Make file uploads a breeze with Laravel Livewire
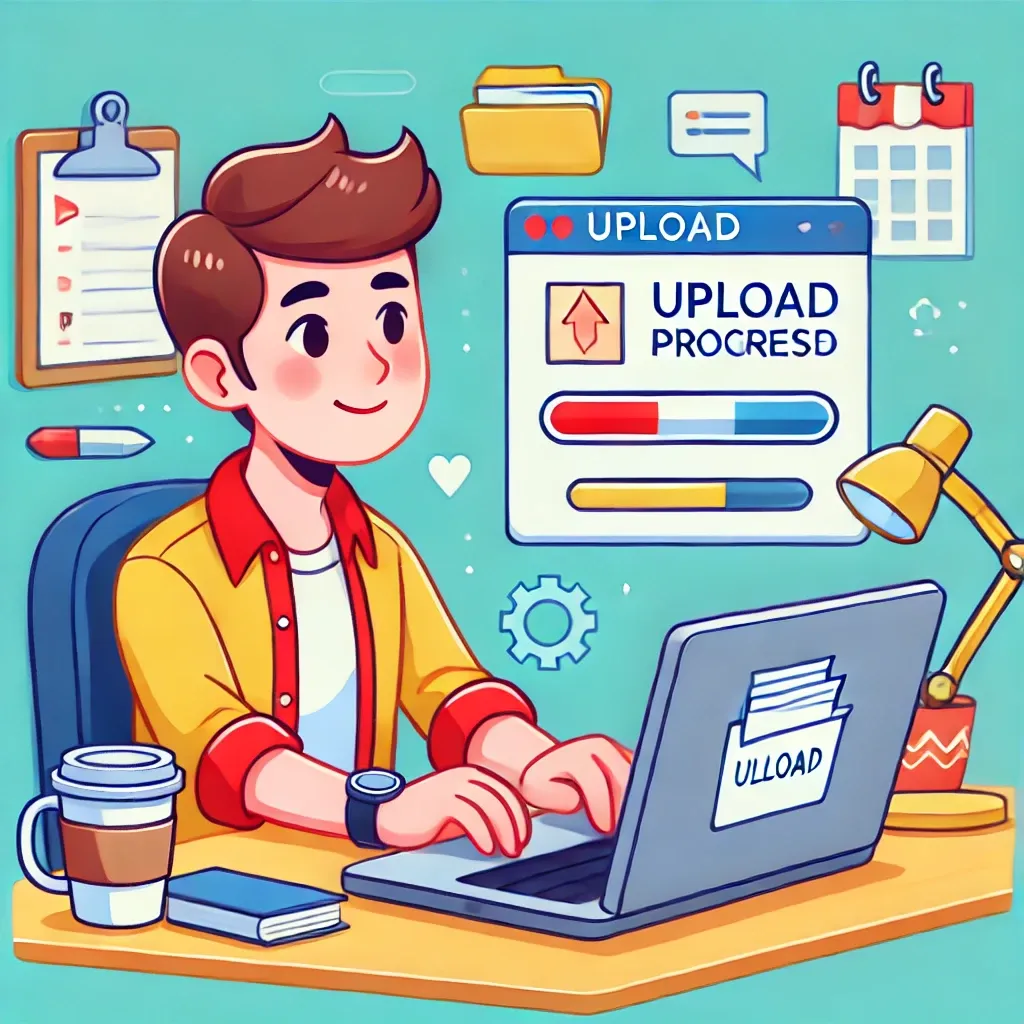
Hey Laravel devs, here is a gem for you! 💎
File uploads are a common requirement in many web applications, but they can be challenging to implement efficiently. With Laravel Livewire, you can handle file uploads seamlessly within your components, making the process straightforward and efficient. In this blog post, we’ll explore how to use Laravel Livewire's file upload capabilities to enhance your application.
Why Use Laravel Livewire for File Uploads?
Laravel Livewire simplifies the process of handling file uploads by providing a smooth, integrated experience without the need for extensive JavaScript. This ensures a cohesive development workflow and a better user experience.
Benefits of Laravel Livewire File Uploads
Using Laravel Livewire for file uploads offers several advantages:
- Seamless Integration: Manage file uploads directly within your Laravel Livewire components.
- Real-Time Feedback: Provide users with immediate feedback on the status of their uploads.
- Ease of Use: Simplify the file upload process without writing complex JavaScript code.
How to Implement File Uploads with Laravel Livewire
Laravel Livewire makes it easy to handle file uploads. Here’s a step-by-step guide to implementing file uploads in your application:
Step 1: Create the Blade Component
Create a Blade component to handle the file input and upload button:
<!-- resources/views/livewire/upload-file.blade.php -->
<div>
<input type="file" wire:model="file">
<button wire:click="save">Upload</button>
</div>
This Blade component contains an input field for selecting a file and a button to trigger the upload action.
Step 2: Create the Laravel Livewire Component
Next, create a Laravel Livewire component to handle the file upload logic:
<?php
namespace App\Http\Livewire;
use Livewire\Component;
use Livewire\WithFileUploads;
class UploadFile extends Component
{
use WithFileUploads;
public $file;
public function save()
{
$this->validate([
'file' => 'required|file|max:1024', // 1MB Max
]);
$this->file->store('uploads');
session()->flash('message', 'File successfully uploaded.');
}
public function render()
{
return view('livewire.upload-file');
}
}
In this component, we use the WithFileUploads
trait to manage the file upload process. The save
method validates the file and stores it in the uploads
directory. A success message is then flashed to the session.
Real-Life Example
Consider a scenario where users need to upload profile pictures. Handling this process efficiently is crucial for providing a good user experience. With Laravel Livewire, you can manage file uploads seamlessly within your application.
Here's how you might implement a profile picture upload feature:
- User Interface: Provide an input field for users to select their profile picture and a button to upload the file.
- File Validation: Ensure that the uploaded file meets the required criteria (e.g., file type, size).
- File Storage: Save the uploaded file in a designated directory and update the user's profile with the new picture.
Example:
// Blade Component: resources/views/livewire/upload-profile-picture.blade.php
<div>
<input type="file" wire:model="profilePicture">
<button wire:click="upload">Upload Profile Picture</button>
</div>
// Livewire Component: app/Http/Livewire/UploadProfilePicture.php
<?php
namespace App\Http\Livewire;
use Livewire\Component;
use Livewire\WithFileUploads;
class UploadProfilePicture extends Component
{
use WithFileUploads;
public $profilePicture;
public function upload()
{
$this->validate([
'profilePicture' => 'required|image|max:1024', // 1MB Max
]);
$path = $this->profilePicture->store('profile-pictures');
auth()->user()->update(['profile_picture' => $path]);
session()->flash('message', 'Profile picture updated successfully.');
}
public function render()
{
return view('livewire.upload-profile-picture');
}
}
Pro Tip
When handling file uploads, always validate the uploaded files to ensure they meet your application's requirements. This helps prevent issues such as oversized files or unsupported file types.
Using Laravel Livewire's file upload capabilities, you can simplify the process of handling file uploads in your Laravel application. This ensures a smooth user experience and a cohesive development workflow. Try integrating file uploads with Laravel Livewire in your next project and see the benefits for yourself!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!