Fluent Email Validation in Laravel with the Email Rule Object
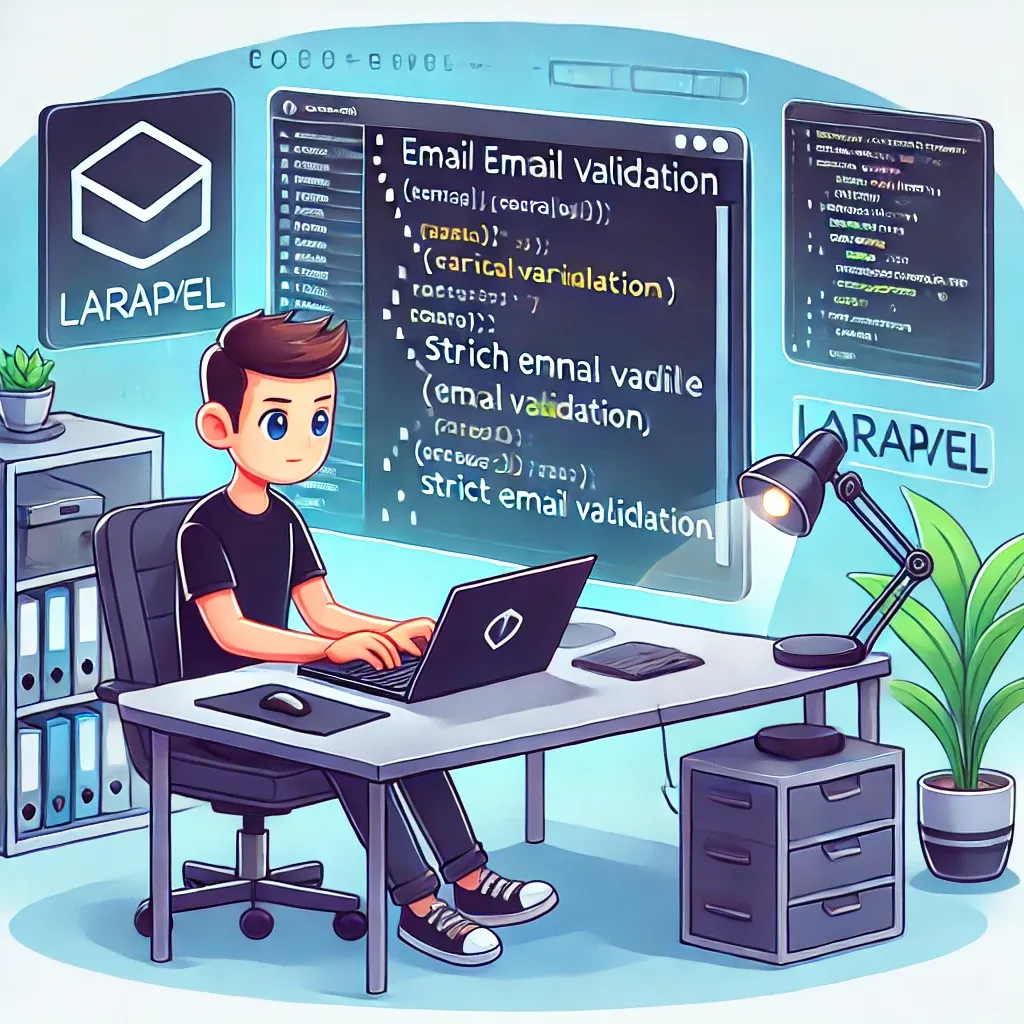
Need more control over email validation in Laravel? The new Email rule object provides a fluent, extensible way to validate email addresses with precise requirements.
Email validation often requires different levels of strictness - from basic format checking to DNS verification and spoofing prevention. Laravel's new Email rule object simplifies this process by providing a fluent interface that makes your validation rules more readable and maintainable.
Let's see how it works:
use Illuminate\Validation\Rule;
$request->validate([
'email' => ['required', 'string', Rule::email()->strict()->dns()]
]);
Real-World Example
Here's how you might implement custom email validation rules for different user types:
class AppServiceProvider extends ServiceProvider
{
public function boot()
{
Email::macro('employee', function () {
return Email::default()
->strict()
->dns()
->rules('ends_with:@company.com,@subsidiary.com');
});
Email::macro('client', function () {
return Email::strictSecurity()
->rules('not_ends_with:@competitor.com');
});
Email::macro('enterprise', function () {
return Email::default()
->dns()
->spoof()
->filter()
->rules('ends_with:@verified-domains.com');
});
}
}
class RegistrationController extends Controller
{
public function store(Request $request)
{
$request->validate([
'personal_email' => ['required', Rule::email()->strict()],
'work_email' => ['required', Email::employee()],
'billing_email' => ['required', Email::enterprise()]
]);
// Registration logic
}
}
The Email rule object brings several advantages over the traditional array-based validation. It provides a more intuitive interface for combining validation rules, makes the code more readable, and allows for easy extension through macros. This is particularly useful when dealing with different email validation requirements across your application.
Think of it as building blocks for email validation - you can mix and match different validation aspects (DNS checks, spoofing prevention, strict mode) while maintaining clean, understandable code. This becomes especially valuable when:
- Managing different email validation requirements for different user types
- Implementing custom validation rules for specific domains
- Ensuring security measures like DNS and spoofing checks
- Creating reusable validation patterns across your application
For example, instead of remembering which combination of array elements creates the most secure validation, you can simply use Email::strictSecurity()
and know you're getting the highest level of validation.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!