Fine-Tuning Input Handling in Laravel: Customizing TrimStrings and ConvertEmptyStringsToNull Middleware
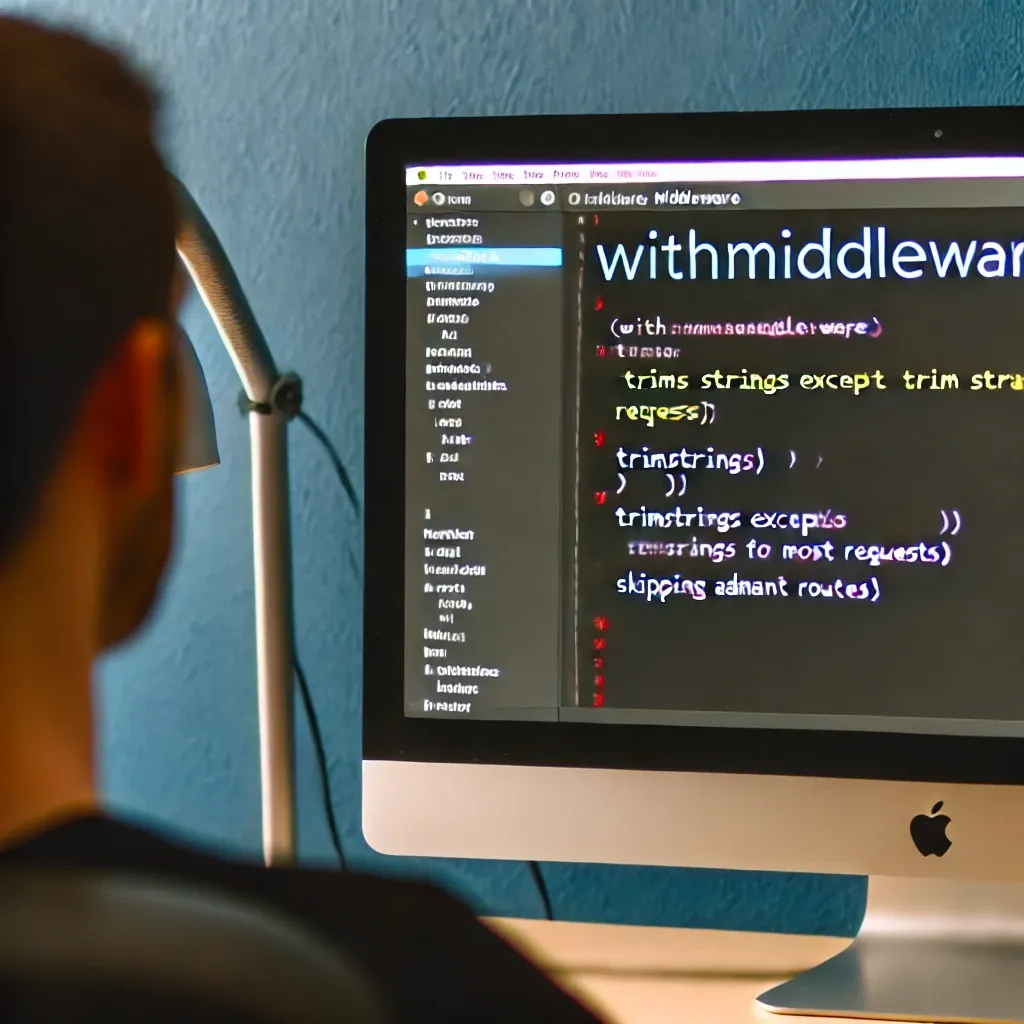
Laravel, out of the box, applies two global middleware to normalize input data: TrimStrings
and ConvertEmptyStringsToNull
. While these are generally helpful, there might be scenarios where you need more control over this behavior. Laravel provides flexible ways to customize or disable these middleware entirely or for specific routes. Let's explore how you can fine-tune input handling in your Laravel applications.
Understanding the Default Behavior
By default, Laravel applies two middleware to all incoming requests:
TrimStrings
: Trims whitespace from the beginning and end of string inputs.ConvertEmptyStringsToNull
: Converts empty strings tonull
values.
Disabling the Middleware Globally
If you want to disable these middleware for all requests, you can remove them from your application's middleware stack in the bootstrap/app.php
file:
use Illuminate\Foundation\Http\Middleware\ConvertEmptyStringsToNull;
use Illuminate\Foundation\Http\Middleware\TrimStrings;
->withMiddleware(function (Middleware $middleware) {
$middleware->remove([
ConvertEmptyStringsToNull::class,
TrimStrings::class,
]);
})
Customizing Middleware Behavior
For more granular control, you can customize when these middleware are applied using closures. This is done in the bootstrap/app.php
file:
->withMiddleware(function (Middleware $middleware) {
$middleware->convertEmptyStringsToNull(except: [
fn (Request $request) => $request->is('admin/*'),
]);
$middleware->trimStrings(except: [
fn (Request $request) => $request->is('admin/*'),
]);
})
In this example, we're disabling both middleware for all routes that start with 'admin/'.
Real-Life Example
Let's consider a scenario where you have a text editor in your admin panel where you want to preserve whitespace and empty strings exactly as entered by the user.
Here's how you might implement this:
<?php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
class AdminPostController extends Controller
{
public function store(Request $request)
{
$validated = $request->validate([
'title' => 'required|string|max:255',
'content' => 'required|string',
]);
$post = Post::create($validated);
return response()->json($post, 201);
}
}
With the middleware customization we set up earlier, when this controller receives a request to the /admin/posts
endpoint, the content
field will preserve any leading or trailing whitespace, and empty strings will remain as empty strings rather than being converted to null
.
Here's what the input and output might look like:
// POST /admin/posts
// Input
{
"title": " My Formatted Post ",
"content": " This content has significant whitespace.\n\nIncluding empty lines.\n "
}
// Output
{
"id": 1,
"title": " My Formatted Post ",
"content": " This content has significant whitespace.\n\nIncluding empty lines.\n ",
"created_at": "2023-06-15T19:30:00.000000Z",
"updated_at": "2023-06-15T19:30:00.000000Z"
}
Notice how the whitespace in both the title and content is preserved, which wouldn't be the case with the default middleware behavior.
By mastering the customization of Laravel's input handling middleware, you can create more flexible and precise applications that handle user input exactly as your use case requires. Whether you're building complex admin interfaces, working with formatted text, or just need more control over your input data, these techniques give you the power to fine-tune Laravel's behavior to your exact needs.
If you found this tip helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel gems!