Fine-Tuning Error Handling: Ignoring Specific Exceptions in Laravel
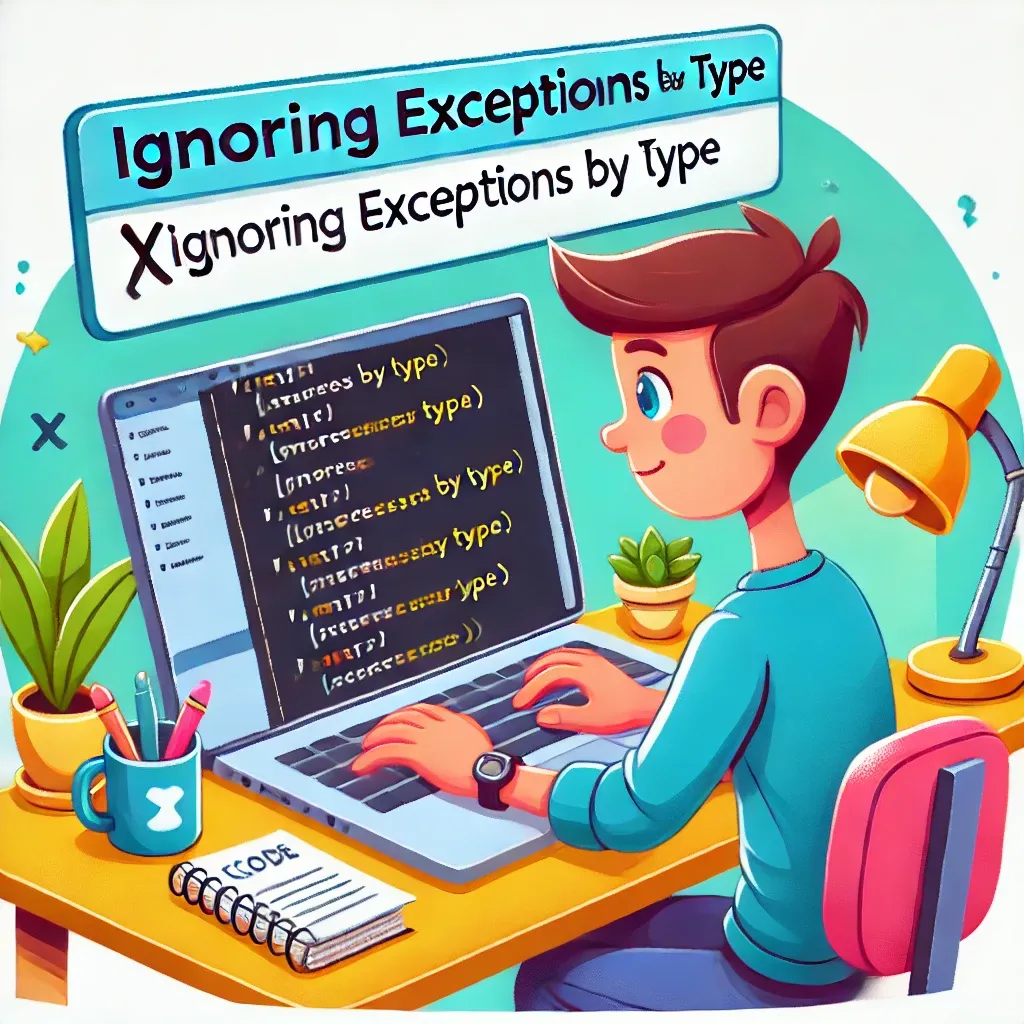
In the world of Laravel development, robust error handling is crucial for maintaining a stable and user-friendly application. However, there are scenarios where you might want to ignore certain types of exceptions. Laravel provides an elegant way to accomplish this, allowing developers to fine-tune their application's error reporting. Let's dive into how you can leverage this feature in your Laravel projects.
Understanding Exception Ignoring
Laravel allows you to specify certain exception types that should not be reported. This is particularly useful for exceptions that you expect to occur and don't want cluttering your logs or error reporting services.
Configuring Ignored Exceptions
To ignore specific exception types, you'll need to modify your application's exception handling configuration. This is typically done in the bootstrap/app.php
file.
Here's how you can ignore exceptions:
use App\Exceptions\InvalidOrderException;
$app->withExceptions(function (Exceptions $exceptions) {
$exceptions->dontReport([
InvalidOrderException::class,
]);
});
In this example, any InvalidOrderException
thrown in your application will not be reported.
Using the ShouldntReport Interface
An alternative approach is to mark exception classes with the Illuminate\Contracts\Debug\ShouldntReport
interface:
use Exception;
use Illuminate\Contracts\Debug\ShouldntReport;
class CustomException extends Exception implements ShouldntReport
{
// Exception logic
}
Exceptions implementing this interface will automatically be ignored by Laravel's exception handler.
Real-World Scenario
Let's consider a practical example. Imagine you have an e-commerce application where you want to handle invalid order submissions without reporting them as errors:
use Exception;
use Illuminate\Contracts\Debug\ShouldntReport;
class InvalidOrderException extends Exception implements ShouldntReport
{
public function render($request)
{
return response()->json(['message' => 'Invalid order submission'], 422);
}
}
class OrderController extends Controller
{
public function store(Request $request)
{
try {
// Order processing logic
if (!$this->isValidOrder($request->all())) {
throw new InvalidOrderException('Invalid order data');
}
// Process the order...
} catch (InvalidOrderException $e) {
// This exception won't be reported, but will be rendered
return $e->render($request);
}
}
}
In this scenario, InvalidOrderException
won't clutter your logs, but you can still handle it gracefully in your application flow.
Stopping Laravel from Ignoring Built-in Exceptions
Laravel internally ignores some types of exceptions, such as 404 HTTP errors. If you want Laravel to stop ignoring these, you can use the stopIgnoring
method:
use Symfony\Component\HttpKernel\Exception\HttpException;
$app->withExceptions(function (Exceptions $exceptions) {
$exceptions->stopIgnoring(HttpException::class);
});
By leveraging Laravel's exception ignoring features, you can create a more focused and efficient error handling system. This not only helps in keeping your logs clean and relevant but also allows you to handle expected exceptions in a more graceful manner. Remember, the goal is not to hide errors, but to manage them effectively, ensuring that your attention is directed to the issues that truly matter in your application.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!