Managing Nested Attributes with Laravel's Fluent set Method
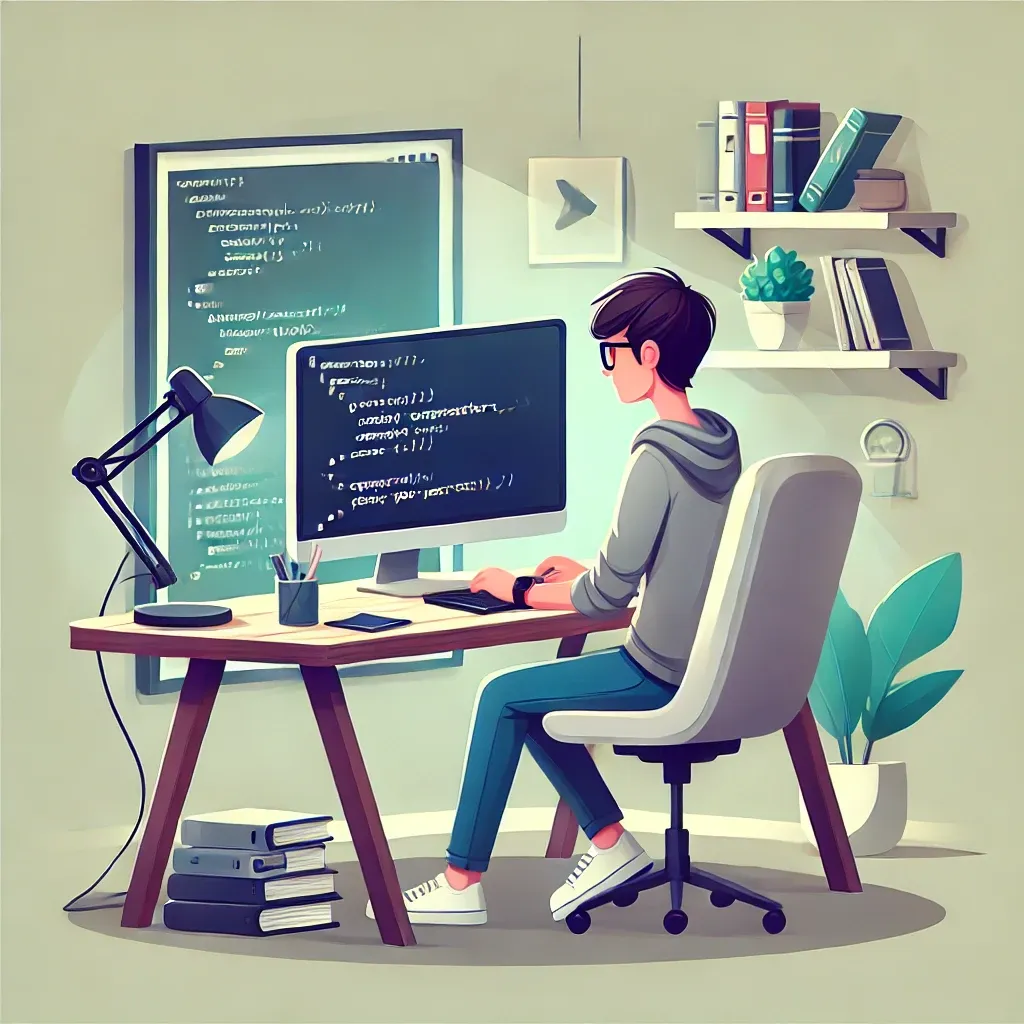
Need to manage nested attributes more effectively? Laravel's Fluent class now includes a set() method that makes handling complex data structures more intuitive.
Basic Usage
Set attributes using dot notation:
$fluent = new Fluent;
// Basic attributes
$fluent->set('product', 'iPhone')
->set('version', 15);
// Nested attributes
$fluent->set('specs.color', 'Space Black')
->set('specs.price.usd', 1199);
// Access values
echo $fluent->product; // "iPhone"
echo $fluent->get('specs.color'); // "Space Black"
echo $fluent->specs['price']['usd']; // 1199
Real-World Example
Here's how you might use it in a product configuration system:
class ProductConfigurator
{
private $config;
public function __construct()
{
$this->config = new Fluent;
}
public function setBasicDetails(string $name, string $sku)
{
$this->config
->set('product.name', $name)
->set('product.sku', $sku)
->set('product.created_at', now());
return $this;
}
public function setPricing(float $base, array $taxes)
{
$this->config
->set('pricing.base', $base)
->set('pricing.taxes', $taxes)
->set('pricing.total', $base + array_sum($taxes));
return $this;
}
public function setShipping(array $dimensions, float $weight)
{
$this->config
->set('shipping.dimensions', $dimensions)
->set('shipping.weight', $weight)
->set('shipping.requires_special', $weight > 20);
return $this;
}
public function getConfiguration()
{
return $this->config;
}
}
// Usage
$configurator = new ProductConfigurator();
$product = $configurator
->setBasicDetails('Gaming Laptop', 'LAP-2024-001')
->setPricing(999.99, ['vat' => 199.99])
->setShipping(['length' => 15, 'width' => 10], 2.5)
->getConfiguration();
The set() method makes it easy to manage complex nested configurations with a clean, fluent interface.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!