Generate Collections on the Fly with Laravel's times Method
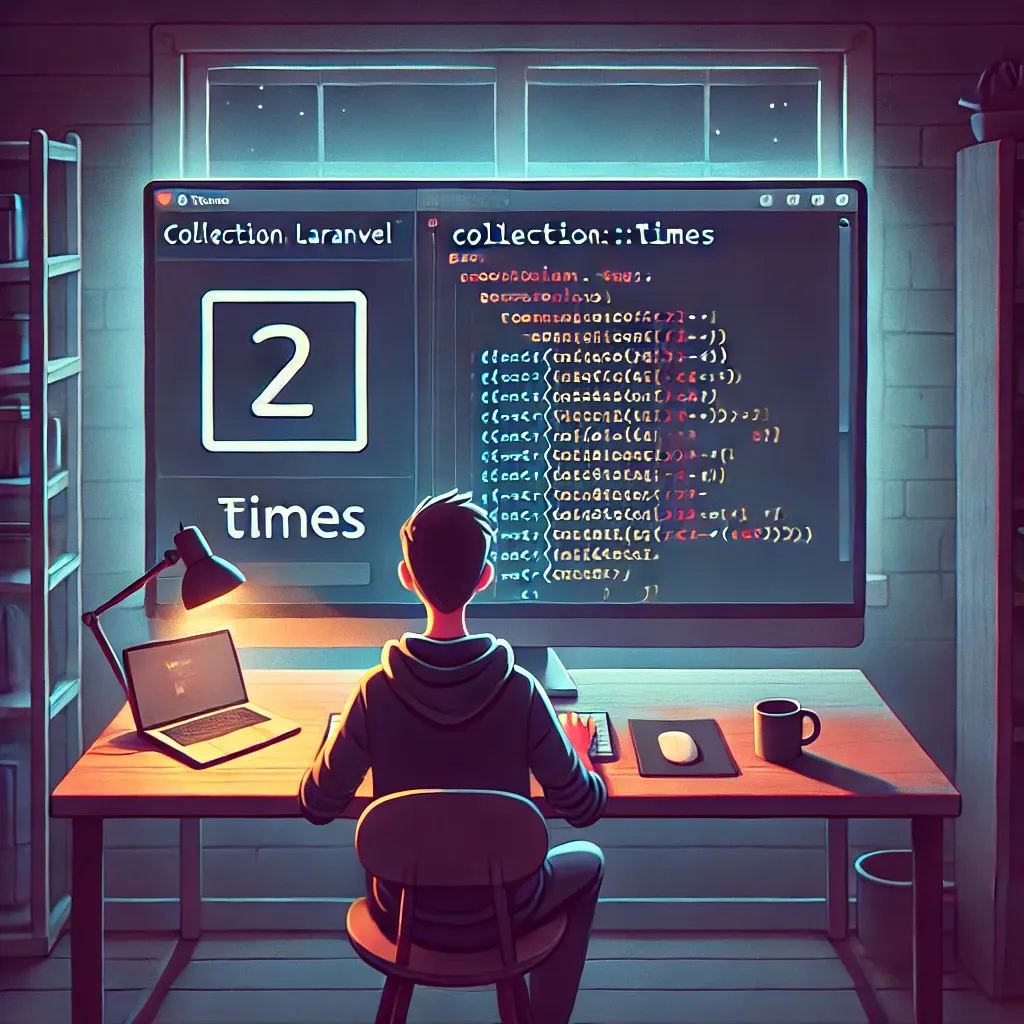
Need to create a collection with calculated values? Laravel's times
method lets you generate collections dynamically!
Basic Usage
Here's the simplest way to use times
:
$collection = Collection::times(10, function (int $number) {
return $number * 9;
});
// [9, 18, 27, 36, 45, 54, 63, 72, 81, 90]
Real-World Example
Here's how you might use it in a date slot generator:
class AppointmentSlotGenerator
{
public function generateDailySlots(
string $startTime = '09:00',
int $slotCount = 8,
int $intervalMinutes = 60
) {
return Collection::times($slotCount, function (int $index) use ($startTime, $intervalMinutes) {
$time = Carbon::parse($startTime)
->addMinutes(($index) * $intervalMinutes);
return [
'slot_id' => $index + 1,
'start_time' => $time->format('H:i'),
'end_time' => $time->addMinutes($intervalMinutes)->format('H:i'),
'is_available' => true
];
});
}
}
// Usage
$generator = new AppointmentSlotGenerator();
$slots = $generator->generateDailySlots('10:00', 6, 30);
$slots->each(function ($slot) {
$this->info("Slot {$slot['slot_id']}: {$slot['start_time']} - {$slot['end_time']}");
});
The times
method is perfect for generating sequences, creating test data, or any scenario where you need calculated values in a collection.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!