Flexible API Responses with Laravel: Mastering Conditional Attributes in API Resources
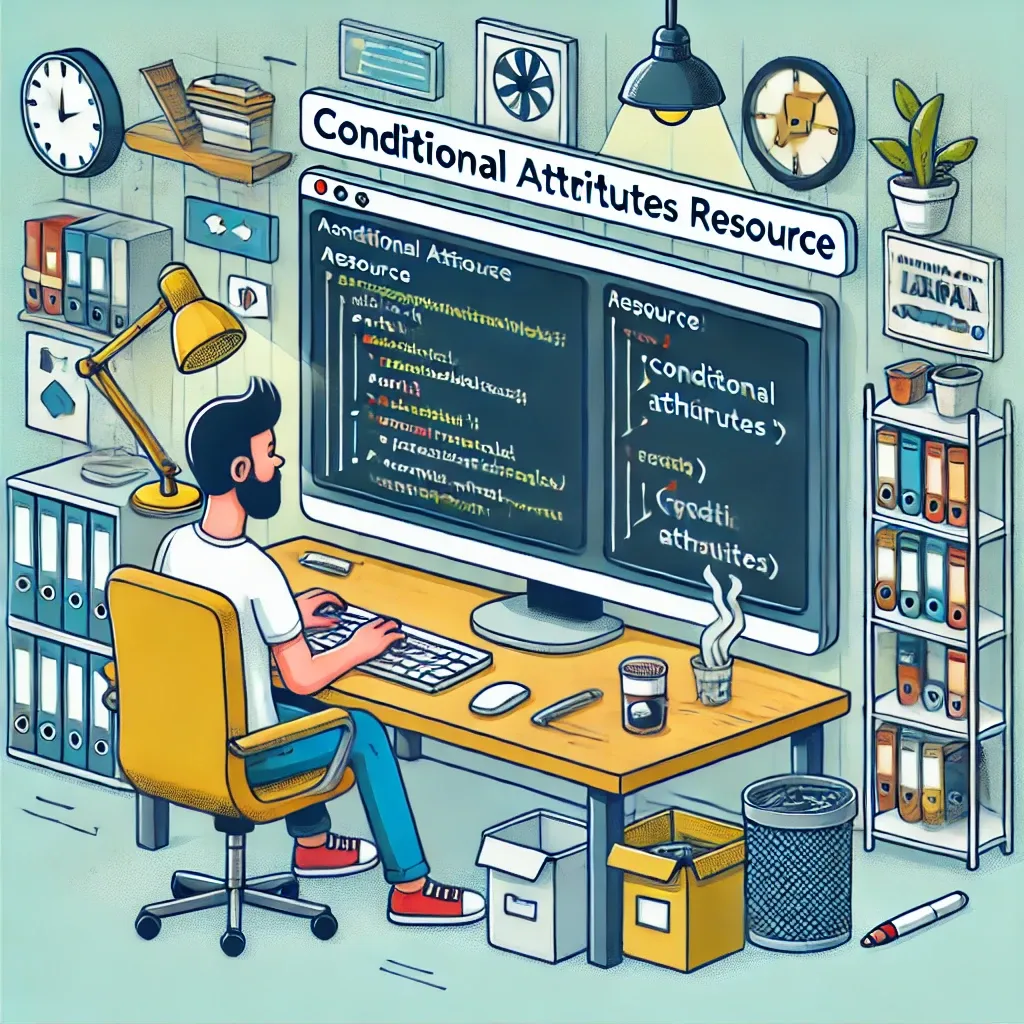
When building APIs with Laravel, you often need to include or exclude certain data based on specific conditions. Laravel's API Resources provide powerful methods to conditionally include attributes in your API responses. Let's explore how to use these features to create more dynamic and efficient API responses.
The when Method
The when
method allows you to include an attribute in a resource response only if a given condition is true:
use Illuminate\Http\Request;
use Illuminate\Http\Resources\Json\JsonResource;
class UserResource extends JsonResource
{
public function toArray(Request $request): array
{
return [
'id' => $this->id,
'name' => $this->name,
'email' => $this->email,
'secret' => $this->when($request->user()->isAdmin(), 'secret-value'),
'created_at' => $this->created_at,
];
}
}
In this example, the secret
key will only be included in the response if the authenticated user is an admin.
Using Closures with when
You can also use a closure as the second argument to when
, allowing for more complex logic:
'secret' => $this->when($request->user()->isAdmin(), function () {
return 'secret-value';
}),
This is particularly useful when the value you want to include requires some computation.
The whenHas Method
The whenHas
method is perfect for including an attribute only if it exists on the model:
'name' => $this->whenHas('name'),
This is especially useful when dealing with optional attributes.
The whenNotNull Method
Similarly, whenNotNull
includes an attribute only if it's not null:
'last_login' => $this->whenNotNull($this->last_login),
This helps in creating cleaner responses by omitting null values.
Practical Example
Let's consider a more comprehensive example with a PostResource
:
use Illuminate\Http\Request;
use Illuminate\Http\Resources\Json\JsonResource;
class PostResource extends JsonResource
{
public function toArray(Request $request): array
{
return [
'id' => $this->id,
'title' => $this->title,
'content' => $this->when(!$request->is('api/posts'), $this->content),
'excerpt' => $this->when($request->is('api/posts'), $this->content),
'author' => new UserResource($this->whenLoaded('author')),
'comments_count' => $this->when($request->include_counts, $this->comments_count),
'created_at' => $this->created_at,
'updated_at' => $this->updated_at,
'admin_notes' => $this->when($request->user()->isAdmin(), $this->admin_notes),
];
}
}
By leveraging these conditional methods in Laravel API Resources, you can create more efficient and context-aware API responses. This not only improves the performance of your API by reducing unnecessary data transfer but also enhances security by ensuring sensitive data is only included when appropriate.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!