Graceful Process Handling in Laravel: Mastering the Stop Command
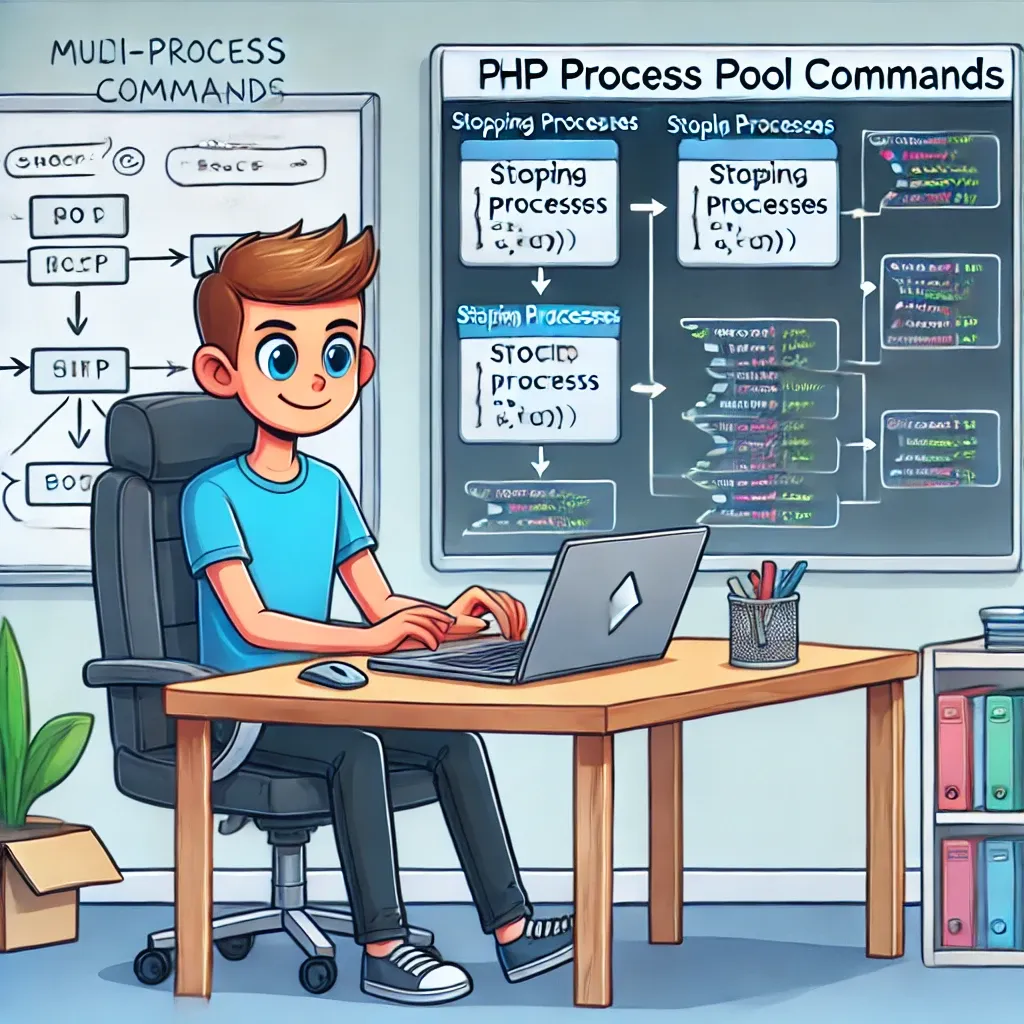
Ever started a long-running process in Laravel and wondered, "How do I stop this thing safely?" Well, you're in luck! Laravel provides some nifty tools to start and stop processes gracefully. Let's dive in!
Stopping a Single Process
First, let's look at how to stop a single process:
$process = Process::timeout(120)->start('bash import.sh');
// ... Some code here ...
$process->stop();
What's happening here?
- We start a process that runs 'import.sh' with a 2-minute timeout
- When we're ready, we call
stop()
to end it gracefully
Handling Process Pools
But what if you're juggling multiple processes? That's where Process Pools come in handy:
$this->pool = Process::pool(function (Pool $pool) {
$pool->path(base_path())->command('sleep 5');
$pool->path(base_path())->command('sleep 10');
})->start();
// Stop all processes in the pool gracefully
$this->pool->stop();
In this example:
- We create a pool with two processes (one sleeps for 5 seconds, the other for 10)
- We start the pool
- When we're done, we stop all processes in the pool with one command
Getting Fancy with Signals
Want more control? You can specify a signal when stopping processes:
// Stop the processes with a specific signal
$this->pool->stop($signal);
This is useful if you need to send a specific termination signal to your processes.
Real-World Example: Background Job Processing
Let's say you're building a system to process large files in the background:
use Illuminate\Support\Facades\Process;
class FileProcessor
{
protected $pool;
public function processFiles(array $files)
{
$this->pool = Process::pool(function (Pool $pool) use ($files) {
foreach ($files as $file) {
$pool->command("php artisan process:file {$file}");
}
})->start();
// Monitor progress...
if ($this->shouldStop()) {
$this->pool->stop();
return 'Processing stopped';
}
return 'Processing completed';
}
private function shouldStop()
{
// Logic to determine if processing should stop
}
}
In this example:
- We create a pool of processes to handle multiple files
- Each process runs an Artisan command to process a file
- We have a method to check if we should stop processing
- If we need to stop, we call
stop()
on the entire pool
This approach allows you to process multiple files concurrently and stop all processes gracefully if needed.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!