HTTP Response Shorthands in Laravel: Simplified Fakes
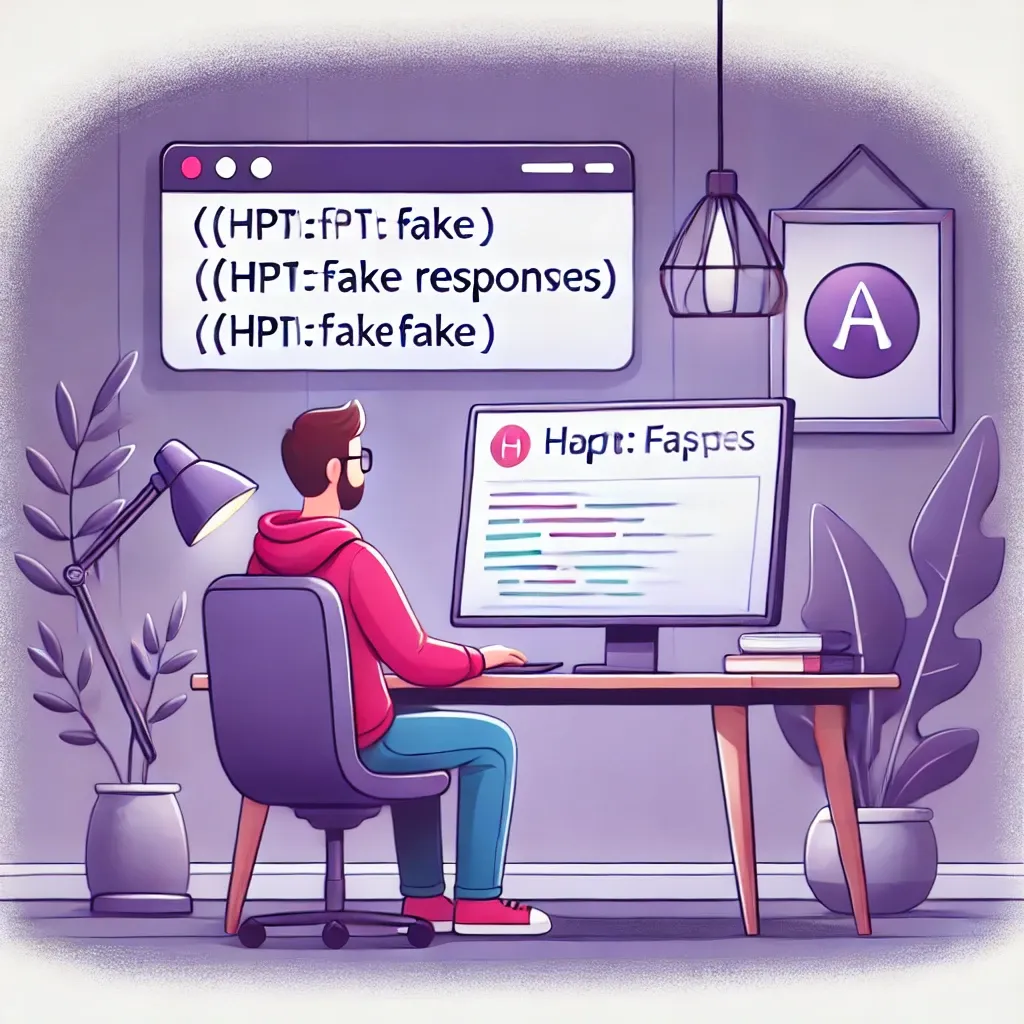
Want to write cleaner HTTP test mocks? Laravel's new shorthand syntax for faking HTTP responses cuts down the boilerplate and makes your tests more readable. Let's dive into these elegant shortcuts.
Basic Usage
use Illuminate\Support\Facades\Http;
Http::fake([
'google.com' => 'Hello World',
'github.com' => ['foo' => 'bar'],
'forge.laravel.com' => 204,
]);
Real-World Example
Here's how to use these shorthands in a practical testing scenario:
class ExternalServiceTest extends TestCase
{
public function test_external_api_integration()
{
Http::fake([
// Simple text responses
'api.weather.com/*' => 'Sunny',
// JSON responses
'api.users.com/*' => [
'users' => [
['id' => 1, 'name' => 'John'],
['id' => 2, 'name' => 'Jane']
]
],
// Status codes for simple endpoints
'api.health.com/ping' => 200,
'api.maintenance.com/*' => 503,
// Mix different response types
'api.complex.com/users' => ['status' => 'active'],
'api.complex.com/error' => 400,
'api.complex.com/text' => 'Error occurred'
]);
// Your test assertions here
$response = Http::get('api.weather.com/forecast');
$this->assertEquals('Sunny', $response->body());
$users = Http::get('api.users.com/list');
$this->assertCount(2, $users['users']);
$health = Http::get('api.health.com/ping');
$this->assertEquals(200, $health->status());
}
}
These new shorthands make your HTTP fakes more concise and easier to read while maintaining all the functionality.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!