Force HTTPS URLs in Laravel
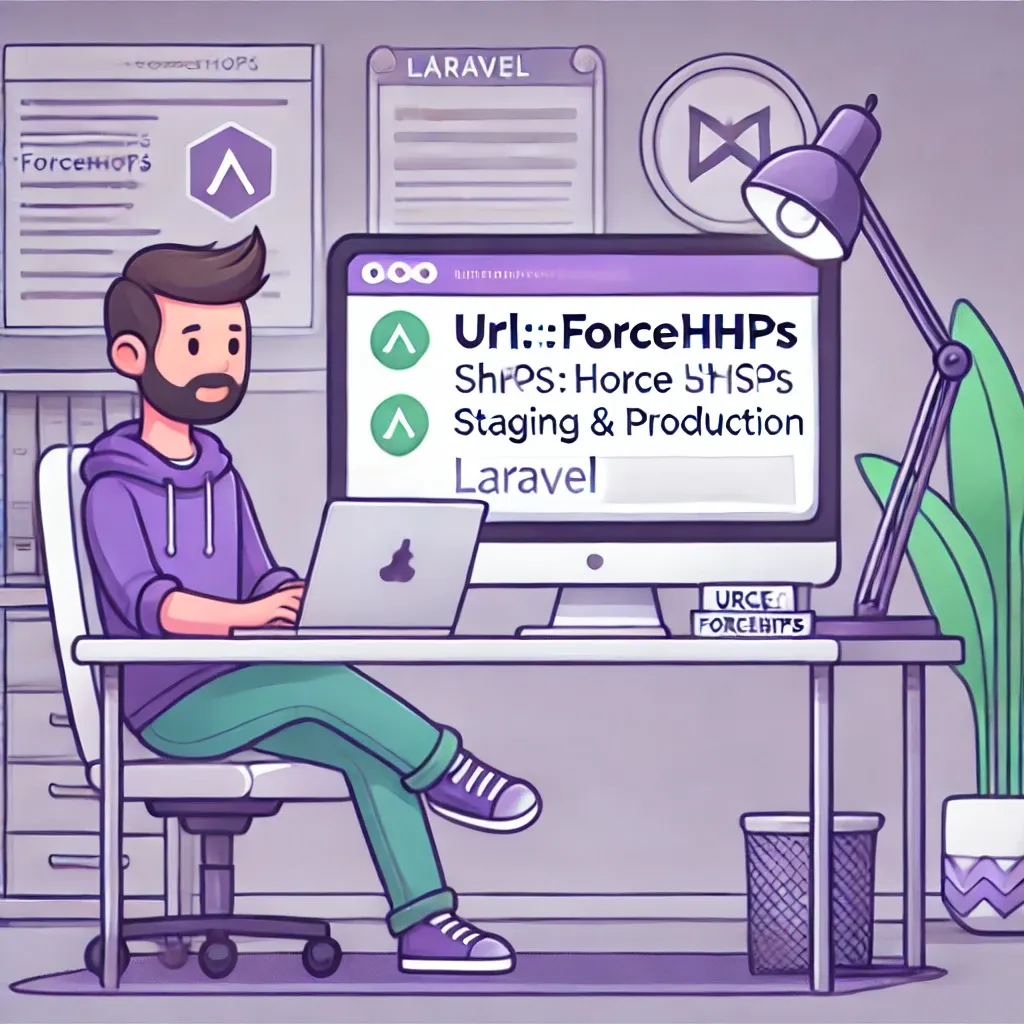
Learn how to ensure your application's URLs are secure by automatically enforcing HTTPS based on your environment. Let's explore Laravel's new forceHttps
method.
Basic Usage
Here's how to enforce HTTPS based on environment:
use Illuminate\Support\Facades\URL;
URL::forceHttps($this->app->isProduction());
Environment-Specific Configuration
URL::forceHttps(
$this->app->environment('staging', 'production')
);
Real-World Example
Here's how to implement comprehensive HTTPS enforcement in your application:
namespace App\Providers;
use Illuminate\Support\Facades\URL;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function boot()
{
// Basic production check
URL::forceHttps($this->app->isProduction());
// Or more specific environment control
URL::forceHttps(
$this->app->environment(['staging', 'production', 'demo'])
&& !$this->app->environment('testing')
);
// You might also want to combine it with other security headers
if ($this->app->isProduction()) {
$this->app['request']->server->set('HTTPS', true);
// Add security headers
$this->app['router']->middleware(function ($request, $next) {
$response = $next($request);
return $response->withHeaders([
'Strict-Transport-Security' => 'max-age=31536000; includeSubDomains',
'X-Frame-Options' => 'SAMEORIGIN',
'X-Content-Type-Options' => 'nosniff'
]);
});
}
}
}
The forceHttps
method provides a clean, declarative way to ensure your application's URLs are secure in production environments.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!