Image Dimension Validation in Laravel
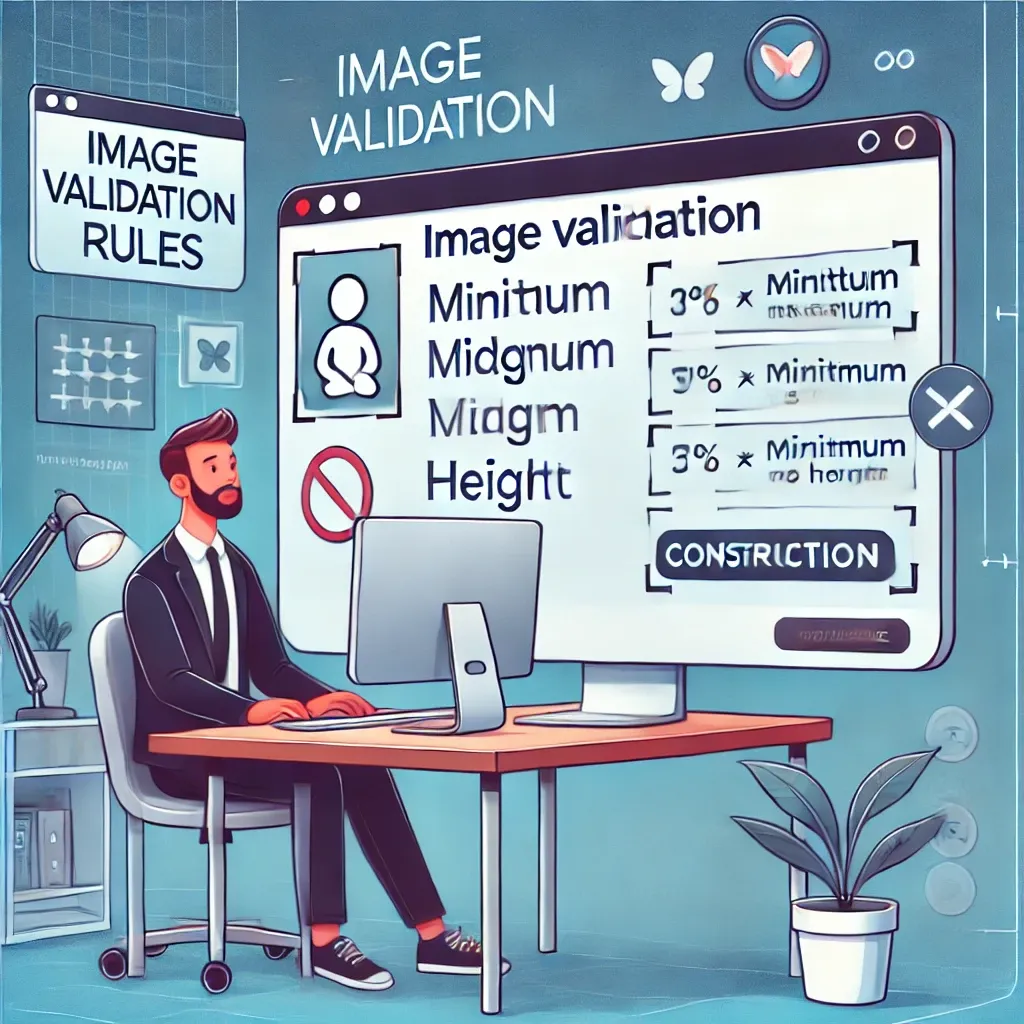
Need to validate image dimensions in your Laravel application? The dimensions validation rule provides a flexible way to ensure uploaded images meet your specific requirements.
When handling image uploads, it's crucial to validate dimensions to maintain consistency and performance in your application. Laravel's dimension validation rule makes this process straightforward, whether you're handling profile pictures, product images, or banner uploads.
Let's see how it works:
use Illuminate\Support\Facades\Validator;
use Illuminate\Validation\Rule;
// Basic validation
$validator = Validator::make($request->all(), [
'profile_photo' => [
'required',
Rule::dimensions()
->minWidth(400)
->minHeight(400)
->maxWidth(2000)
->maxHeight(2000)
]
]);
Real-World Example
Here's how you might implement image validation in a content management system:
class ImageValidator
{
public function getValidationRules(string $type): array
{
return match($type) {
'profile' => [
'required',
'image',
Rule::dimensions()
->minWidth(400)
->minHeight(400)
->maxWidth(1200)
->maxHeight(1200)
->ratio(1) // Square images only
],
'banner' => [
'required',
'image',
Rule::dimensions()
->width(1920)
->height(480) // Fixed dimensions for banners
],
'article' => [
'required',
'image',
Rule::dimensions()
->minWidth(800)
->maxWidth(2400)
->ratio(16/9) // Widescreen ratio
],
default => throw new InvalidArgumentException('Invalid image type')
};
}
public function validate(Request $request, string $type)
{
return Validator::make(
$request->all(),
['image' => $this->getValidationRules($type)]
);
}
}
// Usage in controller
public function store(Request $request)
{
$validator = (new ImageValidator)->validate($request, 'article');
if ($validator->fails()) {
return back()
->withErrors($validator)
->withInput();
}
// Process the valid image
}
The dimensions rule provides comprehensive control over image validation, ensuring your application handles images consistently. Think of it as a quality control system for your image uploads - it helps maintain visual consistency while preventing oversized or incorrectly proportioned images.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!