Getting Default Locale and Currency in Laravel with Number Facade
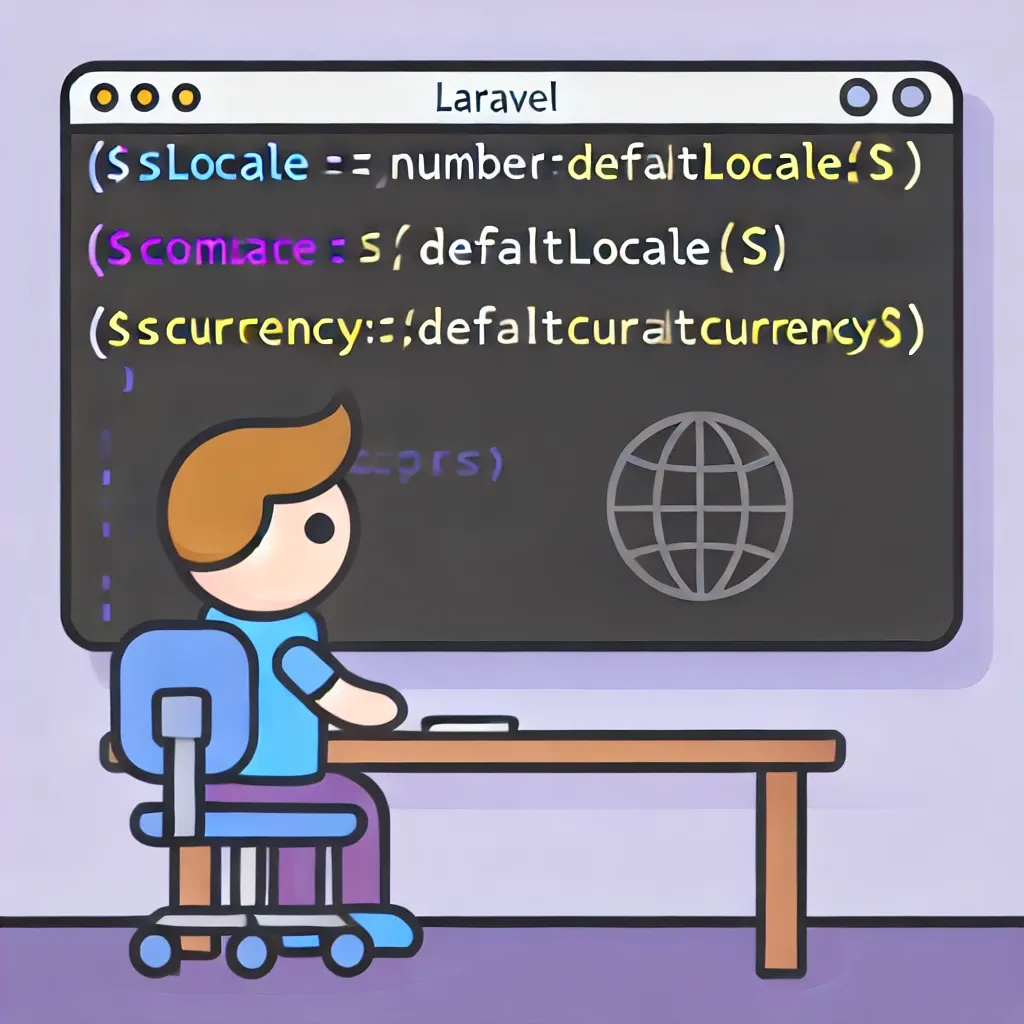
Working with internationalization in Laravel? The Number facade just got smarter with two new helper methods! Let's explore how these handy additions can make working with locales and currencies even easier.
The New Helper Methods
The Number facade now provides two straightforward methods to retrieve defaults:
use Illuminate\Support\Number;
// Get the default locale
$locale = Number::defaultLocale();
// Get the default currency
$currency = Number::defaultCurrency();
Real-World Example
Here's how you might use these methods in an e-commerce application:
class PriceController extends Controller
{
public function display(Product $product, Request $request)
{
// Use user's preferred currency if set, otherwise use default
$currency = $request->user()->preferred_currency
?? Number::defaultCurrency();
// Use user's preferred locale if set, otherwise use default
$locale = $request->user()->preferred_locale
?? Number::defaultLocale();
return [
'price' => Number::currency($product->price, in: $currency),
'locale_info' => "Displayed in {$locale} format",
'system_defaults' => [
'locale' => Number::defaultLocale(),
'currency' => Number::defaultCurrency()
]
];
}
}
These new helper methods make it easy to access and work with your application's default locale and currency settings. Whether you're building an international platform or just need to know your system defaults, these methods have got you covered!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!