Leveraging Lifecycle Hooks in Laravel Livewire: Mastering Component Flow
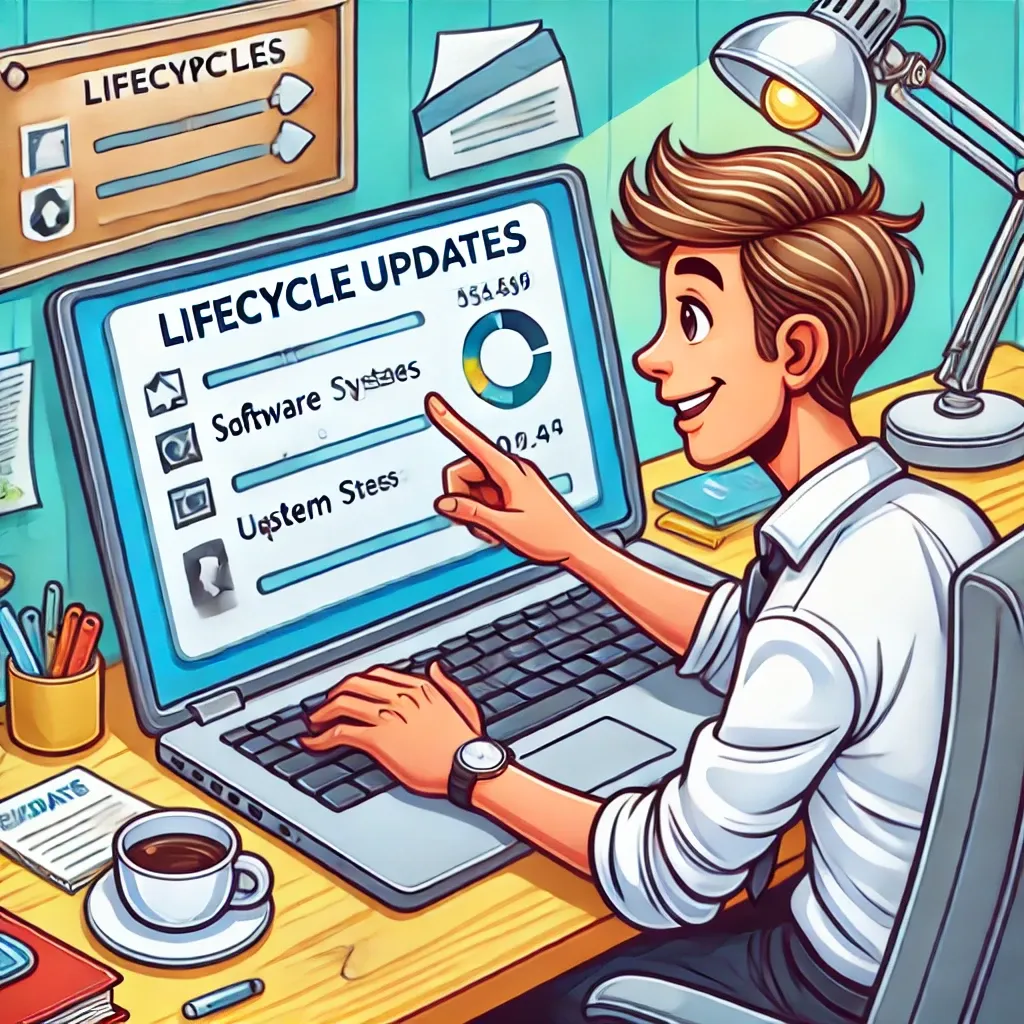
Livewire's lifecycle hooks provide developers with fine-grained control over component behavior at various stages of its lifecycle. Let's explore these hooks and how they can be used effectively.
Mount Hook
The mount()
method is called when a component is first initialized. It's ideal for setting up initial component state:
class SearchComponent extends Component
{
public $search = '';
public function mount($initialSearch = '')
{
$this->search = $initialSearch;
}
}
Hydrate Hook
The hydrate()
method is called when the component is re-hydrated on subsequent requests:
public function hydrate()
{
$this->search = strtolower($this->search);
}
Updating/Updated Hooks
These hooks are called before and after a property is updated:
public function updating($name, $value)
{
if ($name === 'search') {
// Do something before the search property is updated
}
}
public function updated($name, $value)
{
if ($name === 'search') {
$this->results = Product::search($value)->get();
}
}
Dehydrate Hook
This hook is called before the component is dehydrated (serialized for the frontend):
public function dehydrate()
{
$this->results = $this->results->take(5);
}
Rendering Hook
The rendering
and rendered
hooks are called before and after a component is rendered:
public function rendering()
{
// Do something before the component renders
}
public function rendered()
{
// Do something after the component renders
}
Boot Hook
The boot()
method is a static method called when the component is first booted:
public static function boot()
{
// Component-wide setup
}
Real-World Example
Let's look at a more comprehensive example using multiple lifecycle hooks:
class UserDashboard extends Component
{
public $user;
public $stats;
public $recentActivity;
public function mount(User $user)
{
$this->user = $user;
}
public function hydrate()
{
// Refresh user data on each request
$this->user->refresh();
}
public function updating($name, $value)
{
if ($name === 'user.settings') {
// Validate settings before update
$this->validate([
'user.settings' => 'json',
]);
}
}
public function updated($name, $value)
{
if ($name === 'user.settings') {
// Clear cache after settings update
Cache::forget("user-{$this->user->id}-settings");
}
}
public function rendering()
{
// Load stats before rendering
$this->stats = $this->user->getStats();
}
public function rendered()
{
// Log component render
Log::info("UserDashboard rendered for user {$this->user->id}");
}
public function dehydrate()
{
// Limit recent activity for payload optimization
$this->recentActivity = $this->recentActivity->take(10);
}
public static function boot()
{
// Register a global middleware for this component
static::$middleware[] = EnsureUserIsActive::class;
}
public function render()
{
$this->recentActivity = $this->user->recentActivity;
return view('livewire.user-dashboard');
}
}
This example demonstrates how various lifecycle hooks can be used to create a robust, efficient Livewire component. By understanding and utilizing these hooks, you can create more responsive, optimized, and maintainable Livewire applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!