Mastering API Responses in Laravel: Customizing Resource Responses
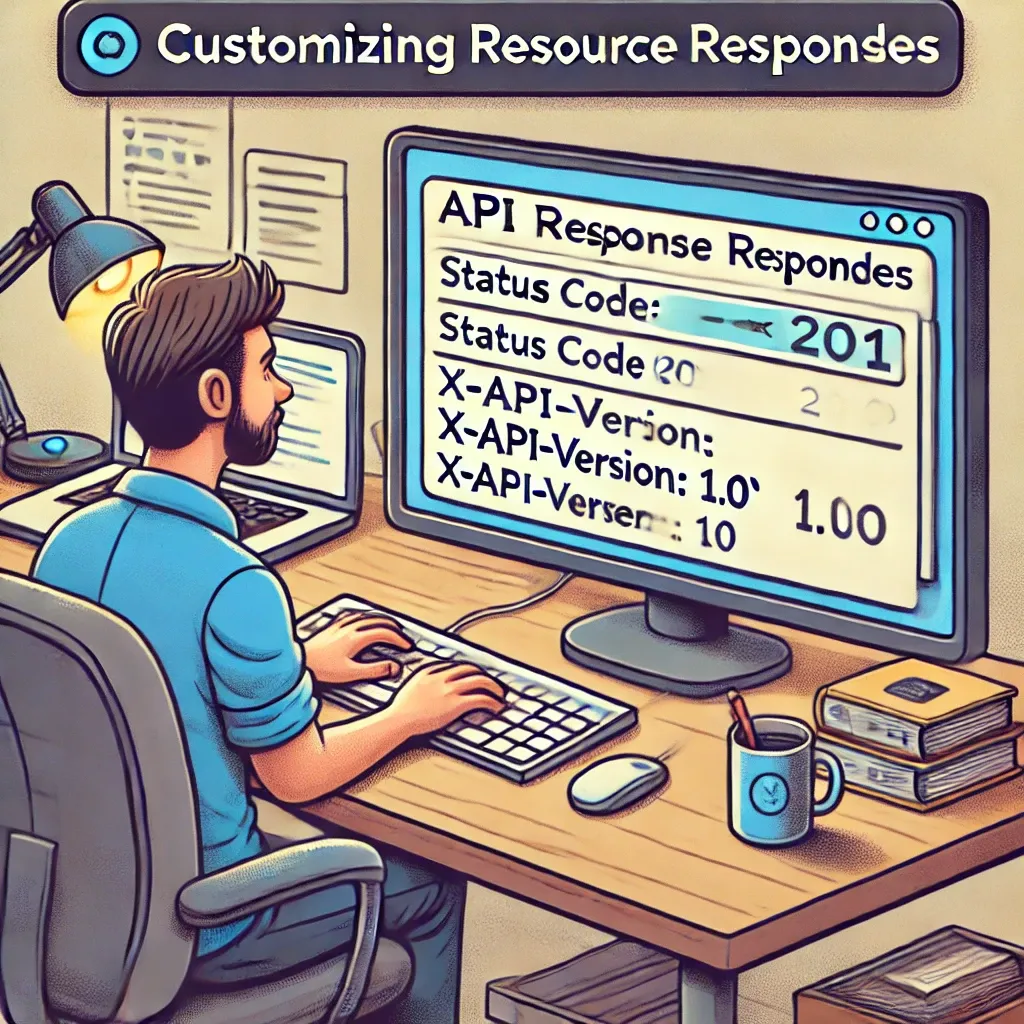
When building APIs with Laravel, fine-tuning your responses can significantly enhance the quality and flexibility of your application. Laravel's API Resources provide a powerful way to transform your Eloquent models and collections into JSON, but sometimes you need even more control. Enter the withResponse()
method – a gem that allows you to customize the outgoing HTTP response for your API resources.
The Power of withResponse()
The withResponse()
method in API Resources gives you the ability to modify the entire response object before it's sent back to the client. This opens up a world of possibilities for customizing your API's behavior.
Let's look at how to implement this:
class UserResource extends JsonResource
{
public function toArray($request)
{
return [
'id' => $this->id,
'name' => $this->name,
'email' => $this->email,
// ... other user attributes
];
}
public function withResponse($request, $response)
{
$response->header('X-API-Version', '1.0');
$response->setStatusCode(201);
}
}
In this example, we're doing two things in the withResponse()
method:
- Adding a custom header
X-API-Version
to indicate the API version. - Setting the HTTP status code to 201 (Created).
Practical Use Cases
1. API Versioning
Adding version information to your API responses can help clients handle potential changes in your API structure:
public function withResponse($request, $response)
{
$response->header('X-API-Version', '1.0');
}
2. Rate Limiting Information
You can include rate limit information in your response headers:
public function withResponse($request, $response)
{
$response->header('X-RateLimit-Limit', 60);
$response->header('X-RateLimit-Remaining', 59);
}
3. Caching Headers
Set caching headers to improve performance for your API consumers:
public function withResponse($request, $response)
{
$response->header('Cache-Control', 'public, max-age=3600');
}
4. Conditional Responses
You can even use withResponse()
to set different status codes based on certain conditions:
public function withResponse($request, $response)
{
$statusCode = $this->wasRecentlyCreated ? 201 : 200;
$response->setStatusCode($statusCode);
}
The withResponse()
method in Laravel's API Resources is a powerful tool for customizing your API responses. Whether you're adding version information, setting custom headers, or dynamically changing status codes, this feature allows you to create more informative and flexible APIs.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!