Laravel's Context Helper: Managing Contextual Data
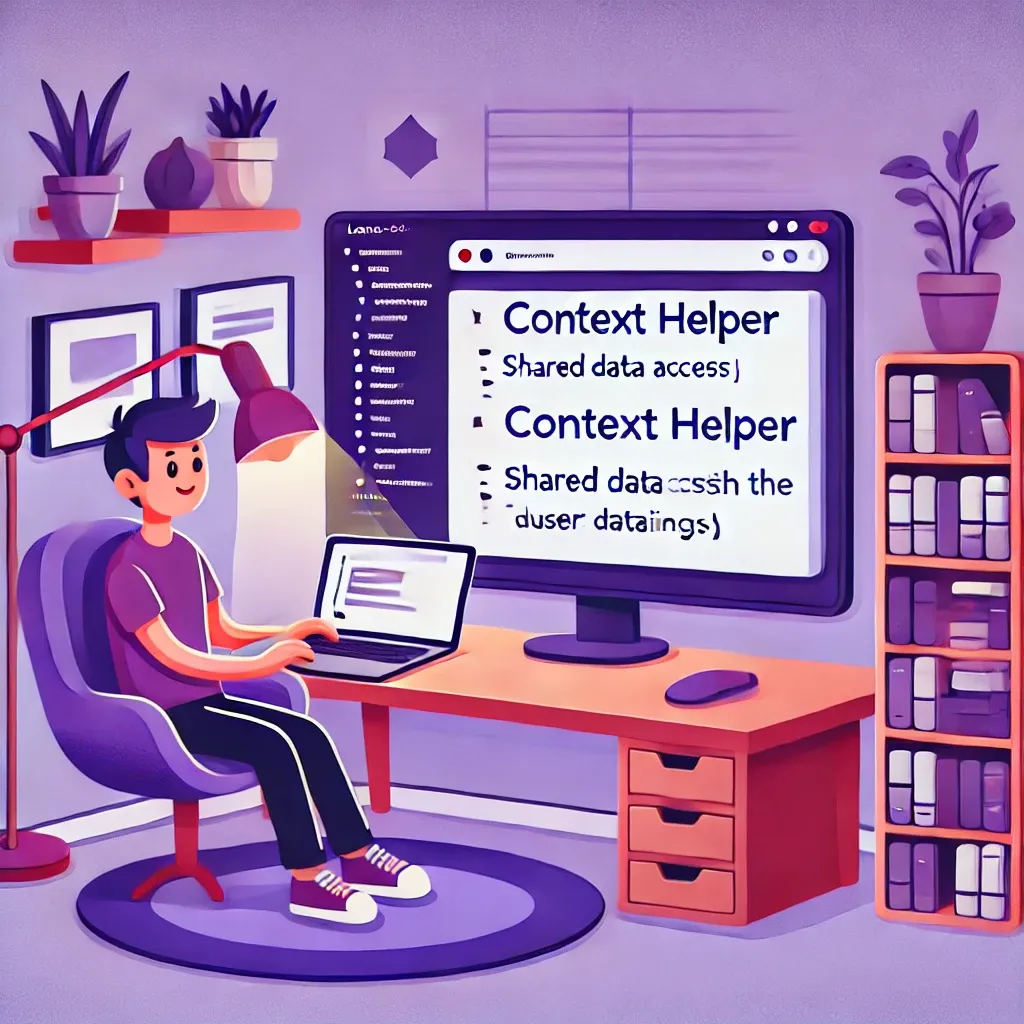
Need a simpler way to handle context in your Laravel application? The new context() helper function brings an elegant solution for managing contextual data throughout your app's lifecycle.
Basic Usage
The context helper provides multiple ways to interact with contextual data:
// Add data to context
context(['user' => auth()->user()]);
// Get entire context object
$context = context();
// Get specific value
$user = context('user');
// Get with default fallback
$theme = context('theme', 'light');
Real-World Example
Here's how you might use context in a multi-tenant application:
class TenantMiddleware
{
public function handle($request, $next)
{
$tenant = Tenant::fromDomain($request->getHost());
context([
'tenant' => $tenant,
'tenant_settings' => $tenant->settings,
'tenant_features' => $tenant->activeFeatures
]);
return $next($request);
}
}
class OrderController extends Controller
{
public function store(OrderRequest $request)
{
$tenant = context('tenant');
$features = context('tenant_features', []);
if (in_array('advanced_ordering', $features)) {
return $this->handleAdvancedOrder($request);
}
// Regular order processing
$order = Order::create([
'tenant_id' => $tenant->id,
'user_id' => auth()->id(),
'items' => $request->items
]);
return response()->json([
'order' => $order,
'settings' => context('tenant_settings')
]);
}
}
The context helper makes it easy to maintain and access shared data throughout your request lifecycle.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!