Localizing Laravel Resource Routes: A Guide to Custom Verbs
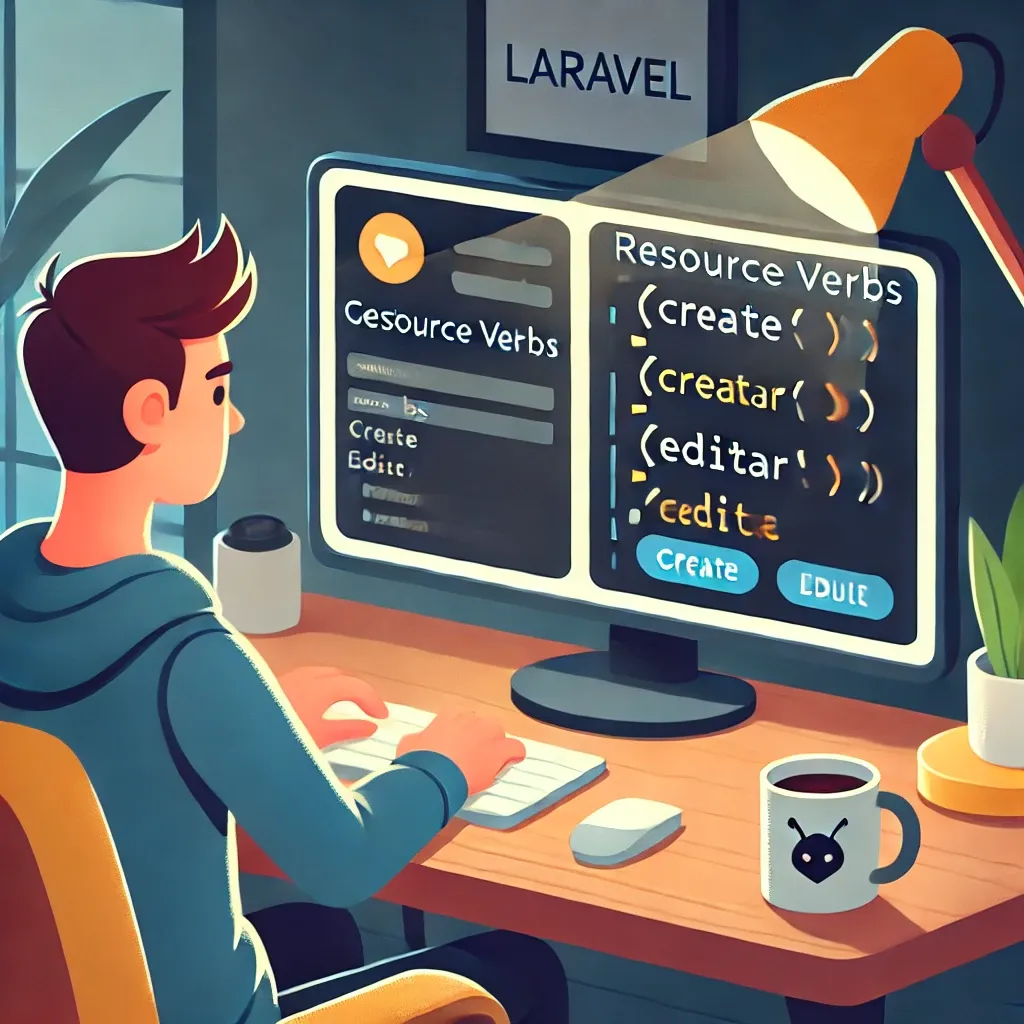
When building applications for a global audience, every detail matters - including your URL structure. Laravel's resource routing is a powerful feature, but it defaults to English verbs. What if you're building an application in Spanish, French, or any other language? Today, we'll explore how to localize resource route verbs in Laravel, making your application truly speak your users' language.
Understanding Resource Route Localization
By default, Laravel's Route::resource
method creates URIs using English verbs. For example, a 'create' route might look like /users/create
. But what if you want it to be /users/crear
in Spanish? Laravel provides a simple solution with the Route::resourceVerbs
method.
Implementing Custom Resource Verbs
To localize your resource route verbs, you'll need to use the Route::resourceVerbs
method in your App\Providers\AppServiceProvider
. Here's how to do it:
<?php
namespace App\Providers;
use Illuminate\Support\Facades\Route;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* Bootstrap any application services.
*/
public function boot(): void
{
Route::resourceVerbs([
'create' => 'crear',
'edit' => 'editar',
]);
}
}
In this example, we're changing the 'create' verb to 'crear' and the 'edit' verb to 'editar', which are their Spanish equivalents.
Real-Life Example
Let's consider a scenario where we're building a blog application for a Spanish-speaking audience. We have a PostController
that manages blog posts. Here's how we might set it up:
- First, we localize the resource verbs in
AppServiceProvider
:
public function boot(): void
{
Route::resourceVerbs([
'create' => 'crear',
'edit' => 'editar',
]);
}
- Then, we define our resource route in
routes/web.php
:
Route::resource('posts', PostController::class);
- Now, let's look at how this affects our URLs:
// Before localization:
// GET /posts/create
// GET /posts/{post}/edit
// After localization:
// GET /posts/crear
// GET /posts/{post}/editar
Here's how you might use these routes in your Blade templates:
<a href="{{ route('posts.crear') }}">Crear Nuevo Post</a>
@foreach ($posts as $post)
<a href="{{ route('posts.editar', $post) }}">Editar</a>
@endforeach
And in your controller:
class PostController extends Controller
{
public function crear()
{
return view('posts.crear');
}
public function editar(Post $post)
{
return view('posts.editar', compact('post'));
}
}
The output of these routes would look like this:
// GET /posts/crear
{
"view": "posts.crear",
"data": {}
}
// GET /posts/1/editar
{
"view": "posts.editar",
"data": {
"post": {
"id": 1,
"title": "Mi Primer Post",
"content": "Contenido del post..."
}
}
}
By localizing your resource route verbs, you create a more cohesive and intuitive experience for your users, especially in non-English applications. This small change can significantly improve the user experience and make your application feel more native to your target audience.
Remember, while we've focused on Spanish in this example, you can use this technique to localize your routes to any language, making Laravel a truly global framework.
If you found this guide helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel tips and tricks!