Graceful Error Handling with Laravel's rescue Function
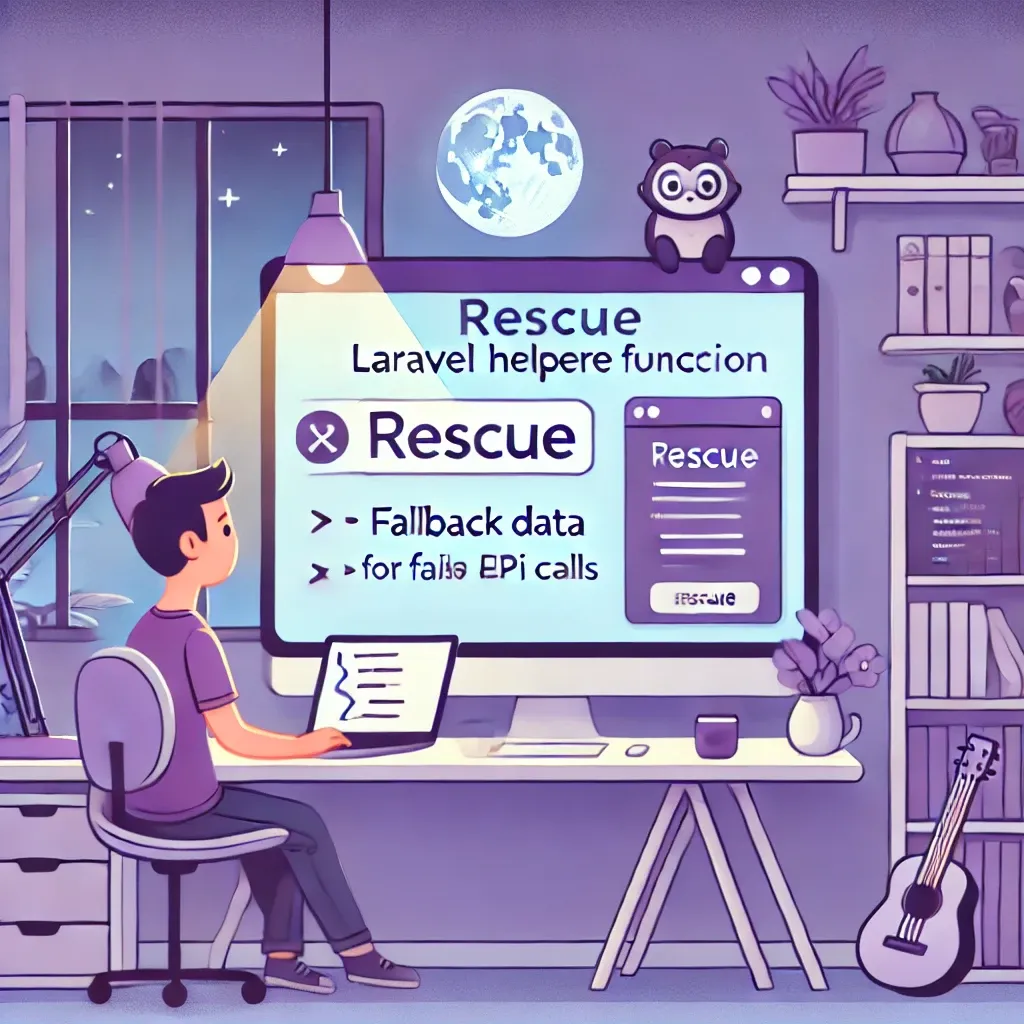
Exception handling shouldn't break your application's flow. Laravel's rescue function provides a clean way to execute potentially risky code while maintaining smooth operation.
Basic Usage
Here's how to use the rescue function:
// Simple usage
return rescue(function () {
return $this->method();
});
// With default value
return rescue(function () {
return $this->method();
}, false);
// With fallback closure
return rescue(function () {
return $this->method();
}, function () {
return $this->failure();
});
Real-World Example
Here's a practical implementation for an API integration:
class ExternalServiceIntegration
{
public function fetchUserData($userId)
{
return rescue(function () use ($userId) {
$response = Http::get("api.example.com/users/{$userId}");
return $response->json();
}, [
'id' => $userId,
'status' => 'unavailable',
'error' => 'Could not fetch user data'
]);
}
public function syncProducts()
{
return rescue(
function () {
$products = $this->api->getProducts();
foreach ($products as $product) {
$this->updateLocalProduct($product);
}
return true;
},
function () {
Cache::put('sync_failed', true, now()->addHour());
return false;
},
report: function (Throwable $e) {
return !($e instanceof TemporaryException);
}
);
}
}
// Usage in controller
class ProductController extends Controller
{
public function index(ExternalServiceIntegration $service)
{
$synced = $service->syncProducts();
return response()->json([
'success' => $synced,
'message' => $synced
? 'Products synchronized successfully'
: 'Sync failed, using cached data'
]);
}
}
The rescue function provides a clean way to handle potential errors while maintaining readable code structure.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!