Fine-Tuning Laravel Middleware Execution: Mastering Priority
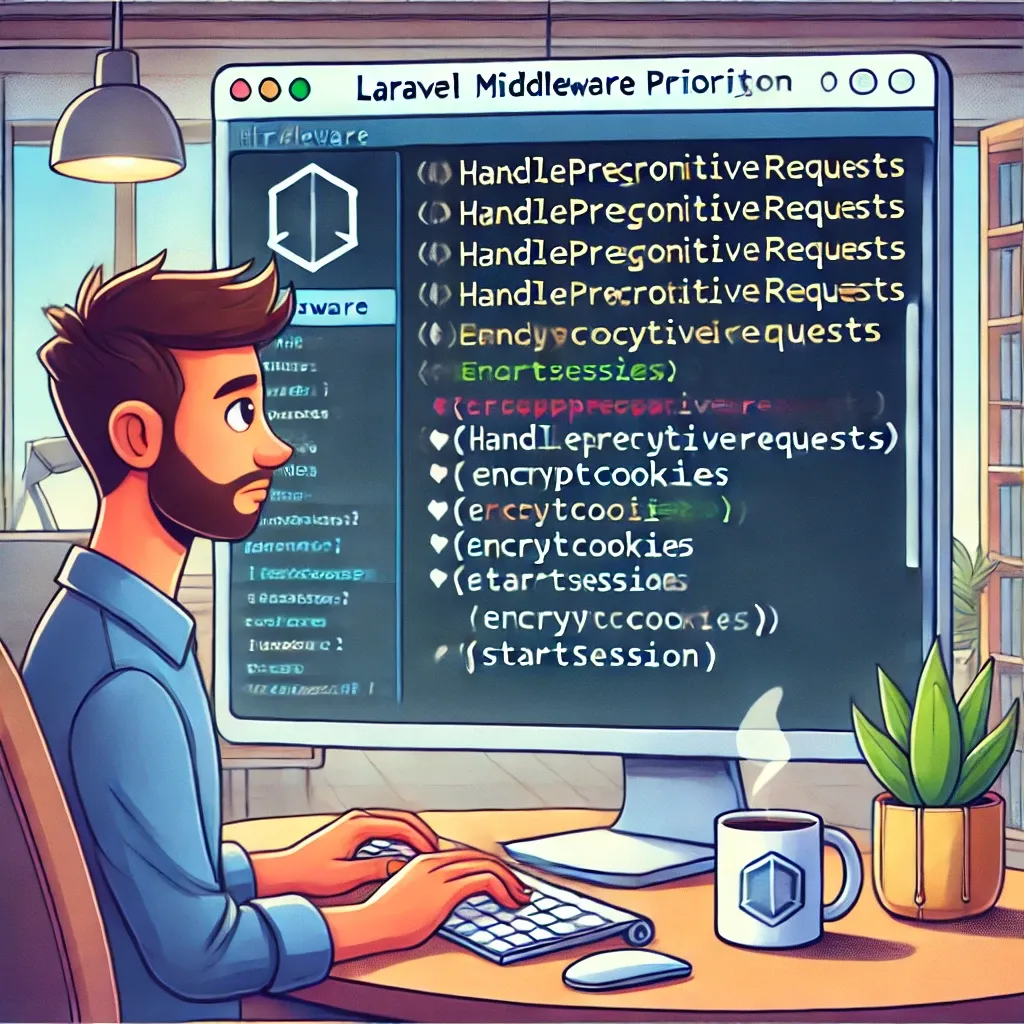
In the world of Laravel application development, middleware plays a crucial role in processing HTTP requests. While the order of middleware execution is typically determined by the order in which they're assigned to routes, there are scenarios where you need more granular control. This is where Laravel's middleware priority feature comes into play. Let's dive deep into how you can leverage this powerful tool to fine-tune your application's request handling pipeline.
Understanding Middleware Priority
Middleware priority allows you to specify a global execution order for your middleware, regardless of the order in which they're assigned to routes. This is particularly useful when you have middleware that must run before others, but you don't have control over their assignment order in various parts of your application.
Setting Middleware Priority
To set middleware priority, you'll need to use the priority
method in your application's bootstrap/app.php
file. Here's how you can do it:
->withMiddleware(function (Middleware $middleware) {
$middleware->priority([
\Illuminate\Foundation\Http\Middleware\HandlePrecognitiveRequests::class,
\Illuminate\Cookie\Middleware\EncryptCookies::class,
\Illuminate\Session\Middleware\StartSession::class,
\Illuminate\View\Middleware\ShareErrorsFromSession::class,
\Illuminate\Foundation\Http\Middleware\ValidateCsrfToken::class,
\Illuminate\Routing\Middleware\SubstituteBindings::class,
\Illuminate\Auth\Middleware\Authorize::class,
]);
})
In this example, we're setting a priority order for several core Laravel middleware. The middleware listed first will be executed first, regardless of how they're assigned to routes.
Real-Life Example
Let's consider a scenario where we're building a web application with some custom middleware for logging, authentication, and request throttling. We want to ensure that logging happens first, followed by authentication, and then throttling, regardless of how these middleware are assigned to routes.
Here's how we might set this up:
// In bootstrap/app.php
use App\Http\Middleware\CustomLogger;
use App\Http\Middleware\CustomAuthenticator;
use App\Http\Middleware\CustomThrottler;
->withMiddleware(function (Middleware $middleware) {
$middleware->priority([
CustomLogger::class,
CustomAuthenticator::class,
CustomThrottler::class,
// ... other middleware
]);
})
Now, let's see how this might affect our request handling:
// In a controller
public function index(Request $request)
{
// This action is protected by our custom middleware
return response()->json(['message' => 'Welcome to the dashboard']);
}
// In routes/web.php
Route::get('/dashboard', [DashboardController::class, 'index'])
->middleware(['custom.throttle', 'custom.auth', 'custom.log']);
Even though we've assigned the middleware in the order custom.throttle
, custom.auth
, custom.log
, they will actually execute in the order we specified in our priority list: logging, then authentication, then throttling.
Here's what the process might look like:
// Request comes in to /dashboard
// 1. CustomLogger middleware runs
// (Logs the incoming request)
// 2. CustomAuthenticator middleware runs
// If not authenticated:
{
"error": "Unauthenticated",
"code": 401
}
// 3. CustomThrottler middleware runs
// If too many requests:
{
"error": "Too Many Requests",
"code": 429
}
// If all middleware pass:
{
"message": "Welcome to the dashboard"
}
This approach ensures that:
- Every request is logged, even if it fails authentication or is throttled.
- Authentication always happens before throttling, preventing unnecessary load on your throttling system from unauthenticated requests.
- The order of middleware execution is consistent across your entire application, regardless of how routes are defined.
If you found this guide helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel tips and tricks!