Implementing Real-Time Validation in Laravel Livewire for a Smoother User Experience
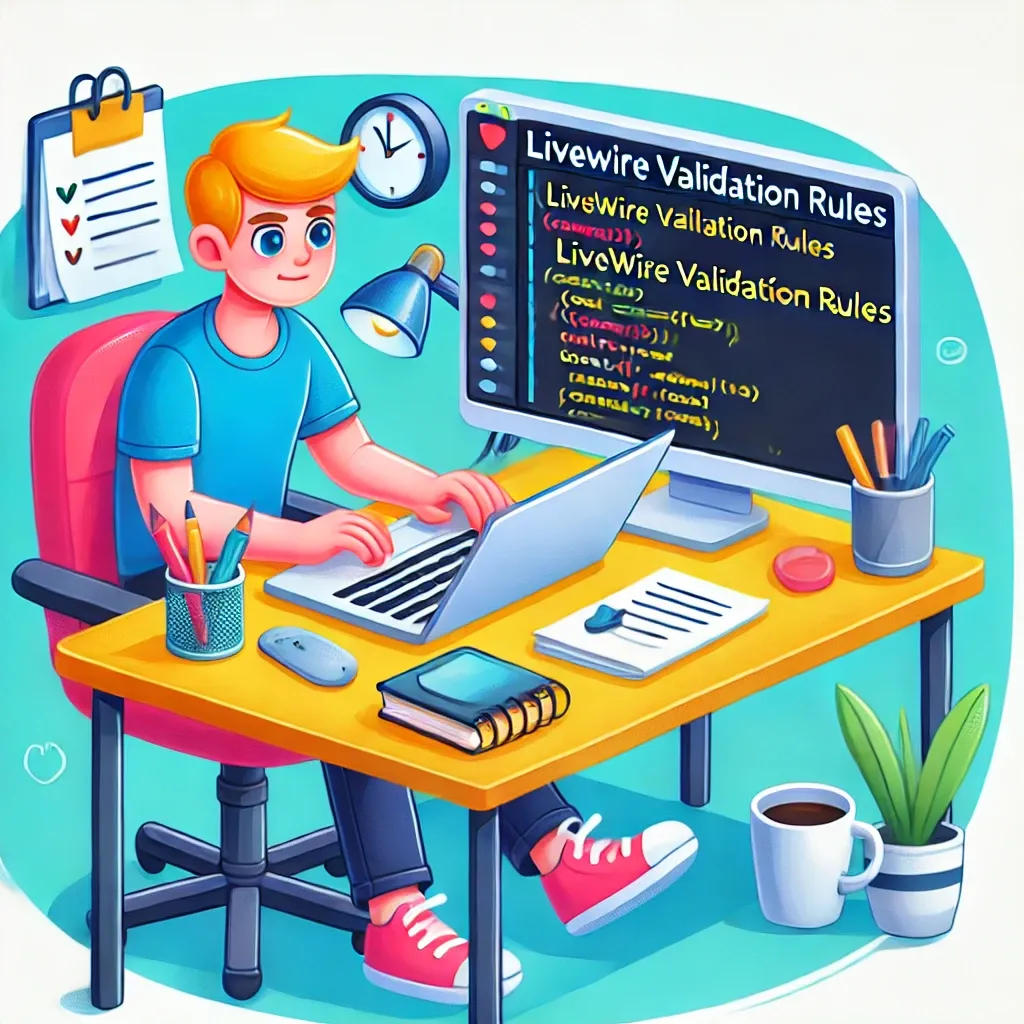
As web developers, we're always looking for ways to enhance user experience. One powerful technique is real-time form validation, which provides instant feedback to users as they fill out forms. Laravel Livewire makes this process incredibly straightforward. In this post, we'll explore how to implement real-time validation in your Livewire components.
Understanding Livewire's Real-Time Validation
Livewire's real-time validation leverages two key features:
- The
$rules
property for defining validation rules - The
updated()
method for triggering validation as users type
Let's dive into how these work together to create a responsive form experience.
Basic Implementation
Here's a basic example of a Livewire component with real-time validation:
use Livewire\Component;
class ContactForm extends Component
{
public $name = '';
public $email = '';
protected $rules = [
'name' => 'required|min:3',
'email' => 'required|email',
];
public function updated($propertyName)
{
$this->validateOnly($propertyName);
}
public function submit()
{
$this->validate();
// Process the form...
}
public function render()
{
return view('livewire.contact-form');
}
}
In the Blade template:
<form wire:submit.prevent="submit">
<div>
<input wire:model.live="name" type="text">
@error('name') <span class="error">{{ $message }}</span> @enderror
</div>
<div>
<input wire:model.live="email" type="email">
@error('email') <span class="error">{{ $message }}</span> @enderror
</div>
<button type="submit">Submit</button>
</form>
Let's break this down:
- We define our form fields as public properties (
$name
and$email
). - The
$rules
property defines the validation rules for each field. - The
updated()
method is called whenever a property changes, validating only that property. - We use
wire:model.live
to bind the input to the property and update in real-time. @error
directives display validation errors for each field.
Advanced Techniques
Custom Validation Messages
You can provide custom error messages for a more tailored user experience:
protected $messages = [
'name.required' => 'How should we address you?',
'name.min' => 'Your name should be at least :min characters.',
'email.required' => 'We\'ll need your email to get in touch.',
'email.email' => 'This doesn\'t look like a valid email address.',
];
Conditional Validation
For more complex forms, you might need conditional validation:
public function updated($propertyName)
{
if ($propertyName === 'email') {
$this->validateOnly($propertyName, [
'email' => ['required', 'email', new UniqueEmail($this->userId)],
]);
} else {
$this->validateOnly($propertyName);
}
}
Debouncing
To prevent excessive validation calls, especially for fields like email, you can use debounce:
<input wire:model.live.debounce.500ms="email" type="email">
This will wait 500 milliseconds after the user stops typing before validating.
Real-World Example: Registration Form
Let's look at a more comprehensive example of a registration form:
class RegistrationForm extends Component
{
public $name = '';
public $email = '';
public $password = '';
public $passwordConfirmation = '';
protected $rules = [
'name' => 'required|min:3',
'email' => 'required|email|unique:users',
'password' => 'required|min:8',
'passwordConfirmation' => 'required|same:password',
];
protected $messages = [
'name.required' => 'Please enter your name.',
'email.unique' => 'This email is already registered. Do you want to login instead?',
'passwordConfirmation.same' => 'The passwords do not match.',
];
public function updated($propertyName)
{
$this->validateOnly($propertyName);
}
public function register()
{
$this->validate();
User::create([
'name' => $this->name,
'email' => $this->email,
'password' => bcrypt($this->password),
]);
session()->flash('message', 'Account successfully created!');
return redirect()->to('/dashboard');
}
public function render()
{
return view('livewire.registration-form');
}
}
This example shows how to handle multiple fields, custom messages, and post-registration actions.
Best Practices
- Be Responsive: Use
wire:model.live
for immediate feedback, but consider debouncing for performance. - Clear Feedback: Provide clear, actionable error messages.
- Progressive Disclosure: Validate fields as they're filled out, not all at once.
- Accessibility: Ensure error messages are accessible to screen readers.
- Server-Side Validation: Always validate on the server side as well for security.
Conclusion
Real-time validation with Laravel Livewire offers a powerful way to enhance your forms and provide immediate feedback to users. By leveraging Livewire's features and following best practices, you can create responsive, user-friendly forms that validate data efficiently and improve overall user experience. Remember, the goal is not just to catch errors, but to guide users towards successful form completion.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!