Mastering Collection Filtering with Laravel's skipWhile Method
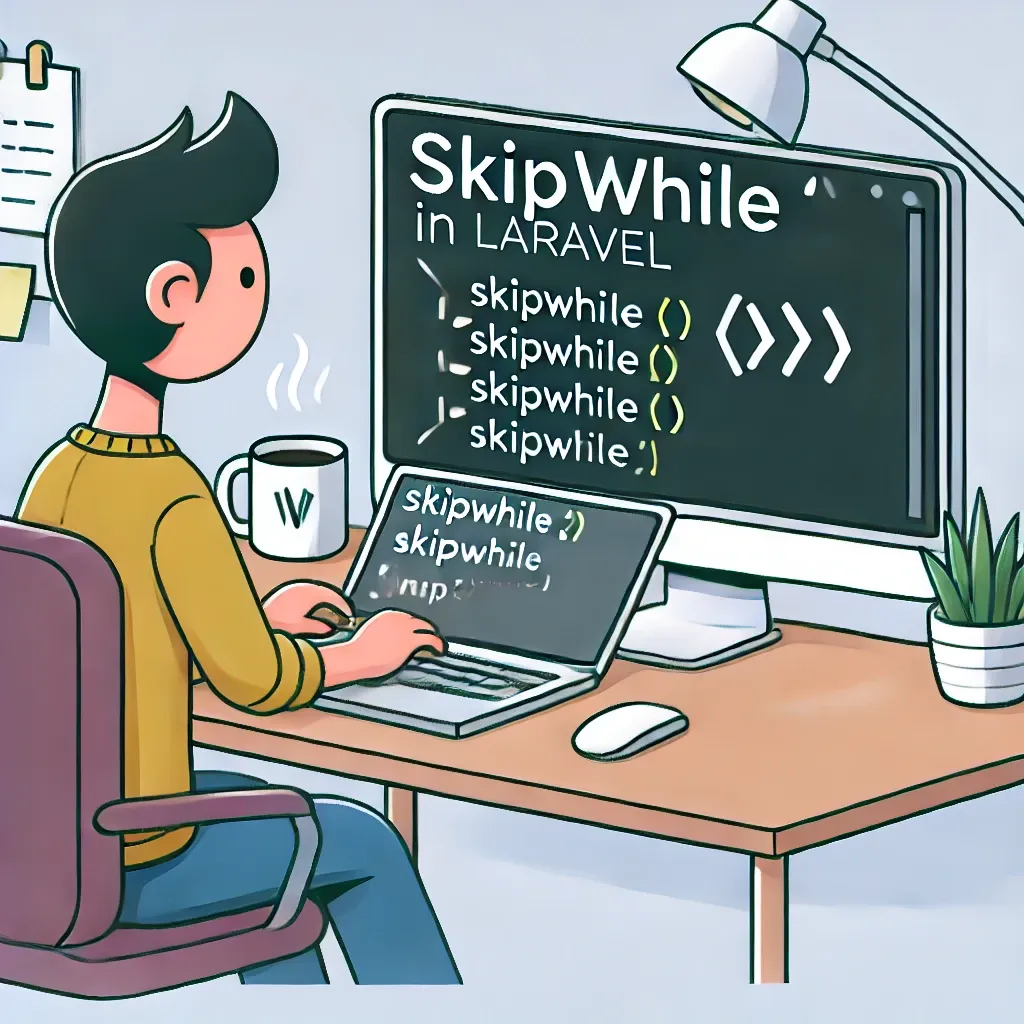
Laravel's Collection class offers a rich set of methods for manipulating arrays and data sets. Among these, the skipWhile
method stands out as a powerful tool for precise filtering of collections. Let's dive into how this method works and how you can leverage it in your Laravel applications.
Understanding skipWhile
The skipWhile
method allows you to skip over items in a collection as long as a given condition is true. Once the condition returns false for an item, that item and all subsequent items are included in the resulting collection.
Basic Usage
Here's a simple example to illustrate how skipWhile
works:
$collection = collect([1, 2, 3, 4, 5, 1, 2, 3]);
$subset = $collection->skipWhile(function ($item) {
return $item < 3;
});
// Result: [3, 4, 5, 1, 2, 3]
In this example, skipWhile
skips over items less than 3. Once it encounters 3, it includes that item and all remaining items, regardless of their value.
Practical Applications
The skipWhile
method can be particularly useful in scenarios where you need to process data streams or filter collections based on dynamic conditions. Here are a few practical applications:
Processing Log Files
Imagine you're analyzing a log file and want to skip entries until a certain timestamp:
$logs = collect([
['time' => '2023-01-01 10:00', 'message' => 'Log 1'],
['time' => '2023-01-01 11:00', 'message' => 'Log 2'],
['time' => '2023-01-01 12:00', 'message' => 'Log 3'],
['time' => '2023-01-01 13:00', 'message' => 'Log 4'],
]);
$relevantLogs = $logs->skipWhile(function ($log) {
return strtotime($log['time']) < strtotime('2023-01-01 12:00');
});
// Result: [
// ['time' => '2023-01-01 12:00', 'message' => 'Log 3'],
// ['time' => '2023-01-01 13:00', 'message' => 'Log 4']
// ]
Data Cleaning
When cleaning data, you might want to skip initial rows that don't meet certain criteria:
$data = collect([
['name' => '', 'email' => ''],
['name' => 'John', 'email' => ''],
['name' => 'Jane', 'email' => 'jane@example.com'],
['name' => 'Bob', 'email' => 'bob@example.com'],
]);
$cleanedData = $data->skipWhile(function ($item) {
return empty($item['name']) || empty($item['email']);
});
// Result: [
// ['name' => 'Jane', 'email' => 'jane@example.com'],
// ['name' => 'Bob', 'email' => 'bob@example.com']
// ]
Financial Data Analysis
In financial applications, you might want to skip over initial losses until you hit the first profit:
$transactions = collect([-100, -50, -20, 30, 50, -10, 100]);
$profitStreak = $transactions->skipWhile(function ($amount) {
return $amount <= 0;
});
// Result: [30, 50, -10, 100]
Combining with Other Collection Methods
skipWhile
can be even more powerful when combined with other collection methods. For example, you can use it with takeWhile
to extract a specific section of a collection:
$numbers = collect([1, 2, 3, 4, 5, 6, 1, 2, 3]);
$middleSection = $numbers->skipWhile(function ($number) {
return $number < 3;
})->takeWhile(function ($number) {
return $number > 2;
});
// Result: [3, 4, 5, 6]
The skipWhile
method in Laravel's Collection class provides a flexible and powerful way to filter collections based on dynamic conditions. Whether you're processing log files, cleaning data sets, or performing complex data analysis, skipWhile
offers a clean and expressive solution for precise data manipulation in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!