Handling Multiple Values in Laravel Artisan Commands
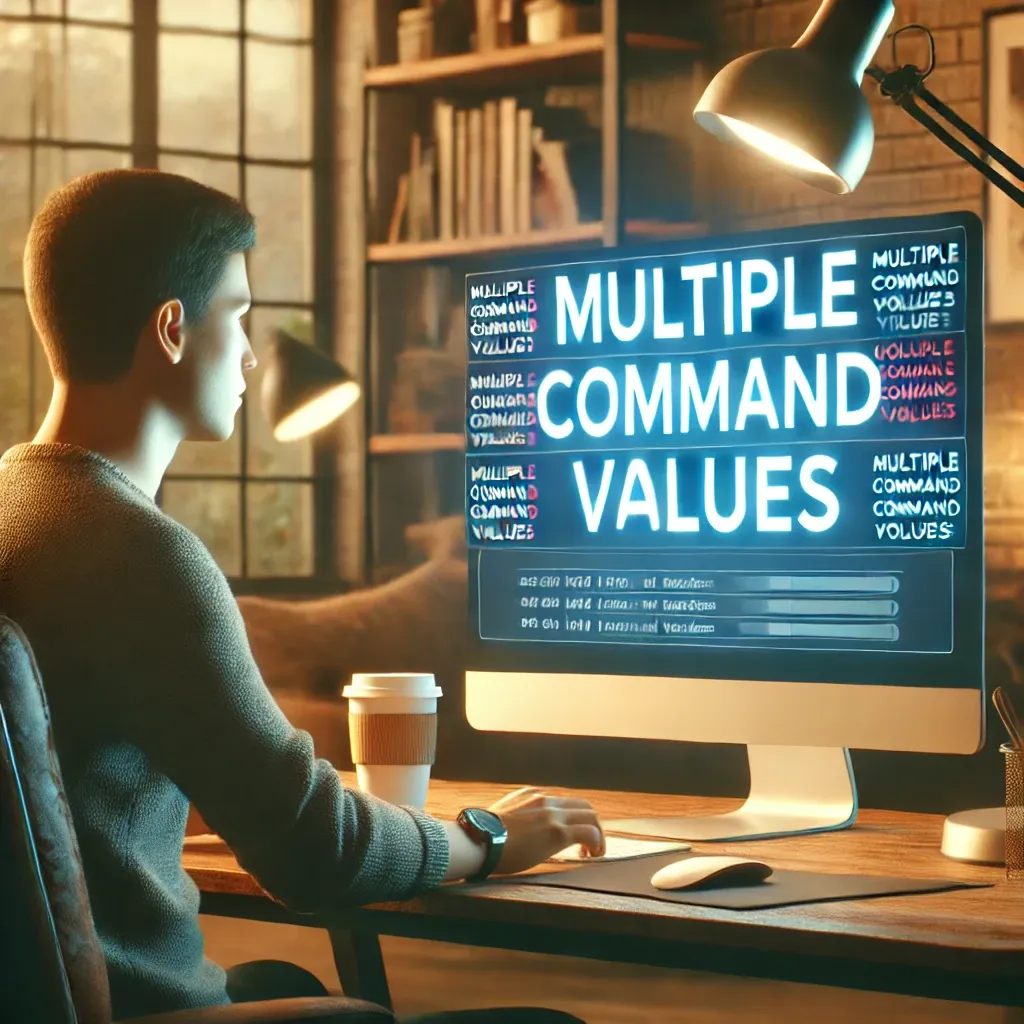
Need to accept multiple values in your Artisan commands? Laravel's array syntax makes it simple! Let's explore how to handle multiple arguments and options in your commands.
Multiple Arguments
For arguments that accept multiple values, use the *
character:
protected $signature = 'mail:send {user*}';
This allows command usage like:
php artisan mail:send 1 2 3
Optional Multiple Arguments
Make multiple arguments optional by combining ?
and *
:
protected $signature = 'mail:send {user?*}';
Multiple Option Values
For options accepting multiple values:
protected $signature = 'mail:send {--id=*}';
Usage:
php artisan mail:send --id=1 --id=2 --id=3
Real-World Example
Here's a practical example for managing user roles:
class AssignUserRoles extends Command
{
protected $signature = 'users:assign-roles
{users*: User IDs to update}
{--R|role=*: Roles to assign}
{--team=*: Team IDs to scope roles to}';
public function handle()
{
$userIds = $this->argument('users');
$roles = $this->option('role');
$teams = $this->option('team');
$this->info("Assigning roles to " . count($userIds) . " users...");
foreach ($userIds as $userId) {
$user = User::findOrFail($userId);
foreach ($roles as $role) {
if ($teams) {
foreach ($teams as $teamId) {
$user->assignRole($role, Team::find($teamId));
$this->info("Assigned {$role} to User {$userId} in Team {$teamId}");
}
} else {
$user->assignRole($role);
$this->info("Assigned {$role} to User {$userId}");
}
}
}
}
}
Usage examples:
# Assign multiple roles to multiple users
php artisan users:assign-roles 1 2 3 --role=editor --role=writer
# Assign roles in specific teams
php artisan users:assign-roles 1 2 --role=editor --team=5 --team=6
Multiple value support makes your commands more flexible and powerful, perfect for batch operations.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!