Interactive Command Prompts in Laravel: Guide to PromptsForMissingInput
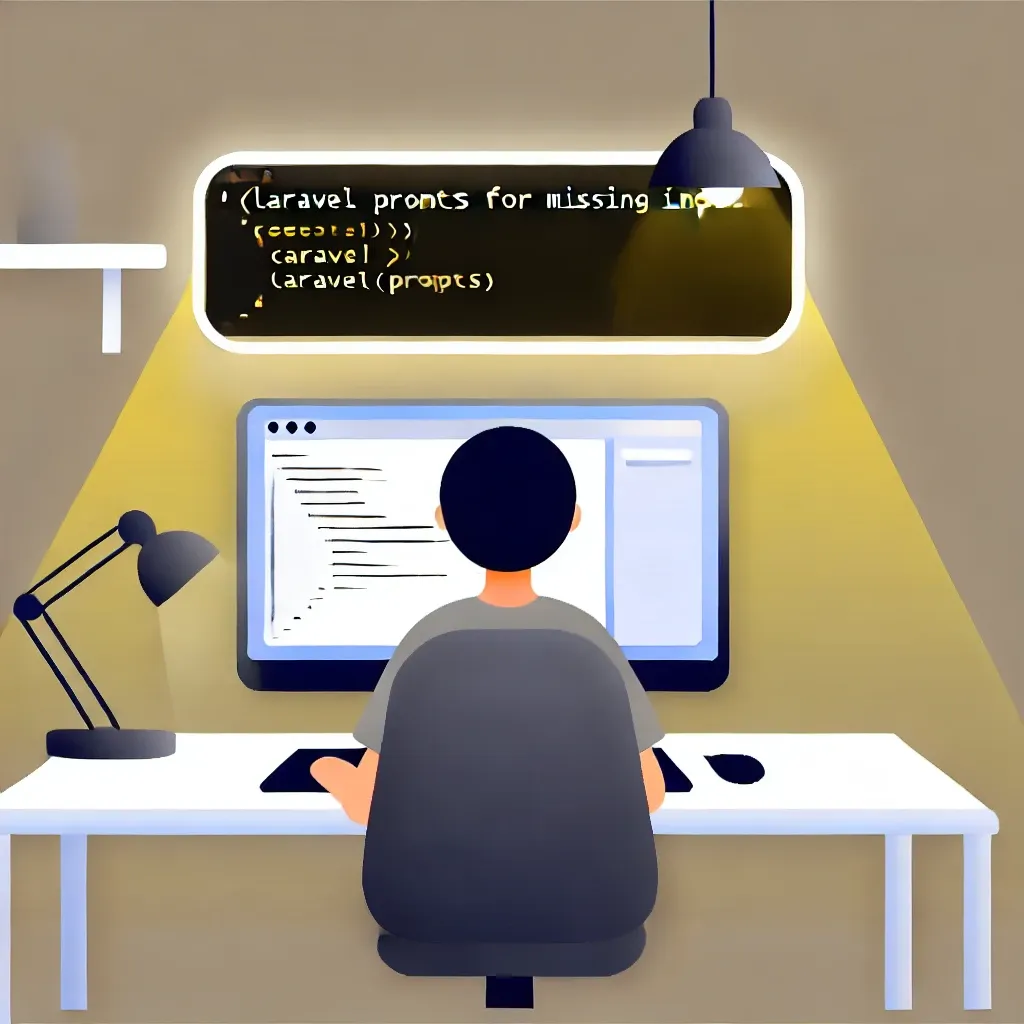
Want to make your Artisan commands more user-friendly? Laravel's PromptsForMissingInput
interface turns missing arguments into interactive prompts! Let's explore this elegant feature.
Basic Implementation
Here's how to add interactive prompts to your command:
use Illuminate\Console\Command;
use Illuminate\Contracts\Console\PromptsForMissingInput;
class SendEmails extends Command implements PromptsForMissingInput
{
protected $signature = 'mail:send {user}';
}
Customizing Prompts
You can customize the prompt messages:
protected function promptForMissingArgumentsUsing(): array
{
return [
'user' => 'Which user ID should receive the mail?'
];
}
Real-World Example
Here's a practical implementation for a deployment command:
class DeployApplication extends Command implements PromptsForMissingInput
{
protected $signature = 'deploy
{environment}
{branch}
{--notify=}';
protected function promptForMissingArgumentsUsing(): array
{
return [
'environment' => [
'Which environment are you deploying to?',
'E.g. staging, production'
],
'branch' => fn () => search(
label: 'Select a branch to deploy:',
placeholder: 'Type to search branches...',
options: fn ($value) => $this->getGitBranches($value)
)
];
}
private function getGitBranches($search)
{
// Simulate Git branch search
$branches = [
'main' => 'main',
'develop' => 'develop',
'feature/user-auth' => 'feature/user-auth',
'hotfix/login-fix' => 'hotfix/login-fix'
];
return array_filter($branches, function($branch) use ($search) {
return str_contains($branch, $search);
});
}
public function handle()
{
$environment = $this->argument('environment');
$branch = $this->argument('branch');
$this->info("Deploying {$branch} to {$environment}...");
// Deployment logic here...
}
}
Usage scenarios:
# With all arguments
php artisan deploy production main
# Without arguments (prompts will appear)
php artisan deploy
Interactive prompts make your commands more user-friendly and help prevent errors from missing arguments.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!