Fluent Request Data Handling in Laravel
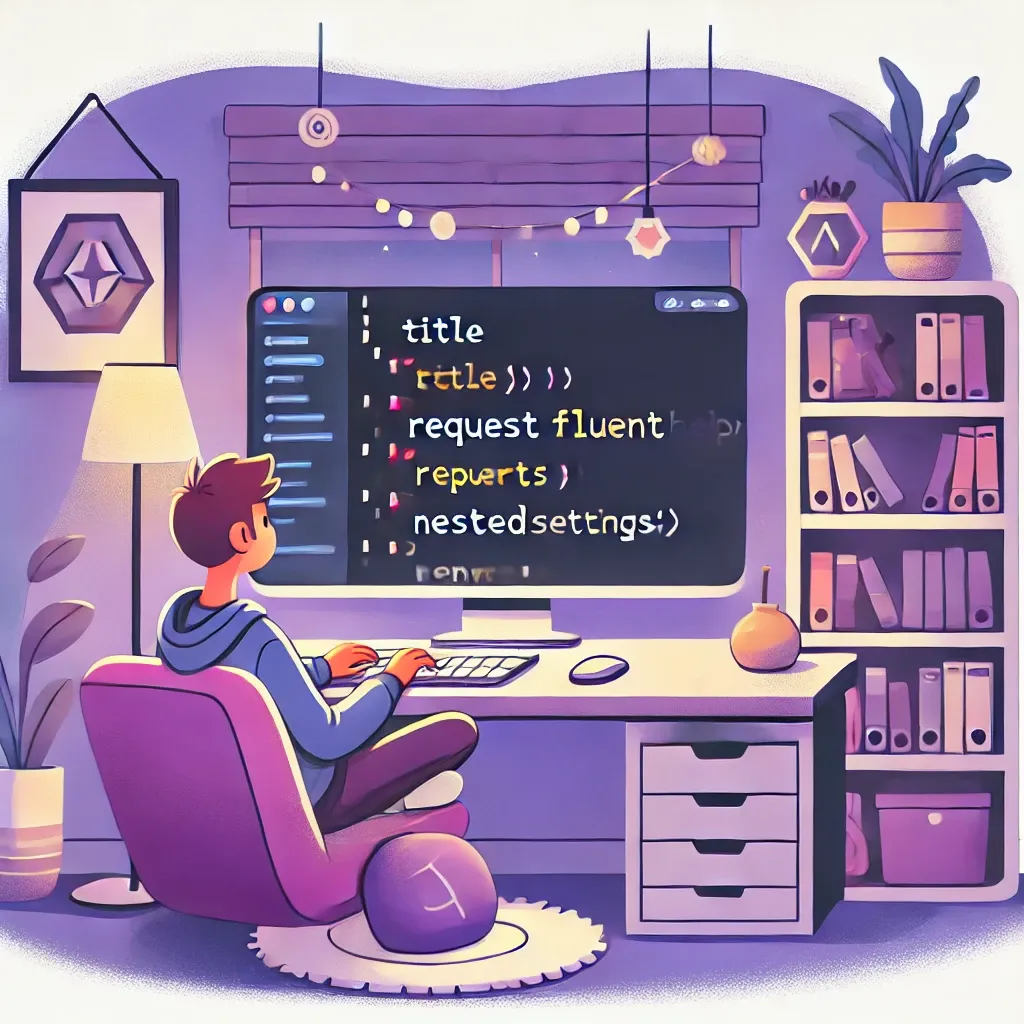
Managing request data just got smoother in Laravel. The new fluent() method on the Request class lets you handle input data with grace, providing null-safe access and enhanced transportability.
Basic Usage
Transform your request data into a fluent instance:
/** @var Illuminate\Http\Request $request */
$data = $request->fluent();
// Access properties safely
$data->title;
$data->body;
Real-World Example
Here's how to implement fluent request handling in a comment system:
class CommentController extends Controller
{
public function store(CommentRequest $request)
{
$comment = (new CreateComment)->handle(
$request->fluent()
);
return response()->json($comment);
}
}
class CreateComment
{
public function handle(Fluent $data): Comment
{
return Comment::create([
'title' => $data->title,
'body' => $data->body,
'user_id' => auth()->id(),
'metadata' => [
'browser' => $data->browser,
'platform' => $data->platform
]
]);
}
}
class ProcessCommentJob implements ShouldQueue
{
public function __construct(
public Fluent $data
) {}
public function handle()
{
// Safe access to nullable fields
$notifications = $this->data->notifications ?? 'default';
$priority = $this->data->priority ?? 'normal';
return Comment::process([
'content' => $this->data->body,
'settings' => [
'notify' => $notifications,
'priority' => $priority
]
]);
}
}
The fluent() method makes request data handling more elegant and safer, especially when dealing with nullable values.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!