Laravel's nullOnUpdate(): A Neat Trick for Foreign Key Relationships
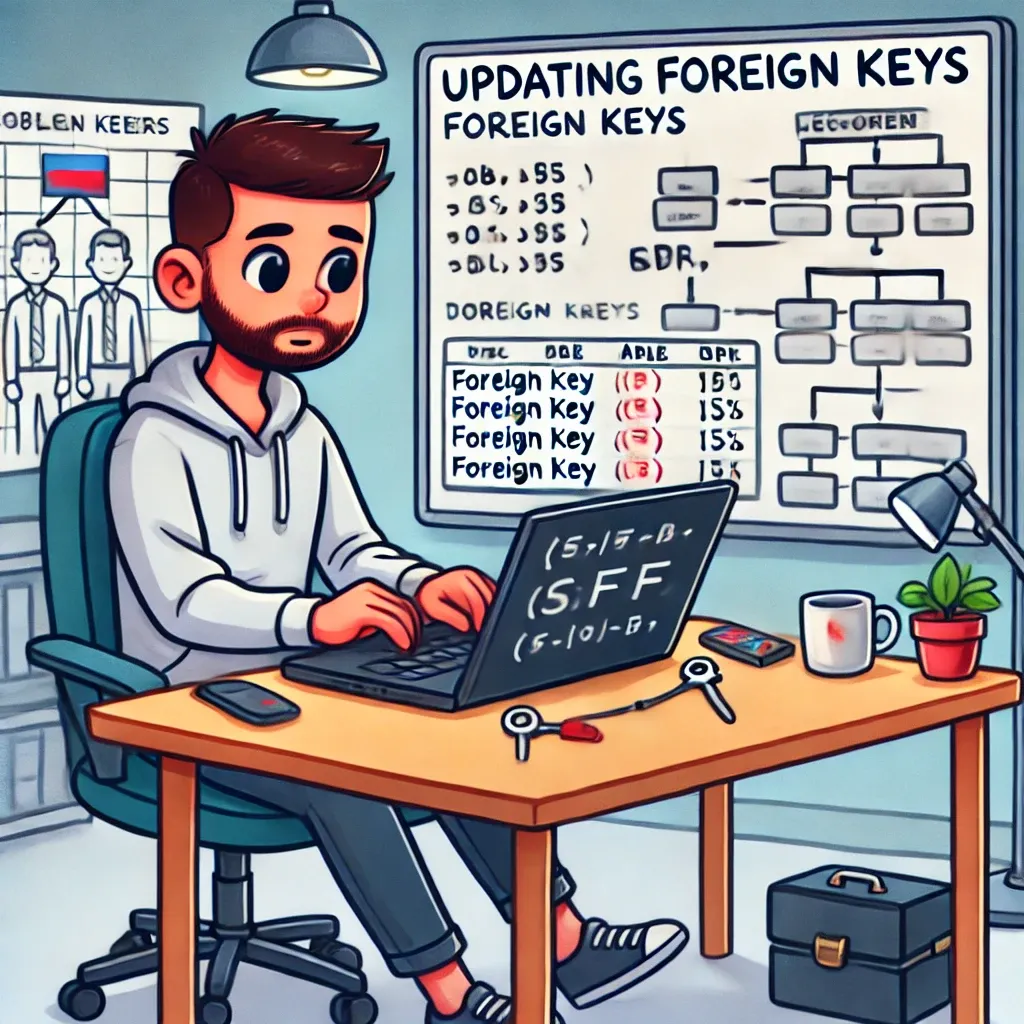
Ever wondered what happens to your foreign keys when the referenced record gets updated? Laravel's nullOnUpdate()
method gives you a neat way to handle this. Let's dive in!
What is nullOnUpdate()?
The nullOnUpdate()
method is a handy addition to Laravel's ForeignKeyDefinition
schema class. It tells your database to set the foreign key to null when the referenced record is updated.
Basic Usage
Here's how you use it:
$table->foreign('user_id')
->references('id')
->on('users')
->nullOnUpdate();
What's happening here?
- We're setting up a foreign key on the
user_id
column - It references the
id
column in theusers
table - The
nullOnUpdate()
part says "If the referenced user's ID changes, set this foreign key to null"
Real-World Example: Comment System
Let's say you're building a comment system for a blog. Here's how you might use nullOnUpdate()
:
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateCommentsTable extends Migration
{
public function up()
{
Schema::create('comments', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('user_id')->nullable();
$table->text('content');
$table->timestamps();
$table->foreign('user_id')
->references('id')
->on('users')
->nullOnUpdate()
->nullOnDelete();
}
}
public function down()
{
Schema::dropIfExists('comments');
}
}
In this example:
- We create a
comments
table with a nullableuser_id
- We set up a foreign key relationship to the
users
table - We use
nullOnUpdate()
to handle user ID changes - We also use
nullOnDelete()
to handle user deletions
Using nullOnUpdate()
gives you more flexibility in managing your database relationships. It's a small feature, but it can make a big difference in how your app handles data changes!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!