Fluent Array Handling in Laravel: The fluent() Helper
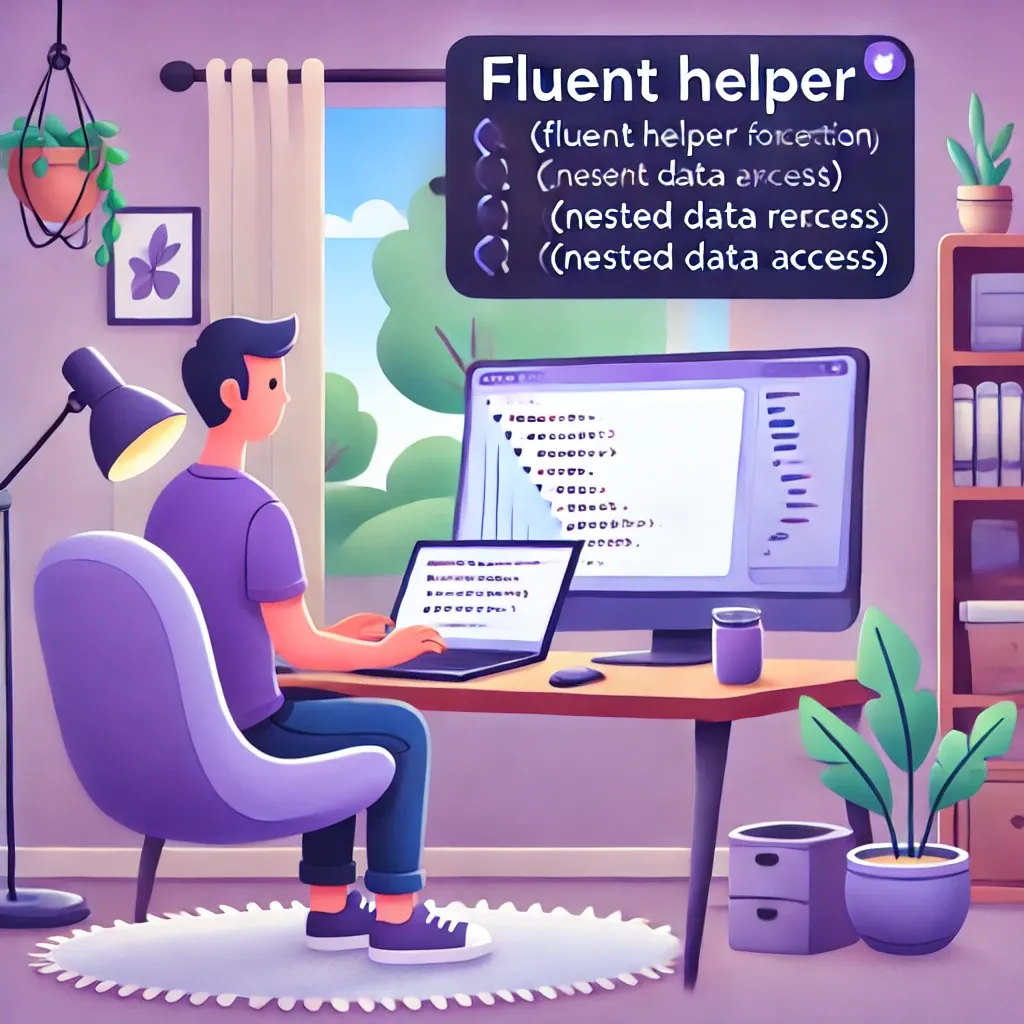
Working with nested arrays in Laravel just got smoother with the introduction of the fluent() helper function, providing a more intuitive way to access and manipulate multi-dimensional data.
Basic Usage
Here's how to work with multi-dimensional arrays using the fluent helper:
$data = [
'user' => [
'name' => 'Philo',
'address' => [
'city' => 'Amsterdam',
'country' => 'Netherlands',
]
],
'posts' => [
['title' => 'Post 1'],
['title' => 'Post 2']
]
];
// Access nested data
fluent($data)->user;
fluent($data)->get('user.name');
// Chain with collections
fluent($data)->collect('posts')->pluck('title');
// JSON conversion
fluent($data)->scope('user.address')->toJson();
Real-World Example
Here's a practical implementation for a user profile system:
class ProfileController extends Controller
{
public function show(User $user)
{
$profileData = [
'user' => [
'info' => [
'name' => $user->name,
'email' => $user->email,
'joined' => $user->created_at
],
'preferences' => [
'notifications' => $user->notification_preferences,
'theme' => $user->theme_settings
]
],
'stats' => [
'posts' => $user->posts->count(),
'comments' => $user->comments->count(),
'likes' => $user->likes->count()
]
];
$profile = fluent($profileData);
return response()->json([
'name' => $profile->get('user.info.name'),
'email' => $profile->get('user.info.email'),
'theme' => $profile->get('user.preferences.theme'),
'engagement' => $profile->scope('stats')->toArray(),
'notifications' => $profile
->collect('user.preferences.notifications')
->filter(fn($enabled) => $enabled)
->keys()
]);
}
}
The fluent helper makes complex array operations more readable and maintainable.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!