Extract Single Matching Items Confidently with Laravel's Arr::sole() Method
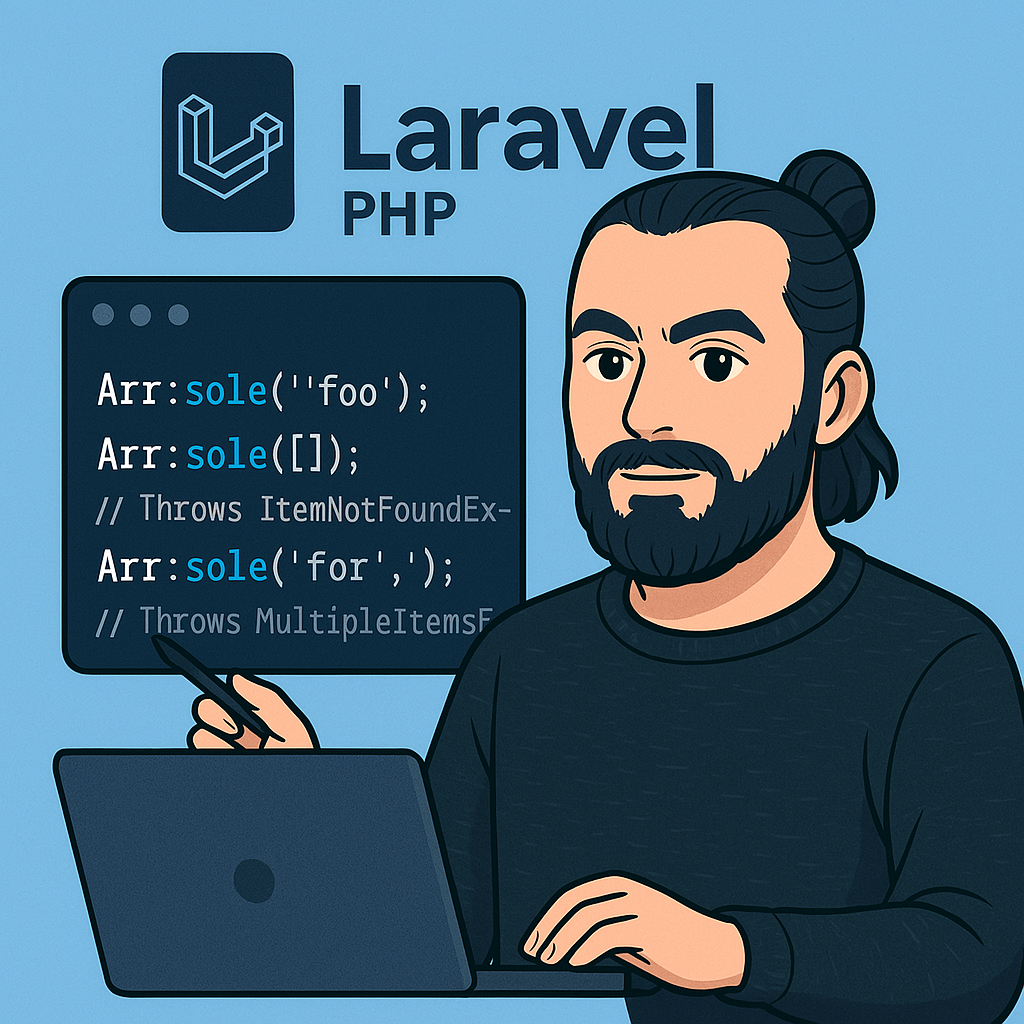
Need to confidently extract a single matching item from an array in Laravel? The new Arr::sole() method brings the power of Eloquent's 'sole' functionality to plain PHP arrays.
When working with arrays, you sometimes need to retrieve an item with confidence that it's the only one matching your criteria. Traditionally, this would require multiple checks - first to find matching items, then to verify there's exactly one match. Laravel's new Arr::sole() method streamlines this process by handling both concerns in a single, expressive operation.
Let's see how it works:
use Illuminate\Support\Arr;
// Returns "foo" if it's the only item in the array
Arr::sole(['foo']); // "foo"
// Throws an exception if the array is empty
Arr::sole([]); // Throws ItemNotFoundException
// Throws an exception if there are multiple items
Arr::sole(['foo', 'bar']); // Throws MultipleItemsFoundException
Real-World Example
The Arr::sole() method becomes even more powerful when combined with a callback function to filter items based on specific criteria:
use Illuminate\Support\Arr;
// User data array
$users = [
['id' => 1, 'name' => 'Taylor', 'role' => 'admin'],
['id' => 2, 'name' => 'Abigail', 'role' => 'user'],
['id' => 3, 'name' => 'James', 'role' => 'user'],
];
// Get the sole admin user
$adminUser = Arr::sole($users, fn ($user) => $user['role'] === 'admin');
// Returns ['id' => 1, 'name' => 'Taylor', 'role' => 'admin']
// This would throw MultipleItemsFoundException if there were multiple admins
// This would throw ItemNotFoundException if there were no admins
The method will respond differently based on what it finds:
// Throws ItemNotFoundException if no items match the callback
Arr::sole(['foo', 'bar'], fn (string $value) => $value === 'baz');
// Throws MultipleItemsFoundException if multiple items match
Arr::sole(['baz', 'foo', 'baz'], fn (string $value) => $value === 'baz');
By leveraging Arr::sole(), you can write more expressive, intention-revealing code that clearly communicates your expectation of finding exactly one matching item.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!