Fine-Tune Number Formatting with Laravel's Enhanced Number::spell Method
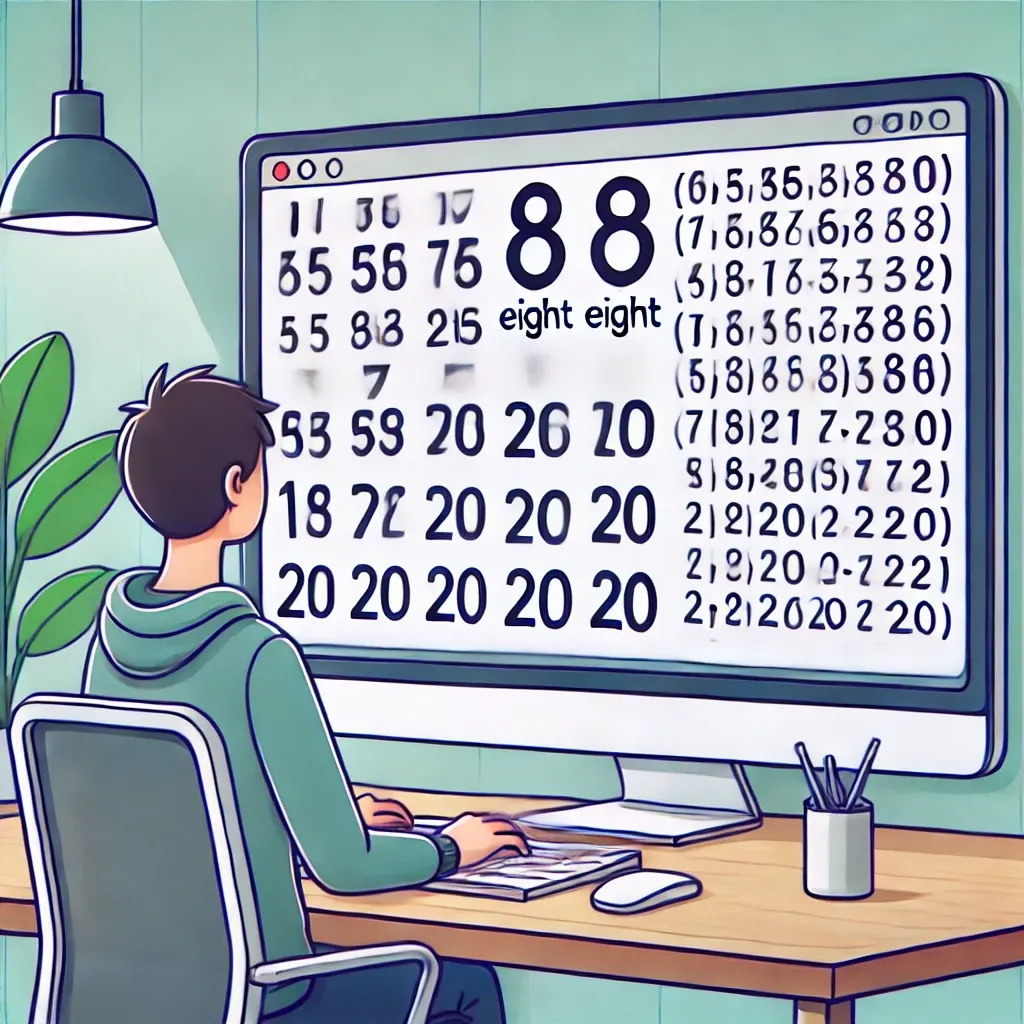
Need to spell out only certain numbers in your Laravel application? The enhanced Number::spell method now accepts 'until' and 'after' parameters to define exactly when numbers should be written as words.
When presenting numbers in user interfaces, documentation, or reports, you might want to follow typography conventions where small numbers are spelled out while larger values remain as digits. Laravel's Number helper has been enhanced to make this pattern easy to implement with new threshold parameters.
Let's see how these new parameters work:
use Illuminate\Support\Number;
// Use 'until' parameter to spell out numbers below the threshold
Number::spell(8, until: 10); // "eight"
Number::spell(10, until: 10); // "10"
Number::spell(20, until: 10); // "20"
// Use 'after' parameter to spell out numbers above the threshold
Number::spell(9, after: 10); // "9"
Number::spell(11, after: 10); // "eleven"
Number::spell(12, after: 10); // "twelve"
Real-World Example
This feature is particularly useful when implementing typography best practices or creating readable content. Here's a practical example for generating report descriptions:
class SalesReportController extends Controller
{
public function summary()
{
$data = [
'total_customers' => 8,
'new_customers' => 12,
'products_sold' => 843,
'top_product_sales' => 137
];
return view('reports.summary', [
'data' => $data,
'description' => $this->generateDescription($data)
]);
}
private function generateDescription($data)
{
// Spell out small numbers, use digits for larger ones
return "We served " . Number::spell($data['total_customers'], until: 10) .
" existing customers and welcomed " . Number::spell($data['new_customers'], until: 10) .
" new ones this month. Overall, we sold " . Number::spell($data['products_sold']) .
" products, with our top performer accounting for " . Number::spell($data['top_product_sales']) .
" units.";
}
}
This would generate: "We served eight existing customers and welcomed 12 new ones this month. Overall, we sold 843 products, with our top performer accounting for 137 units."
By leveraging these new threshold parameters, you can implement sophisticated number formatting rules with minimal code, leading to cleaner views and more readable content.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!