Fine-Tuning Access Control with Laravel's Policy-Based Authorization
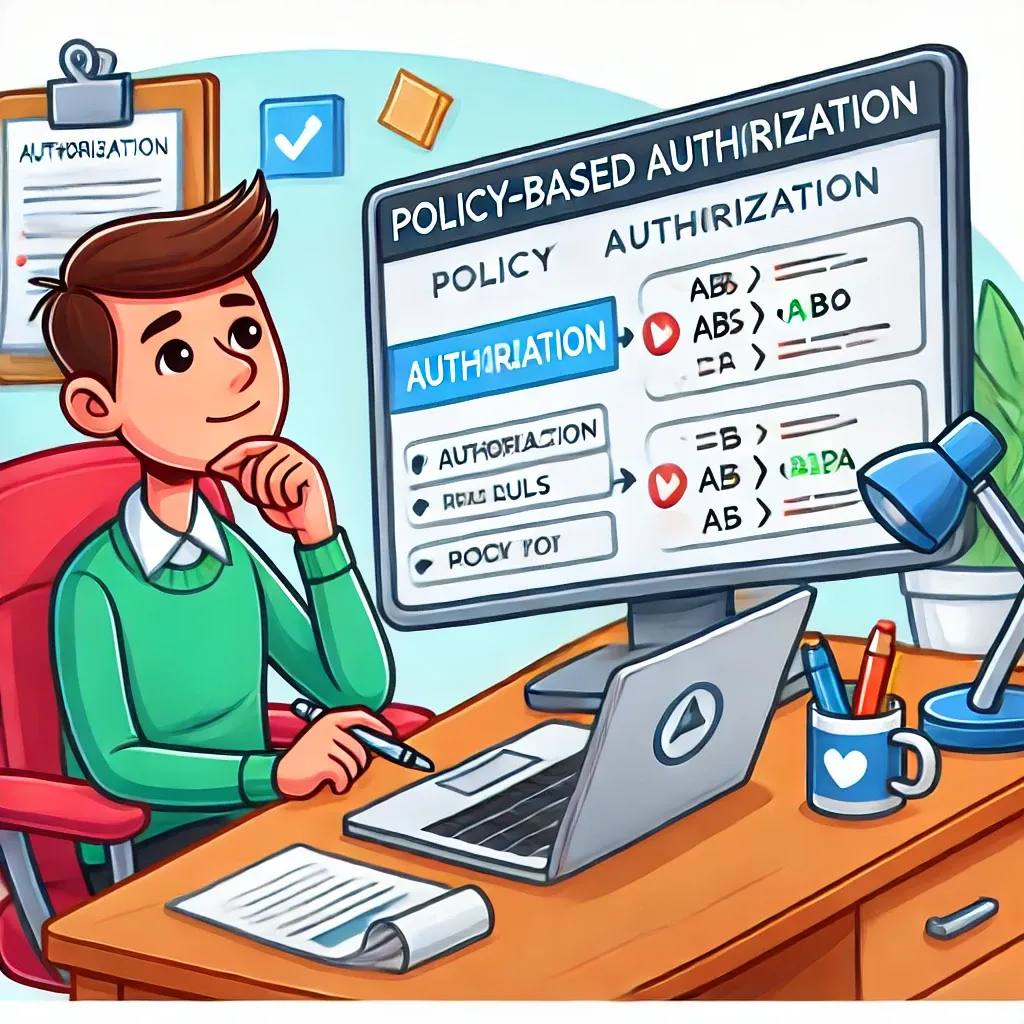
Laravel's policy-based authorization provides a powerful and expressive way to handle complex permissions in your application. This feature allows you to organize your authorization logic around specific models or resources, making your code more maintainable and easier to understand.
Creating a Policy
To create a policy, use the Artisan command:
php artisan make:policy PostPolicy --model=Post
This generates a policy class with stub methods for various actions:
namespace App\Policies;
use App\Models\Post;
use App\Models\User;
class PostPolicy
{
public function viewAny(User $user)
{
//
}
public function view(User $user, Post $post)
{
//
}
public function create(User $user)
{
//
}
public function update(User $user, Post $post)
{
return $user->id === $post->user_id;
}
// Other methods...
}
Registering Policies
Register your policies in the AuthServiceProvider
:
protected $policies = [
Post::class => PostPolicy::class,
];
Using Policies in Controllers
You can use the authorize
method in your controllers:
public function update(Request $request, Post $post)
{
$this->authorize('update', $post);
// Update logic...
}
Or use the @can
directive in Blade templates:
@can('update', $post)
<button>Edit Post</button>
@endcan
Policy Filters
For app-wide rules, use policy filters in the AuthServiceProvider
:
public function boot()
{
Gate::before(function ($user, $ability) {
if ($user->isAdministrator()) {
return true;
}
});
}
Resource Controllers
When using resource controllers, you can authorize actions in the constructor:
public function __construct()
{
$this->authorizeResource(Post::class, 'post');
}
Custom Policy Methods
You can define custom methods in your policies:
public function publish(User $user, Post $post)
{
return $user->role === 'editor' && !$post->is_published;
}
And use them like this:
if ($user->can('publish', $post)) {
// Publish the post...
}
Policy Responses
Policies can return more than just booleans:
public function view(User $user, Post $post)
{
if ($post->is_draft && $user->id !== $post->user_id) {
return Response::deny('You cannot view draft posts.', 403);
}
return Response::allow();
}
Guest Users
For unauthenticated users, you can use the ?
operator:
public function view(?User $user, Post $post)
{
return $post->is_published;
}
Testing Policies
You can easily test your policies:
use Illuminate\Support\Facades\Gate;
public function test_user_can_update_own_post()
{
$user = User::factory()->create();
$post = Post::factory()->create(['user_id' => $user->id]);
$this->assertTrue(Gate::forUser($user)->allows('update', $post));
}
Laravel's policy-based authorization provides a clean and organized way to implement complex permission systems. By centralizing your authorization logic in policies, you can create more maintainable and secure applications, easily adapting to changing business rules and user roles.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!