Managing Scheduled Task Output in Laravel
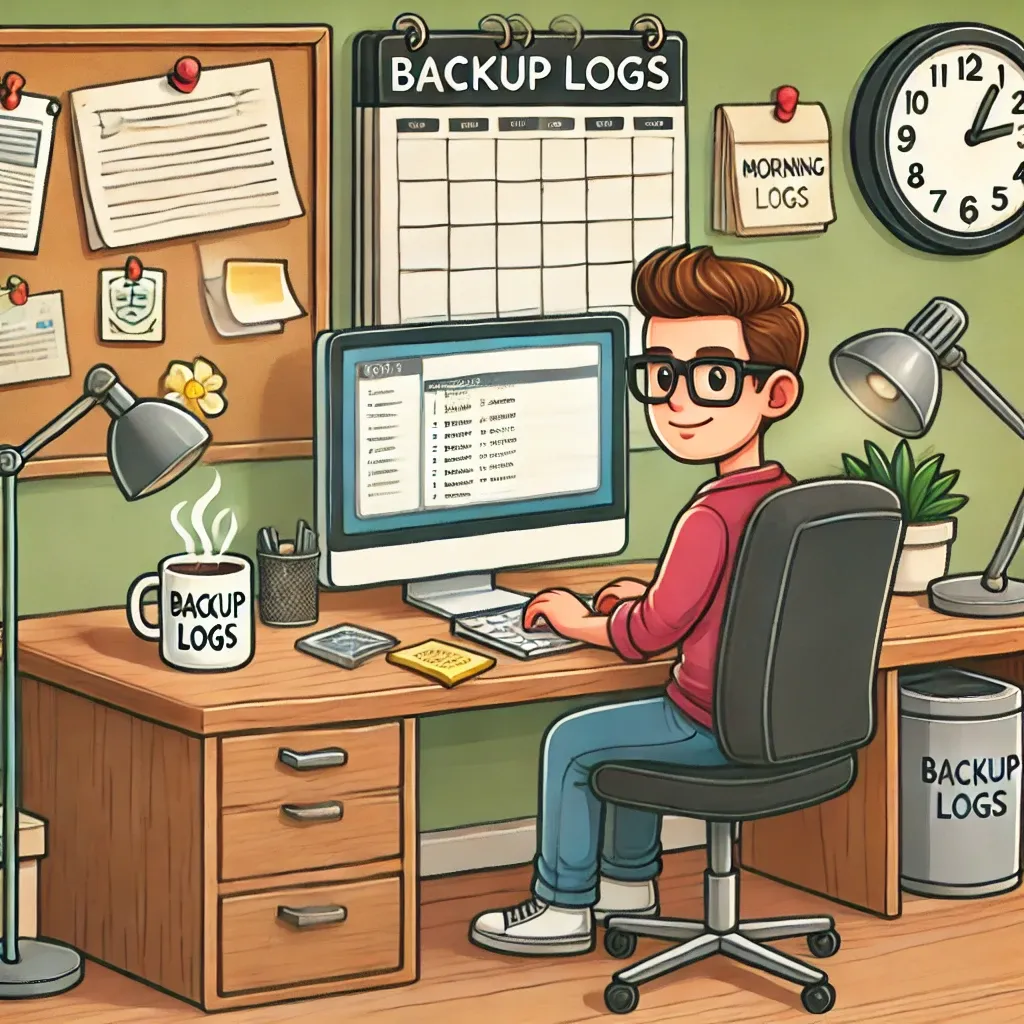
Need to track your scheduled task outputs? Laravel's scheduler provides elegant methods for capturing and storing command output for later review.
Basic Usage
Save command output to a file:
use Illuminate\Support\Facades\Schedule;
// Save output to file
Schedule::command('emails:send')
->daily()
->sendOutputTo($filePath);
// Append output to existing file
Schedule::command('emails:send')
->daily()
->appendOutputTo($filePath);
Real-World Example
Here's how you might implement comprehensive task output logging:
class TaskScheduler
{
protected $outputPath = 'storage/logs/scheduled-tasks';
public function registerTasks()
{
// Database backup with output logging
Schedule::command('backup:run')
->daily()
->at('01:00')
->appendOutputTo($this->getLogPath('backup'))
->before(function () {
Log::info('Starting database backup...');
})
->after(function () {
Log::info('Backup process completed');
});
// Report generation with separate logs
Schedule::command('reports:generate')
->weekly()
->mondays()
->at('07:00')
->sendOutputTo($this->getLogPath('reports'));
// Clean old logs weekly
Schedule::call(function () {
$this->cleanOldLogs();
})->weekly();
}
protected function getLogPath($task)
{
$date = now()->format('Y-m-d');
return storage_path(
"logs/scheduled-tasks/{$task}-{$date}.log"
);
}
protected function cleanOldLogs()
{
$files = File::files($this->outputPath);
foreach ($files as $file) {
$age = now()->diffInDays(File::lastModified($file));
if ($age > 30) {
File::delete($file);
}
}
}
}
These output handling methods make it easy to track and debug your scheduled tasks.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!