Mastering concurrency with Laravel's Cache::lock()
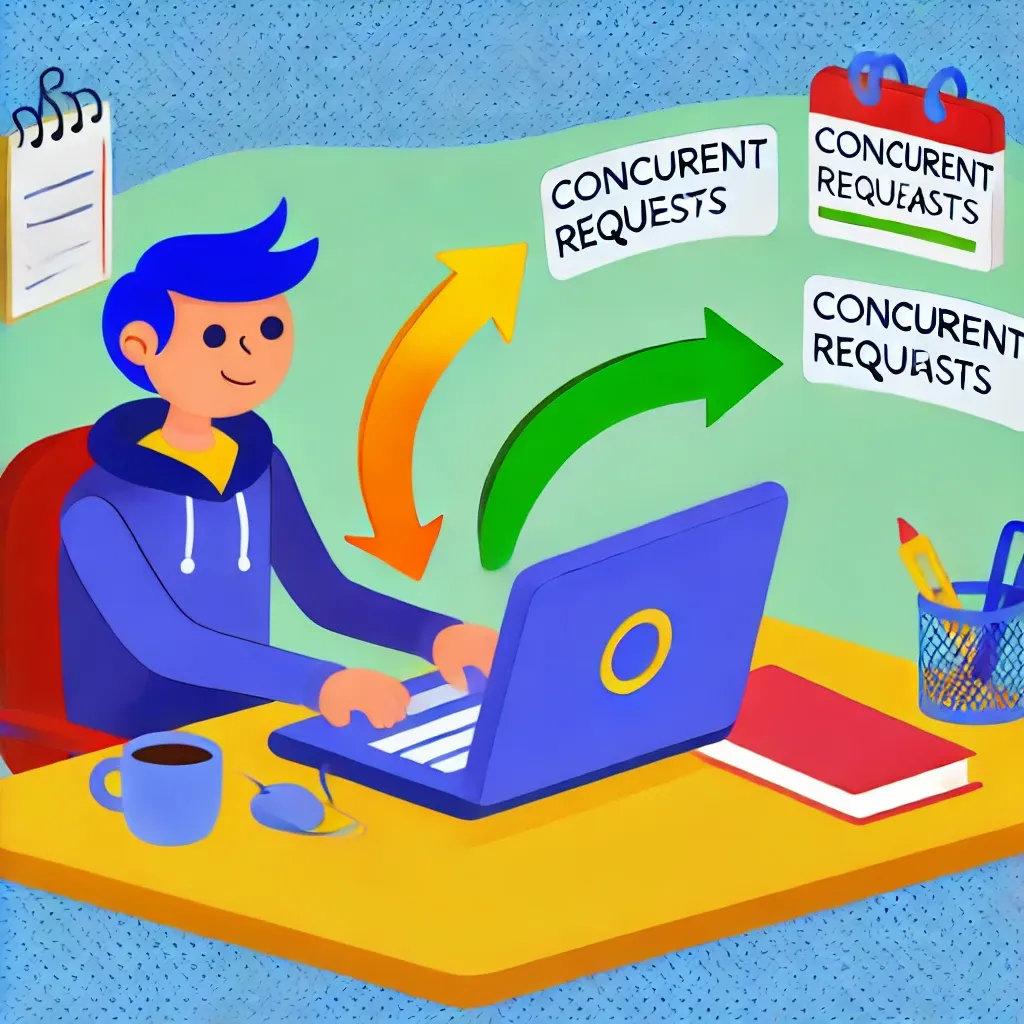
In the world of web development, managing concurrent operations can be a significant challenge. Race conditions, where multiple processes compete to modify shared resources, can lead to data inconsistencies and bugs that are difficult to track down. Fortunately, Laravel provides a powerful tool to help manage these scenarios: Cache::lock(). In this post, we'll explore how to use this feature to ensure the integrity of your application's critical operations.
What is Cache::lock()?
Cache::lock() is a method provided by Laravel that allows you to create a distributed lock. This lock ensures that only one process can execute a particular piece of code at any given time, even across multiple servers or worker processes.
How to Use Cache::lock()
Here's a basic example of how to use Cache::lock():
use Illuminate\Support\Facades\Cache;
class OrderProcessor
{
public function processOrders()
{
$lock = Cache::lock('process-orders', 10);
if ($lock->get()) {
try {
// Process orders here
$this->processOrderQueue();
} finally {
$lock->release();
}
} else {
// Could not obtain lock
Log::info('Order processing is already in progress.');
}
}
private function processOrderQueue()
{
// Implementation of order processing
}
}
Let's break this down:
- We create a lock named 'process-orders' that will last for 10 seconds.
- We attempt to acquire the lock using $lock->get().
- If we get the lock, we process the orders and then release the lock in a finally block to ensure it's always released, even if an exception occurs.
- If we can't get the lock, we log a message indicating that order processing is already in progress.
Benefits of Using Cache::lock()
- Prevent Race Conditions: Ensure that critical operations are not executed concurrently, preventing data corruption.
- Improved Reliability: Avoid duplicate processing in distributed systems.
- Flexibility: Locks can be used with different cache drivers, including Redis and Memcached.
- Automatic Release: Locks are automatically released after their specified duration, preventing indefinite locks if a process fails.
Real-World Example: Scheduled Task Management
Let's consider a more complex real-world scenario where Cache::lock() can be incredibly useful: managing scheduled tasks in a multi-server environment.
use Illuminate\Support\Facades\Cache;
use Illuminate\Support\Facades\Log;
class DailyReportGenerator
{
public function generate()
{
$lock = Cache::lock('generate-daily-report', 3600); // 1 hour lock
if ($lock->get()) {
try {
Log::info('Starting daily report generation');
// Step 1: Gather data
$data = $this->gatherData();
// Step 2: Process data
$processedData = $this->processData($data);
// Step 3: Generate report
$report = $this->createReport($processedData);
// Step 4: Send report
$this->sendReport($report);
Log::info('Daily report generation completed');
} catch (\Exception $e) {
Log::error('Error generating daily report: ' . $e->getMessage());
} finally {
$lock->release();
}
} else {
Log::info('Daily report generation is already in progress');
}
}
// Other methods implementation...
}
In this example:
- We create a lock that lasts for an hour, which should be more than enough time for the report to generate.
- If we acquire the lock, we proceed with the report generation process, which involves several steps.
- We use a try-catch block to handle any exceptions that might occur during the process.
- Whether the process succeeds or fails, we ensure the lock is released in the finally block.
- If we can't acquire the lock, we log a message indicating that report generation is already in progress.
This approach ensures that even if this task is scheduled to run on multiple servers, only one instance will actually perform the work. This prevents duplicate reports from being generated and sent, which could lead to confusion and wasted resources.
Conclusion
Laravel's Cache::lock() is a powerful tool for managing concurrency in your applications. By providing a simple way to implement distributed locks, it helps prevent race conditions and ensures the integrity of your critical operations.
Remember, while locks are powerful, they should be used judiciously. Always consider the duration of your locks carefully, and be prepared to handle scenarios where a lock can't be acquired. With proper use, Cache::lock() can significantly improve the reliability and consistency of your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!