Making API Resources More Elegant with Laravel's Fluent Methods
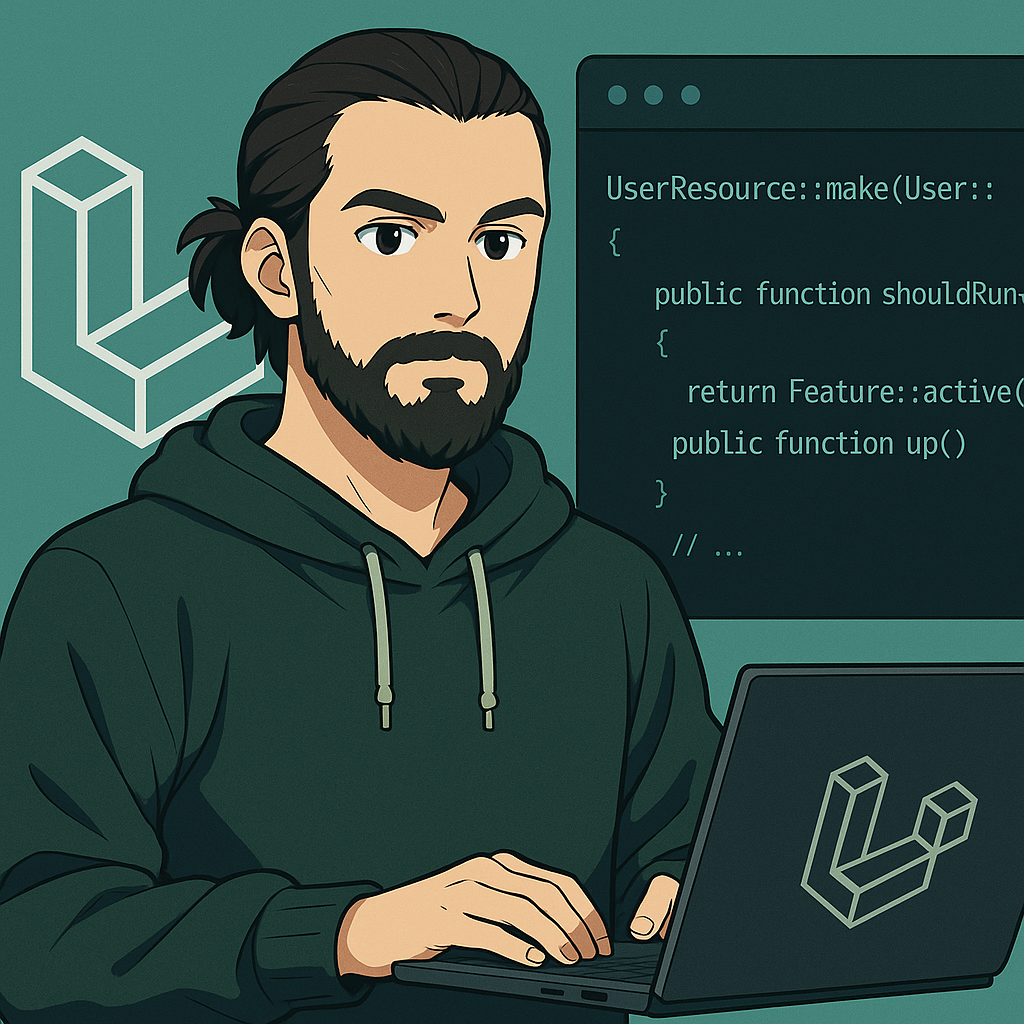
Tired of jumping through hoops to transform your Eloquent models into API resources? Laravel's fluent resource methods offer a more elegant approach that will make your code cleaner and more intuitive.
Understanding the Problem
When building APIs with Laravel, transforming your Eloquent models into resources is a common task. Traditionally, this has been done by wrapping your models with resource classes:
UserResource::make(User::find(1));
UserResource::collection(User::query()->active()->paginate());
While this approach works well, it creates a separation between your models and the transformation process. You first retrieve your model, then wrap it in a separate step. This two-step process can make your code feel less fluent and natural.
The Fluent Resource Solution
Laravel now offers fluent methods directly on Eloquent models and collections that streamline this process:
User::find(1)->toResource(UserResource::class);
User::query()->active()->paginate()->toResourceCollection(UserResource::class);
This approach creates a more natural flow from model retrieval to transformation, and your code reads from left to right without mental context switching.
Convention-Based Resources
Taking things even further, Laravel can automatically guess the resource class based on model name conventions:
User::find(1)->toResource();
When calling toResource()
without arguments, Laravel will look for a resource class named after your model. If your model is User
, it will look for UserResource
. This convention-over-configuration approach reduces boilerplate code while maintaining clarity.
Real-World Example
Let's look at a practical example of how these fluent methods improve your controller code:
class UserController extends Controller
{
public function show($id)
{
// Before:
// return UserResource::make(User::findOrFail($id));
// After:
return User::findOrFail($id)->toResource();
}
public function index(Request $request)
{
$users = User::query()
->when($request->has('active'), fn($query) => $query->active())
->latest()
->paginate();
// Before:
// return UserResource::collection($users);
// After:
return $users->toResourceCollection();
}
}
The fluent approach creates a more natural code flow that follows how we think about the problem: "Get me this user and transform it into a resource."
Stay up to date with more Laravel tips and tricks by following me: