Fluent Date Validation with Laravel's Date Rule
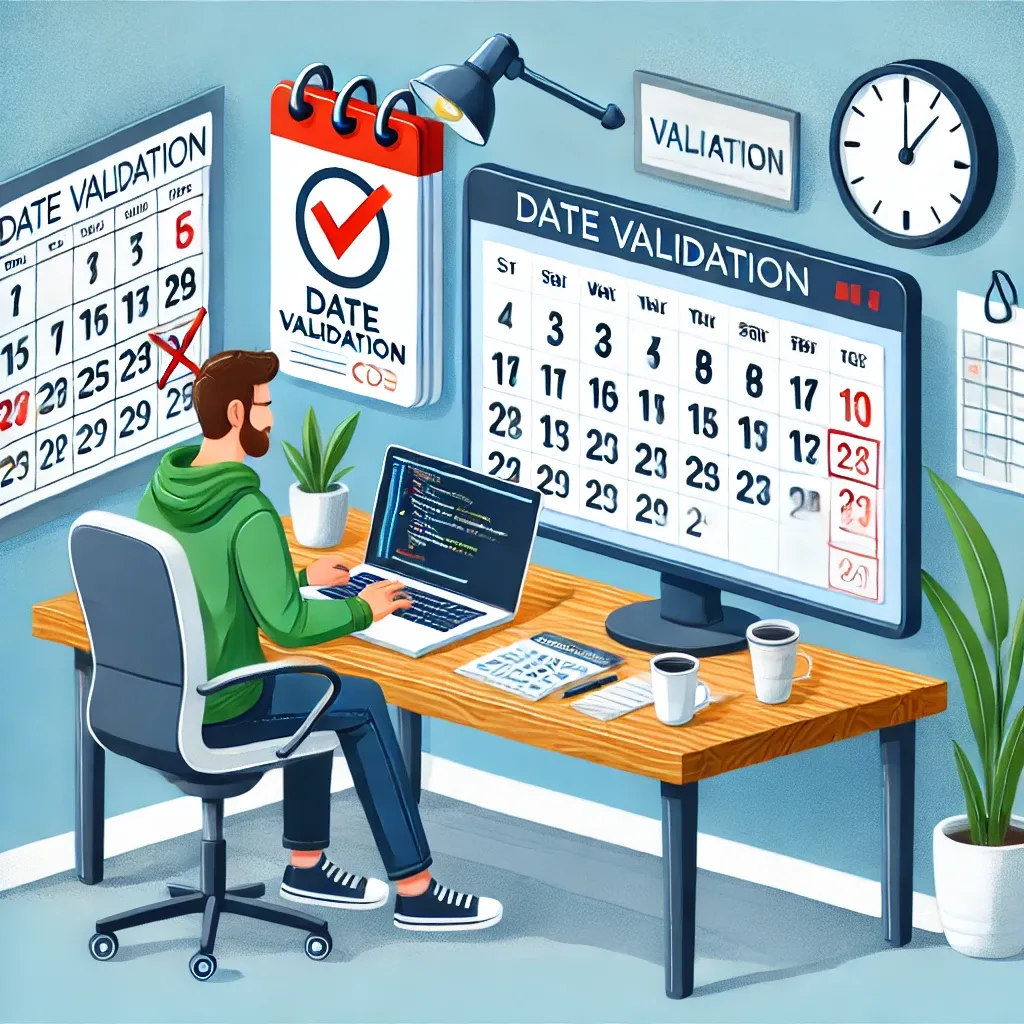
Need more control over date validation? Laravel's new Date rule brings a fluent, chainable interface for creating complex date validation requirements with minimal code.
Date validation often requires multiple conditions like format checking, range validation, and relative date comparisons. Laravel's new Date rule simplifies these requirements by providing a clean, chainable syntax for all your date validation needs.
Let's see how it works:
use Illuminate\Validation\Rules\Date;
public function rules()
{
return [
'start_date' => [
'required',
new Date // Basic date validation
],
'end_date' => [
'required',
(new Date)
->after('start_date')
->before('2025-01-01')
],
'birth_date' => [
'required',
(new Date)
->format('d/m/Y')
->beforeToday()
]
];
}
Real-World Example
Here's how you might use it in an event management system:
class EventRegistrationController extends Controller
{
public function store(Request $request)
{
$request->validate([
'event_date' => [
'required',
(new Date)
->format('Y-m-d')
->afterToday()
->before('2025-12-31')
],
'registration_deadline' => [
'required',
(new Date)
->beforeOrEqual('event_date')
->after('today')
],
'early_bird_until' => [
'required',
(new Date)
->between('today', 'registration_deadline')
],
'cancellation_date' => [
'required',
(new Date)
->betweenOrEqual('registration_deadline', 'event_date')
]
]);
// Create event logic...
}
}
The Date rule provides a comprehensive set of methods that make complex date validation requirements easy to read and maintain. Think of it as a dedicated toolkit for date validation - whether you need simple format validation or complex date relationships, there's a method for that.
This becomes especially valuable when:
- Validating date ranges for bookings or events
- Ensuring logical date sequences
- Handling different date formats
- Working with relative dates
- Implementing age restrictions
For example, instead of writing custom validation logic for checking if a date falls within a specific range, you can simply chain the appropriate methods together.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!