Handling Non-Existent Models with Laravel's existsOr Method
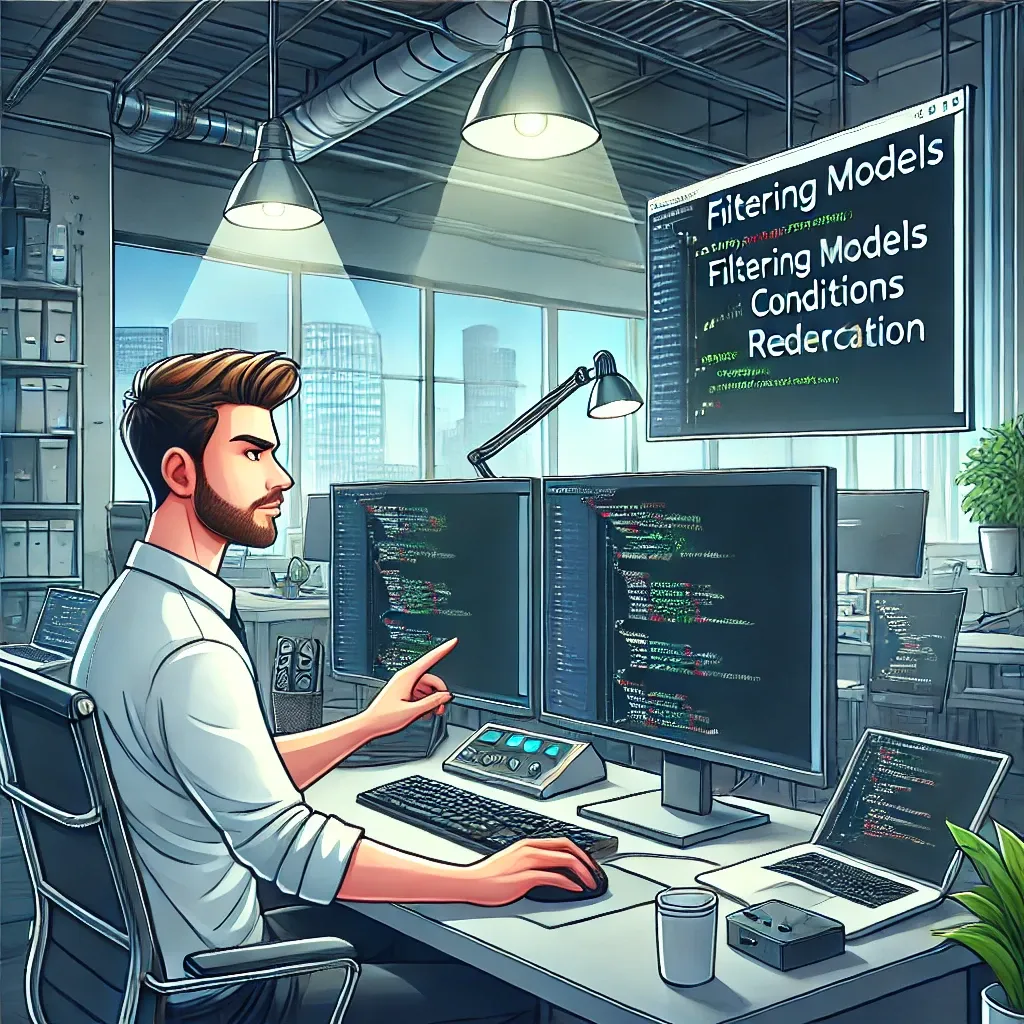
Need to handle cases when a model doesn't exist? Laravel's existsOr method provides an elegant way to execute code when a model query returns no results.
Basic Usage
Handle non-existent models with a closure:
Model::where(...)
->existsOr(function () {
// Some logic if the model does not exist
});
Real-World Example
Here's how you might use it in a product management system:
class ProductController extends Controller
{
public function checkAvailability($sku)
{
return Product::where('sku', $sku)
->existsOr(function () use ($sku) {
Log::info("Product lookup attempted for non-existent SKU: {$sku}");
return response()->json([
'available' => false,
'message' => 'Product not found in catalog'
], 404);
});
}
public function assignToCategory($productId, $categoryId)
{
return Product::where('id', $productId)
->existsOr(function () {
throw new ProductNotFoundException(
'Cannot assign category to non-existent product'
);
});
}
public function checkStock($productId)
{
return Product::where('id', $productId)
->where('status', 'active')
->existsOr(function () use ($productId) {
event(new ProductLookupFailed($productId));
return redirect()
->route('products.index')
->with('error', 'Product not available');
});
}
}
The existsOr method simplifies handling cases where your model queries return no results.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!