Finding Duplicates in Laravel Collections
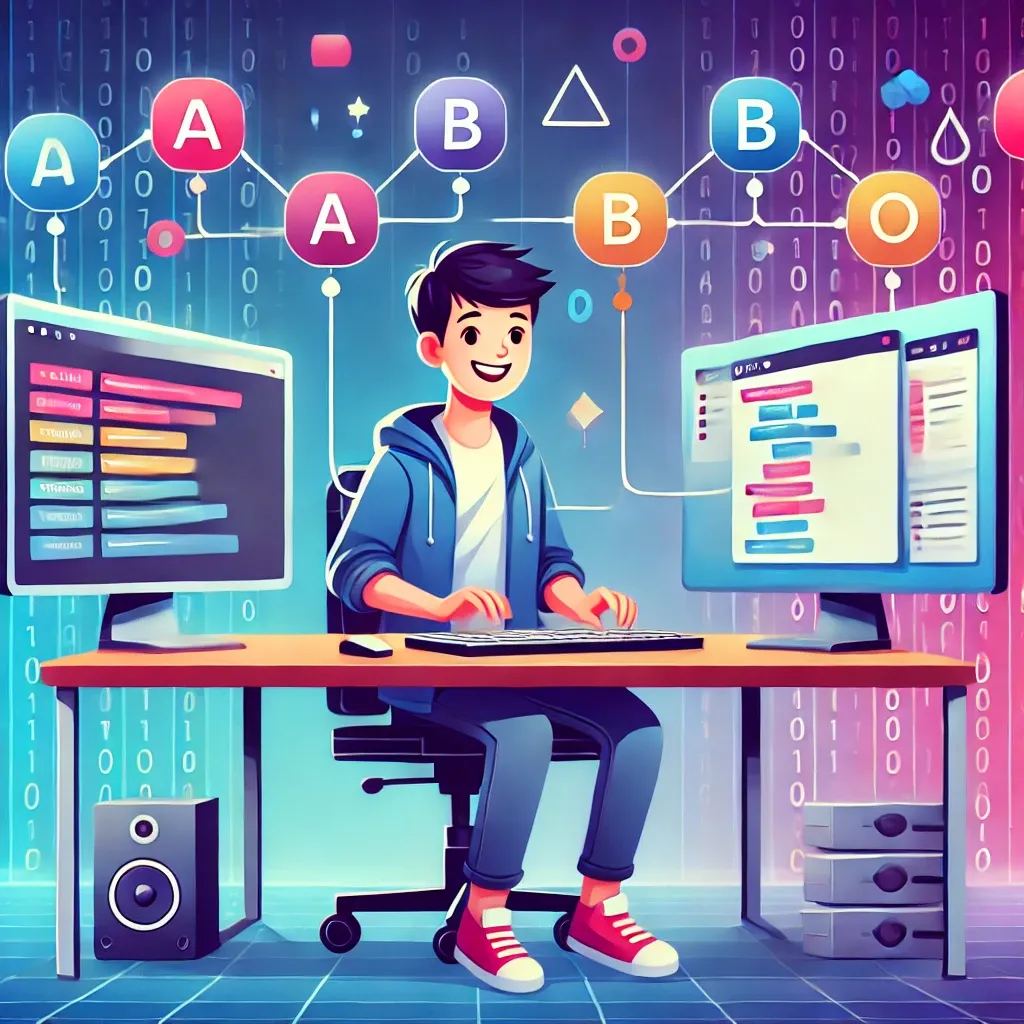
Need to track down duplicates in your collection? Laravel's duplicates methods provide a simple way to find repeated values, whether they're simple types or nested attributes.
Basic Usage
Find duplicates with regular and strict checking:
// Regular duplicate checking
$collection = collect(['a', 'b', 'A', 'a', 'B', 'b']);
$duplicates = $collection->duplicates();
// Result: [3 => 'a', 5 => 'b'] // Case-insensitive
// Strict duplicate checking
$strictDuplicates = $collection->duplicatesStrict();
// Result: [3 => 'a', 5 => 'b'] // Case-sensitive
Working with Arrays and Objects
Check for duplicates in specific attributes:
$employees = collect([
['email' => 'abigail@example.com', 'position' => 'Developer'],
['email' => 'james@example.com', 'position' => 'Designer'],
['email' => 'victoria@example.com', 'position' => 'Developer']
]);
$duplicatePositions = $employees->duplicates('position');
// Result: [2 => 'Developer']
Real-World Example
Here's how you might use it in a data validation service:
class DataValidator
{
public function validateUniqueEmails(Collection $users)
{
// Regular duplicate check for emails
$duplicates = $users->duplicates('email');
if ($duplicates->isNotEmpty()) {
throw new ValidationException(
"Duplicate emails found: " .
$duplicates->implode(', ')
);
}
}
public function findDuplicateTags(Collection $tags)
{
return [
'case_insensitive' => $tags->duplicates(),
'case_sensitive' => $tags->duplicatesStrict()
];
}
public function checkUniqueTags(Collection $posts)
{
$tags = collect([
'php', 'PHP', 'php', 'laravel', 'Laravel', 'LARAVEL'
]);
return [
'basic_duplicates' => $tags->duplicates(),
// Result: [2 => 'php', 4 => 'Laravel']
'case_sensitive_duplicates' => $tags->duplicatesStrict(),
// Result: [2 => 'php']
];
}
}
The duplicates methods make it easy to identify repeated values in your collections, helping ensure data integrity and validation.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!