Maintaining Data Structure in Laravel API Resources: Preserving Collection Keys
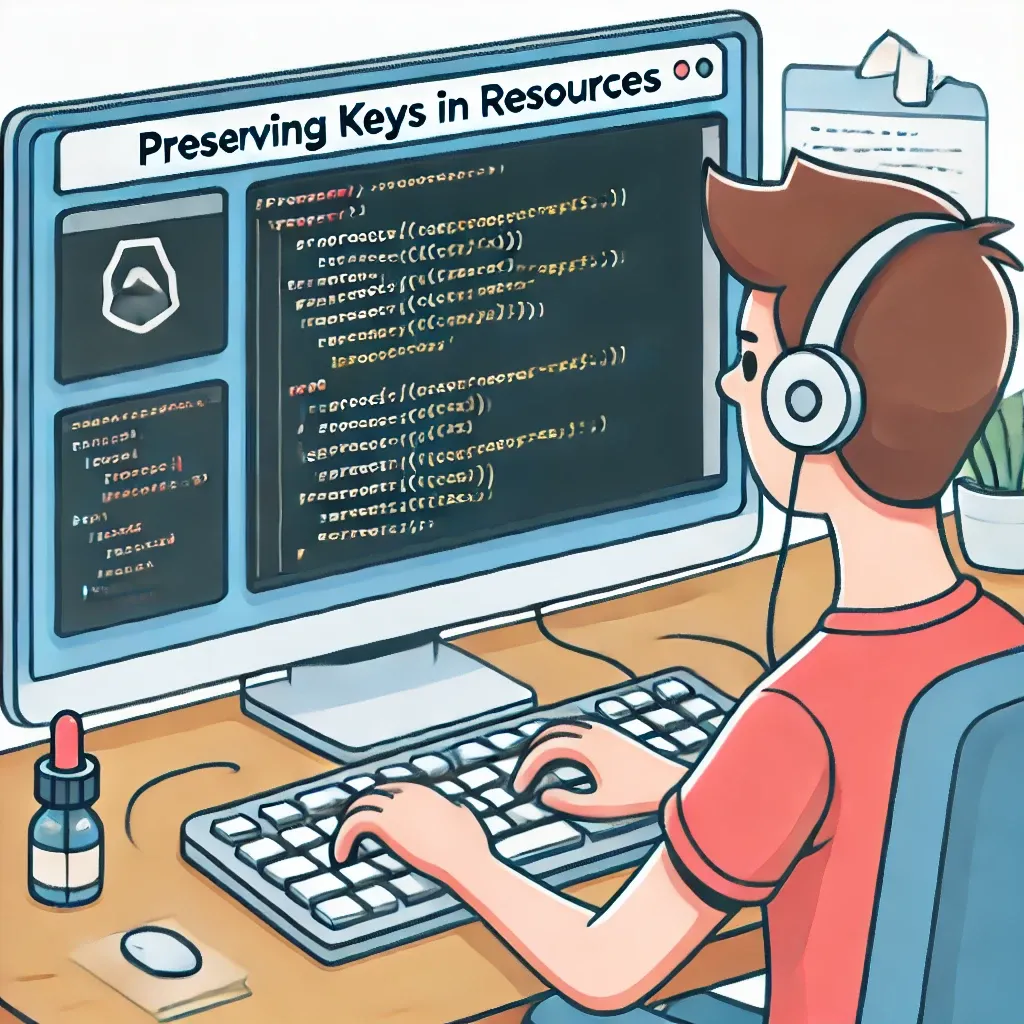
When building APIs with Laravel, you often use API resources to transform your models into JSON responses. By default, Laravel resets the keys of resource collections to numerical indexes. However, there are scenarios where preserving the original keys is crucial. Let's explore how to maintain your data structure using the preserveKeys
property in Laravel API resources.
The Default Behavior
Normally, when you return a resource collection from a route, Laravel reindexes the collection numerically. For example:
Route::get('/users', function () {
return UserResource::collection(User::all()->keyBy('id'));
});
This might result in a response like:
{
"data": [
{ "id": 1, "name": "Alice" },
{ "id": 2, "name": "Bob" }
]
}
Preserving Original Keys
To maintain the original keys, you can use the preserveKeys
property in your resource class:
<?php
namespace App\Http\Resources;
use Illuminate\Http\Resources\Json\JsonResource;
class UserResource extends JsonResource
{
public $preserveKeys = true;
public function toArray($request)
{
return [
'id' => $this->id,
'name' => $this->name,
// ... other attributes
];
}
}
Now, when you return a collection of UserResource
, the original keys will be preserved:
Route::get('/users', function () {
return UserResource::collection(User::all()->keyBy('id'));
});
This will result in a response like:
{
"data": {
"1": { "id": 1, "name": "Alice" },
"2": { "id": 2, "name": "Bob" }
}
}
Real-World Application
Preserving keys can be particularly useful in scenarios where the keys themselves carry meaning. For instance, consider a system where you're returning a collection of configuration settings:
class ConfigResource extends JsonResource
{
public $preserveKeys = true;
public function toArray($request)
{
return [
'value' => $this->value,
'last_updated' => $this->updated_at->toDateTimeString(),
];
}
}
Route::get('/config', function () {
$config = Config::all()->keyBy('key');
return ConfigResource::collection($config);
});
This might return a response like:
{
"data": {
"site_name": { "value": "My Awesome Site", "last_updated": "2023-05-15 10:30:00" },
"maintenance_mode": { "value": false, "last_updated": "2023-05-14 09:15:00" }
}
}
Here, the configuration keys are preserved, making it easy for the client to access specific settings directly.
Conclusion
The preserveKeys
property in Laravel API resources provides a simple yet powerful way to maintain the structure of your data when building APIs. By preserving collection keys, you can create more meaningful and easily consumable API responses, especially when dealing with key-value pairs or when the keys themselves carry important information.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!