Laravel's Route::can() Gets Enum-Friendly: Simplifying Permission Checks
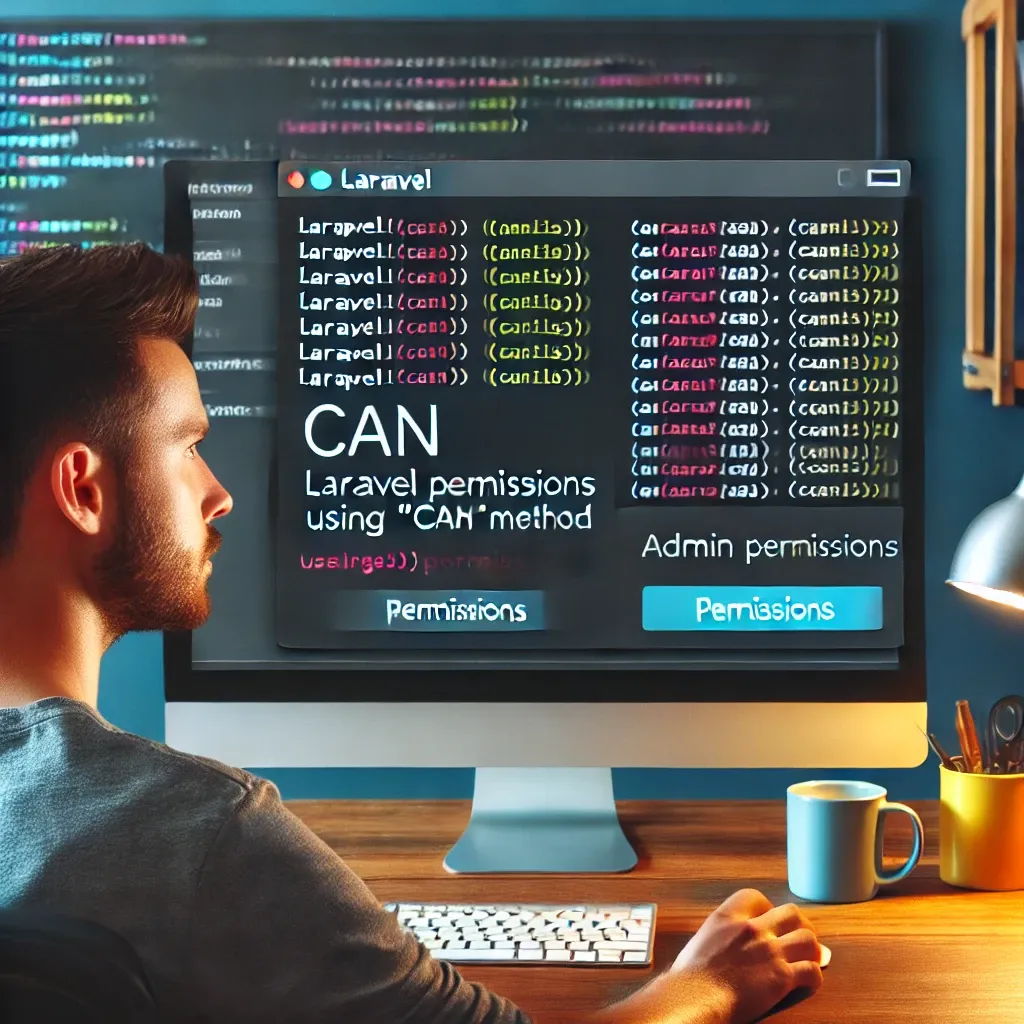
Ever found yourself typing out ->value
when using enums with Laravel's Route::can()
method? Well, good news! Laravel has made working with backed enums in route permissions even smoother. Let's dive into this small but mighty update.
The Old Way vs. The New Way
Here's a quick before and after:
// Before
Route::post('/request', function () {...})->can(Permissions::CAN_REQUEST->value);
// After
Route::post('/request', function () {...})->can(Permissions::CAN_REQUEST);
See that? No more ->value
needed!
Real-World Example: Admin Panel Routes
Let's say you're building an admin panel with different permission levels. Here's how you might use this:
use App\Enums\AdminPermissions;
class AdminPermissions extends BackedEnum
{
case VIEW_DASHBOARD = 'view_dashboard';
case MANAGE_USERS = 'manage_users';
case EDIT_SETTINGS = 'edit_settings';
}
Route::prefix('admin')->group(function () {
Route::get('/dashboard', [DashboardController::class, 'index'])
->can(AdminPermissions::VIEW_DASHBOARD);
Route::resource('users', UserController::class)
->can(AdminPermissions::MANAGE_USERS);
Route::put('/settings', [SettingsController::class, 'update'])
->can(AdminPermissions::EDIT_SETTINGS);
});
In this setup:
- We define an enum for admin permissions
- We group our admin routes
- We apply permissions using
can()
with our enum values directly
This approach makes your routes more readable and your permissions more manageable. Plus, it leverages the power of PHP's backed enums for type safety and autocompletion.
By embracing this update, you're not just saving keystrokes – you're making your Laravel routes more expressive and your code more robust. It's a small change that can make a big difference in your day-to-day coding!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!