Mastering Command Signatures in Laravel: A Complete Guide
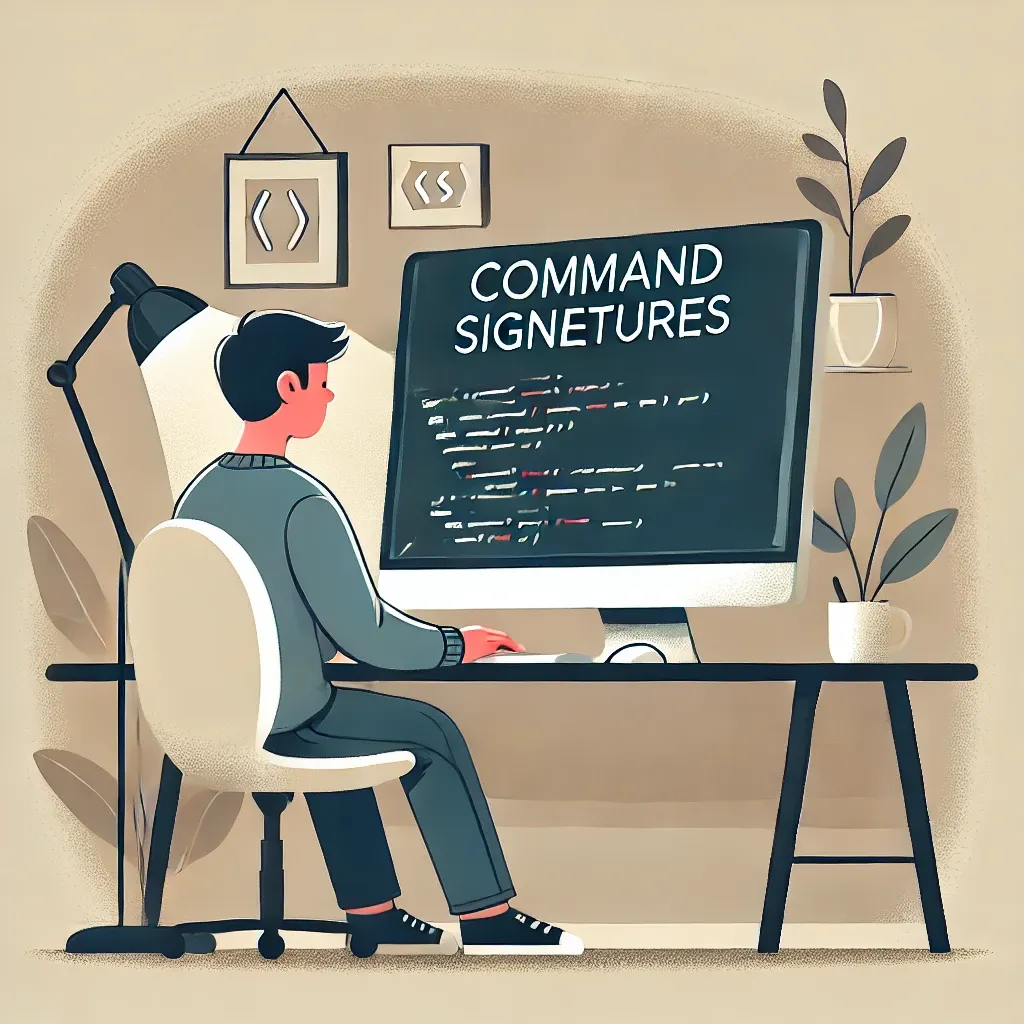
Need to create user-friendly console commands? Laravel's signature syntax makes it a breeze to define arguments and options! Let's explore this powerful feature.
Basic Arguments
The simplest form uses required arguments:
protected $signature = 'mail:send {user}';
You can make arguments optional or set defaults:
// Optional
protected $signature = 'mail:send {user?}';
// With default
protected $signature = 'mail:send {user=john}';
Working with Options
Options provide additional flexibility:
// Boolean switch
protected $signature = 'mail:send {user} {--queue}';
// Option with value
protected $signature = 'mail:send {user} {--queue=}';
// Option with default value
protected $signature = 'mail:send {user} {--queue=default}';
Real-World Example
Here's how you might build an article publishing command:
class PublishArticle extends Command
{
protected $signature = 'blog:publish
{article : The ID of the article to publish}
{--schedule= : Schedule publication for a future date}
{--notify : Send notification to subscribers}
{--channel=website : Publishing channel (website/social)}';
public function handle()
{
$articleId = $this->argument('article');
$schedule = $this->option('schedule');
$shouldNotify = $this->option('notify');
$channel = $this->option('channel');
$this->info("Publishing article #{$articleId}");
if ($schedule) {
$this->info("Scheduled for: {$schedule}");
}
// Publishing logic here...
if ($shouldNotify) {
$this->info('Sending notifications...');
}
$this->info("Published to {$channel}!");
}
}
Usage examples:
# Basic publish
php artisan blog:publish 123
# Scheduled publish with notifications
php artisan blog:publish 123 --schedule="2024-01-01 10:00" --notify
# Publish to social media
php artisan blog:publish 123 --channel=social
Command signatures make it easy to create intuitive, flexible console commands that are a joy to use.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!