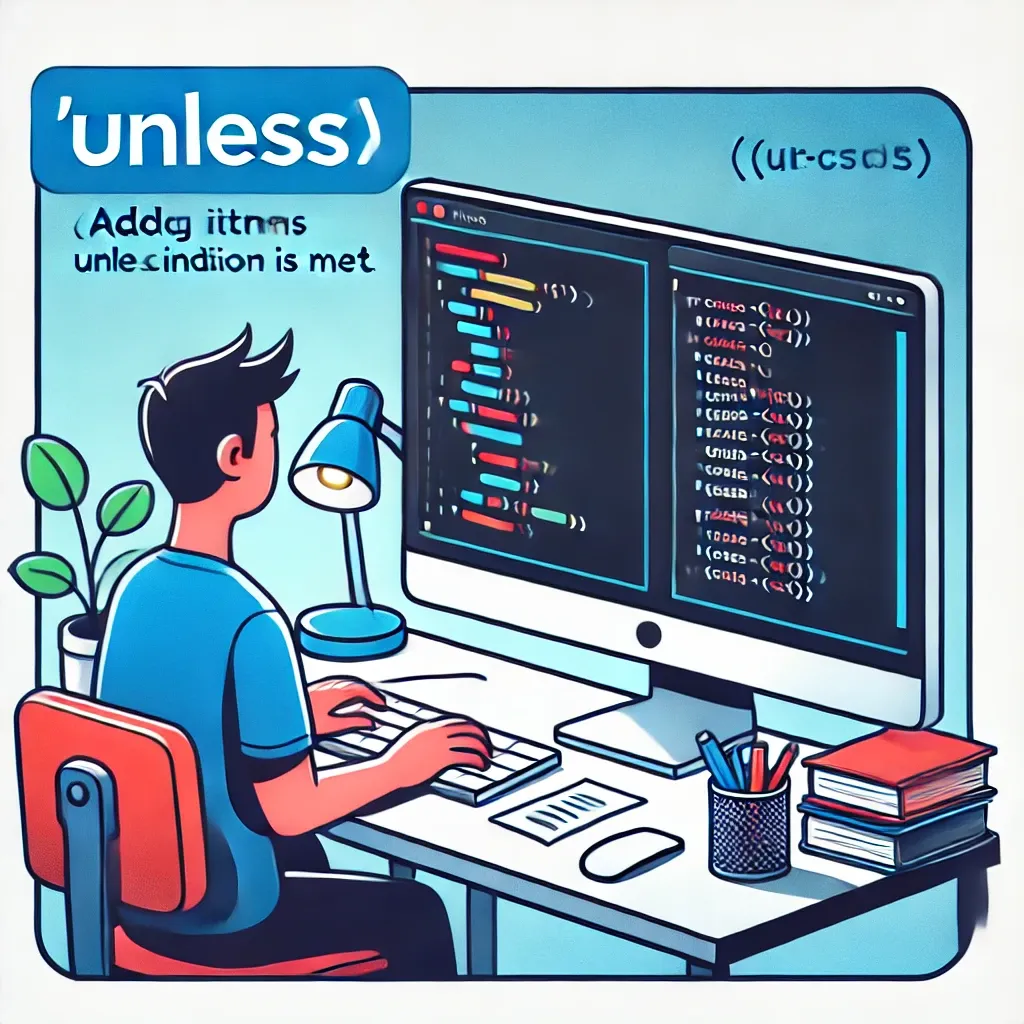
Laravel's Collection class offers a variety of methods to manipulate and process data. Among these, the unless
method stands out as a powerful tool for implementing conditional logic in a clean and expressive way. Let's dive into how this method works and explore its practical applications in Laravel development.
Understanding unless
The unless
method allows you to execute a given callback unless a specified condition evaluates to true. It's essentially the inverse of the when
method, providing a more readable way to express negative conditions.
Basic Usage
Here's a simple example to illustrate how unless
works:
$collection = collect([1, 2, 3]);
$result = $collection->unless(true, function ($collection) {
return $collection->push(4);
});
// Result: [1, 2, 3]
$result = $collection->unless(false, function ($collection) {
return $collection->push(5);
});
// Result: [1, 2, 3, 5]
In this example, the first unless
call doesn't modify the collection because the condition is true. The second call adds an element because the condition is false.
Advanced Usage
The unless
method also accepts a second callback, which is executed when the condition is true:
$collection = collect([1, 2, 3]);
$result = $collection->unless(
$collection->isEmpty(),
function ($collection) {
return $collection->push(4);
},
function ($collection) {
return $collection->push(0);
}
);
// Result: [1, 2, 3, 4]
Practical Applications
Conditional Data Transformation
Imagine you're processing user data and want to apply a transformation only if certain conditions are not met:
$users = collect([
['name' => 'John', 'active' => true],
['name' => 'Jane', 'active' => false],
['name' => 'Bob', 'active' => true]
]);
$processedUsers = $users->map(function ($user) {
return $this->unless($user['active'], function () use ($user) {
$user['name'] = strtoupper($user['name']);
return $user;
}, function () use ($user) {
return $user;
});
});
// Result: [
// ['name' => 'John', 'active' => true],
// ['name' => 'JANE', 'active' => false],
// ['name' => 'Bob', 'active' => true]
// ]
Conditional API Responses
When building APIs, you might want to include additional data only under certain conditions:
public function show(User $user)
{
$response = [
'id' => $user->id,
'name' => $user->name,
'email' => $user->email,
];
return collect($response)->unless(
!auth()->user()->isAdmin(),
function ($collection) use ($user) {
return $collection->merge([
'last_login' => $user->last_login,
'created_at' => $user->created_at,
]);
}
);
}
Conditional Database Queries
You can use unless
to conditionally add query constraints:
$query = User::query();
$activeOnly = false;
$users = collect([$query])->unless($activeOnly, function ($collection) {
return $collection->map(function ($query) {
return $query->where('active', true);
});
})->first()->get();
Combining with Other Collection Methods
The unless
method can be chained with other collection methods for more complex logic:
$numbers = collect([1, 2, 3, 4, 5]);
$result = $numbers
->unless($numbers->contains(6), function ($collection) {
return $collection->push(6);
})
->map(function ($number) {
return $number * 2;
});
// Result: [2, 4, 6, 8, 10, 12]
The unless
method in Laravel's Collection class provides a clean and expressive way to implement conditional logic. By allowing you to clearly express negative conditions, it can make your code more readable and intuitive. Whether you're transforming data, building API responses, or constructing complex queries, unless
offers a powerful tool for handling conditional operations in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!