Mastering Blade Stacks: Organizing Your Laravel Views with Precision
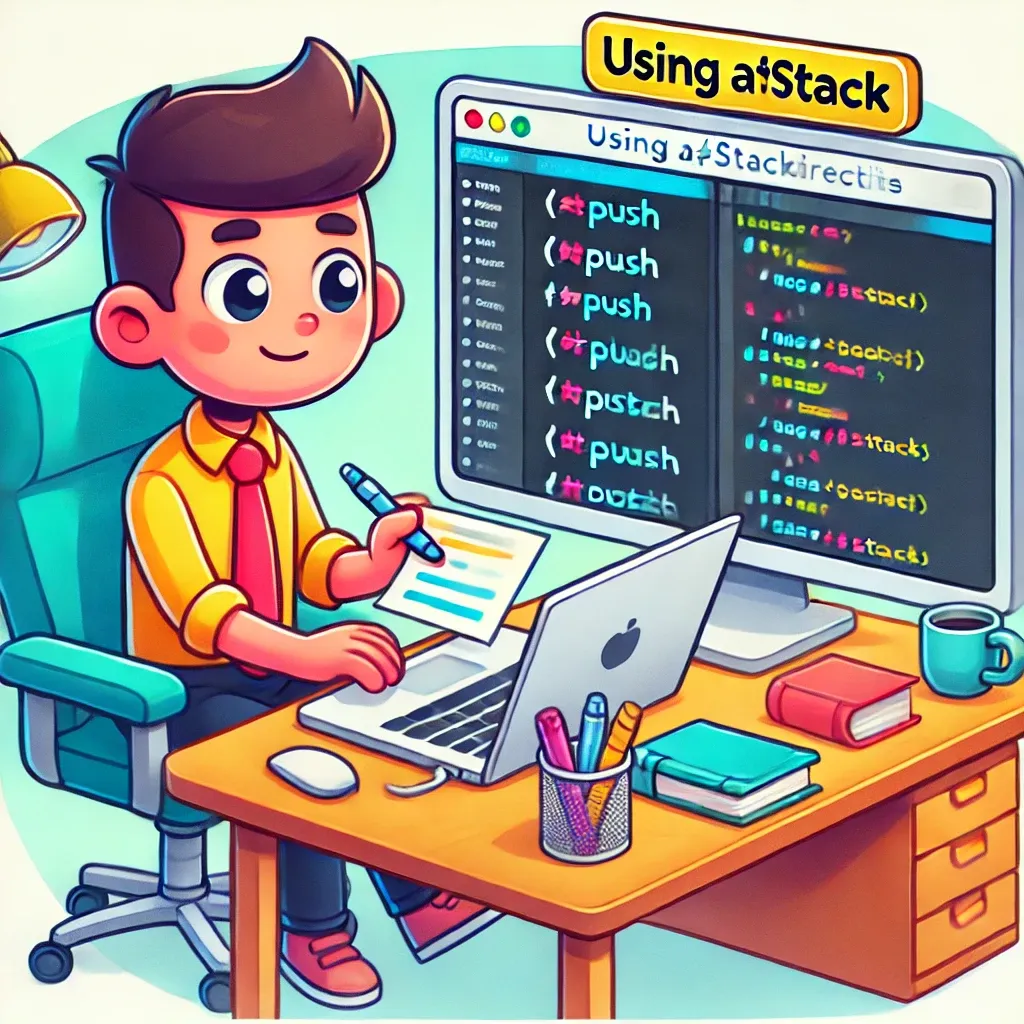
In the world of Laravel development, organizing your views efficiently can significantly improve your code's maintainability and flexibility. One powerful feature that Laravel's Blade templating engine offers for this purpose is "stacks". Let's dive into how you can leverage Blade stacks to create more modular and organized views in your Laravel applications.
Understanding Blade Stacks
Blade stacks allow you to push content into named stacks which can be rendered elsewhere in another view or layout. This is particularly useful for specifying assets like JavaScript or CSS files that a child view may require.
Basic Usage of Blade Stacks
Here's how you can use Blade stacks in your views:
// In a child view
@push('scripts')
<script src="/example.js"></script>
@endpush
// In your layout
<head>
<!-- Head Contents -->
@stack('scripts')
</head>
In this example, the script tag is pushed onto the 'scripts' stack, which is then rendered in the layout.
Advanced Stack Usage
Prepending to Stacks
Sometimes you might want to add content to the beginning of a stack rather than the end. For this, you can use the @prepend
directive:
@prepend('scripts')
<script src="/first-to-load.js"></script>
@endprepend
Conditional Pushing
You can conditionally push content to a stack using the @pushIf
directive:
@pushIf($shouldPushScript, 'scripts')
<script src="/conditional-script.js"></script>
@endPushIf
Real-World Example: A Complex Layout with Multiple Sections
Let's consider a more comprehensive example of how stacks can be used in a real-world scenario:
// resources/views/layouts/app.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>@yield('title', 'My App')</title>
<link rel="stylesheet" href="/app.css">
@stack('styles')
</head>
<body>
<nav>
<!-- Navigation content -->
</nav>
<main>
@yield('content')
</main>
<footer>
<!-- Footer content -->
</footer>
<script src="/app.js"></script>
@stack('scripts')
</body>
</html>
// resources/views/posts/show.blade.php
@extends('layouts.app')
@section('title', 'View Post')
@section('content')
<h1>{{ $post->title }}</h1>
<p>{{ $post->content }}</p>
@endsection
@push('styles')
<link rel="stylesheet" href="/posts.css">
@endpush
@push('scripts')
<script src="/posts.js"></script>
@endpush
// resources/views/posts/create.blade.php
@extends('layouts.app')
@section('title', 'Create Post')
@section('content')
<h1>Create a New Post</h1>
<!-- Post creation form -->
@endsection
@push('styles')
<link rel="stylesheet" href="/markdown-editor.css">
@endpush
@push('scripts')
<script src="/markdown-editor.js"></script>
<script>
initializeMarkdownEditor();
</script>
@endpush
In this example, we have a main layout that defines stacks for both styles and scripts. Different views (like show.blade.php
and create.blade.php
) can then push their specific assets onto these stacks, allowing for a clean and modular structure.
Blade stacks provide a powerful way to organize and manage the inclusion of assets and other content in your Laravel views. By allowing you to push content from child views into predefined locations in your layouts, stacks enable you to create more modular, flexible, and maintainable templates. Whether you're working on a simple blog or a complex web application, mastering Blade stacks can significantly enhance your Laravel development workflow.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!