Leveling Up Laravel Middleware: Mastering Parameterized Middleware
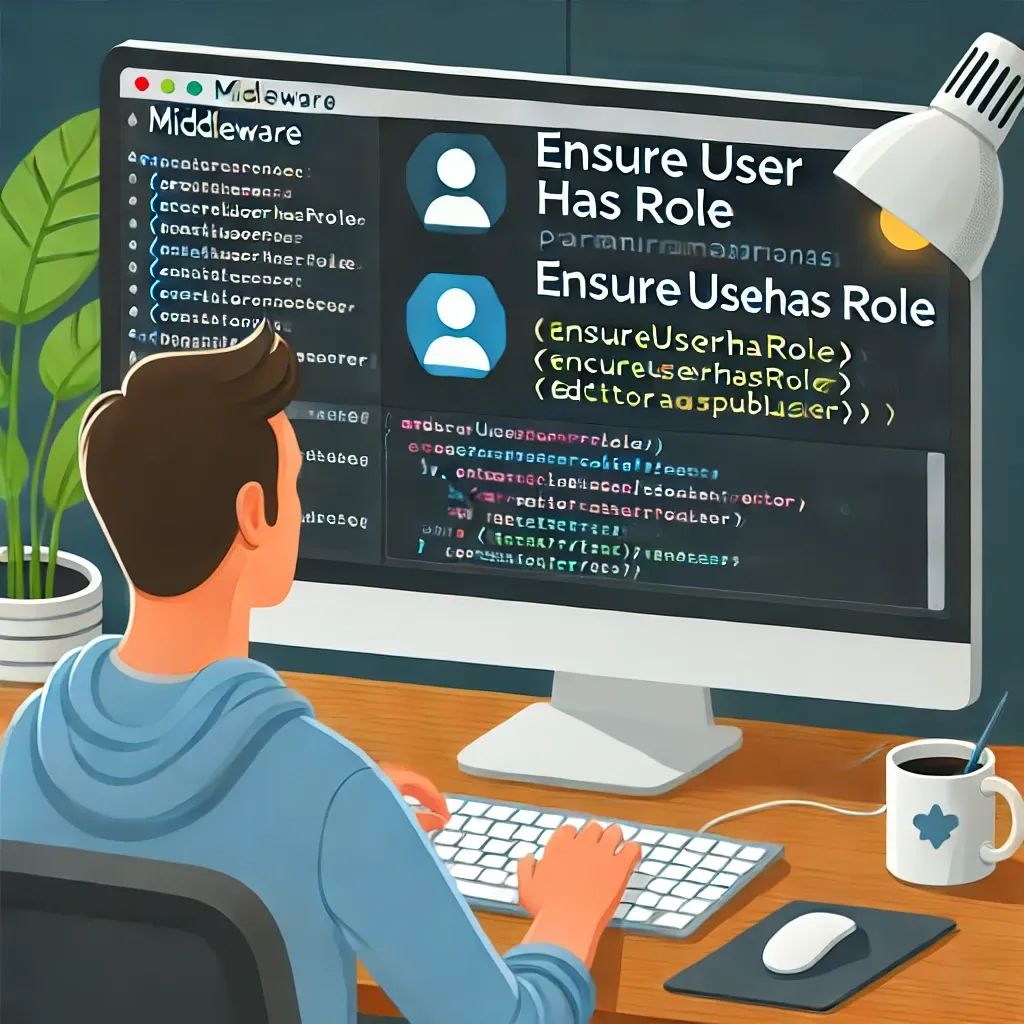
In the world of web application development, flexibility is key. Laravel's middleware system is already powerful, but did you know you can make it even more dynamic by passing parameters? This feature allows you to create reusable middleware that can adapt its behavior based on the parameters you provide. Let's dive into how you can leverage this powerful feature in your Laravel applications.
Understanding Parameterized Middleware
Parameterized middleware in Laravel allows you to pass additional data to your middleware at the point of use. This is particularly useful when you want to reuse a piece of middleware logic but slightly alter its behavior in different contexts.
Creating Parameterized Middleware
Let's look at an example of a middleware that ensures a user has a specific role:
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
use Symfony\Component\HttpFoundation\Response;
class EnsureUserHasRole
{
public function handle(Request $request, Closure $next, string $role): Response
{
if (! $request->user()->hasRole($role)) {
return redirect('home')->with('error', 'You do not have the right permissions.');
}
return $next($request);
}
}
In this middleware, $role
is a parameter that we'll pass when applying the middleware to a route.
Using Parameterized Middleware
You can use this middleware in your routes file like this:
use App\Http\Middleware\EnsureUserHasRole;
Route::put('/post/{id}', function (string $id) {
// Update post logic here
})->middleware(EnsureUserHasRole::class.':editor');
Here, we're specifying that the user must have the 'editor' role to access this route.
You can even pass multiple parameters:
Route::put('/post/{id}', function (string $id) {
// Update post logic here
})->middleware(EnsureUserHasRole::class.':editor,publisher');
This would allow users with either the 'editor' or 'publisher' role to access the route.
Real-Life Example
Let's expand on our EnsureUserHasRole
middleware to create a more comprehensive role-based access control system:
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
use Symfony\Component\HttpFoundation\Response;
class EnsureUserHasRole
{
public function handle(Request $request, Closure $next, string ...$roles): Response
{
if (! $request->user() || ! $request->user()->hasAnyRole($roles)) {
return response()->json(['error' => 'Unauthorized'], 403);
}
return $next($request);
}
}
Now, let's use this middleware in our routes:
use App\Http\Controllers\PostController;
use App\Http\Middleware\EnsureUserHasRole;
Route::prefix('posts')->group(function () {
Route::get('/', [PostController::class, 'index']);
Route::post('/', [PostController::class, 'store'])
->middleware(EnsureUserHasRole::class.':editor,admin');
Route::put('/{id}', [PostController::class, 'update'])
->middleware(EnsureUserHasRole::class.':editor,admin');
Route::delete('/{id}', [PostController::class, 'destroy'])
->middleware(EnsureUserHasRole::class.':admin');
});
In this setup:
- Anyone can view posts
- Editors and admins can create and update posts
- Only admins can delete posts
Here's how the responses might look:
// GET /posts
// Accessible to all
[
{ "id": 1, "title": "First Post", "content": "..." },
{ "id": 2, "title": "Second Post", "content": "..." }
]
// POST /posts (as editor)
// Success:
{
"id": 3,
"title": "New Post",
"content": "...",
"created_at": "2023-06-15T12:00:00.000000Z"
}
// DELETE /posts/1 (as editor)
// Failure:
{
"error": "Unauthorized"
}
// DELETE /posts/1 (as admin)
// Success:
{
"message": "Post deleted successfully"
}
By mastering parameterized middleware in Laravel, you can create more flexible, reusable, and maintainable applications. This powerful feature allows you to implement complex authorization and filtering logic while keeping your code DRY (Don't Repeat Yourself) and your routes clean and readable.
If you found this guide helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel tips and tricks!