Extending Laravel's URI Class with Custom Macros
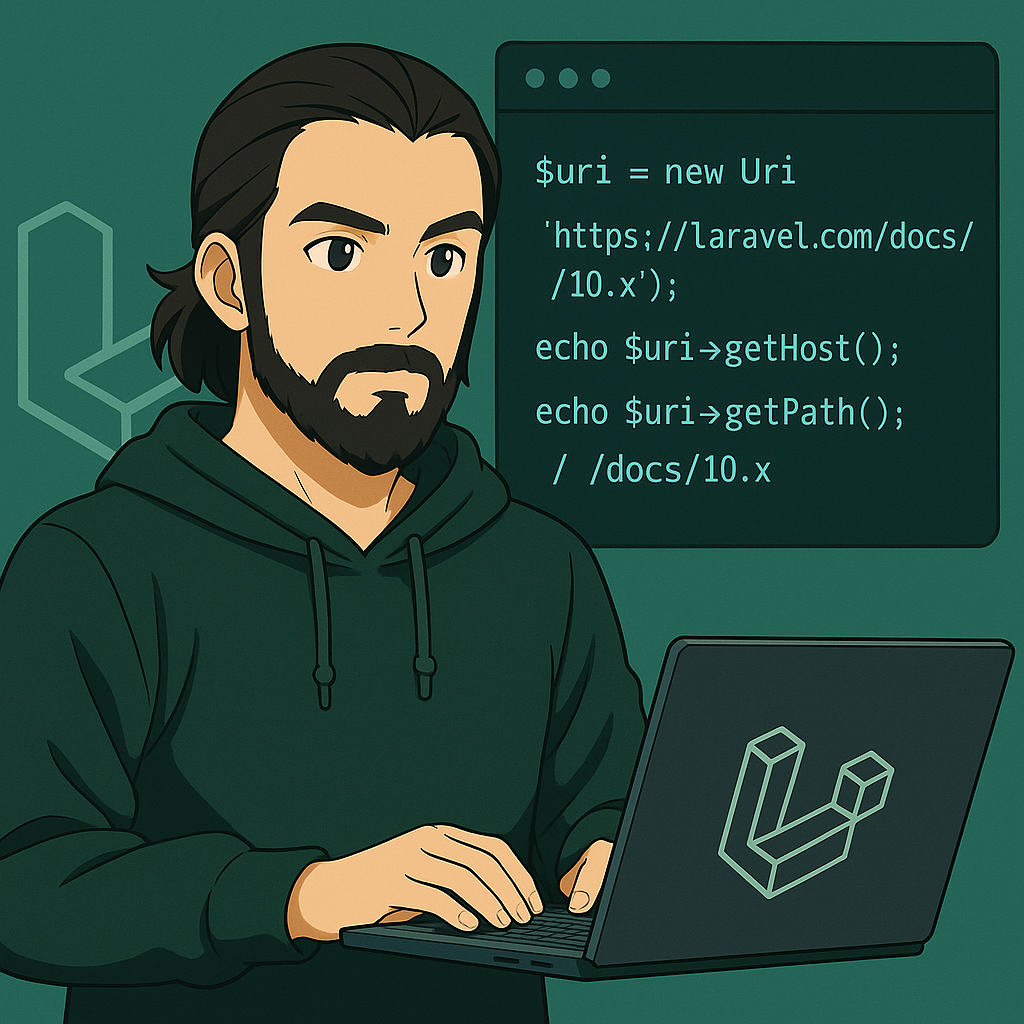
Laravel has always excelled at making common web development tasks elegant and expressive. With the addition of the Macroable
trait to the URI class, you can now create your own custom URL manipulation methods that feel like they were built into the framework from day one.
Understanding the URI Class
Laravel's Illuminate\Support\Uri
class provides a clean, object-oriented way to work with URLs. It allows you to parse, manipulate, and build URLs without resorting to PHP's native URL functions or string manipulation.
Basic usage of the URI class looks like this:
use Illuminate\Support\Uri;
$uri = new Uri('https://laravel.com/docs/10.x');
echo $uri->getHost(); // laravel.com
echo $uri->getPath(); // /docs/10.x
While this API is already quite convenient, Laravel has now made it even more powerful and customizable.
The Power of Macroable
The Macroable
trait is a pattern used throughout Laravel that allows you to add custom methods to existing classes at runtime. It's a clean way to extend functionality without modifying source code or creating complex inheritance structures.
With the URI class now implementing this trait, you can define your own URL manipulation methods that fit your application's specific needs.
Creating Your First URI Macro
Let's look at how you can create a simple URI macro:
use Illuminate\Support\Uri;
Uri::macro('docs', fn () => $this->withPath('docs'));
$uri = new Uri('https://laravel.com/');
$docsUri = $uri->docs(); // https://laravel.com/docs
This macro adds a docs()
method to all URI instances, which conveniently changes the path to "docs". The beautiful thing is that it reads like a native method, making your code clean and intuitive.
Real-World Example
Let's explore a more practical example. Imagine you're building an application that frequently needs to generate different types of URLs for your API:
use Illuminate\Support\Uri;
// Register macros in a service provider
class AppServiceProvider extends ServiceProvider
{
public function boot()
{
// Add version to API URL
Uri::macro('v1', function () {
return $this->withPath('api/v1/' . trim($this->getPath(), '/'));
});
// Convert to documentation URL
Uri::macro('apiDocs', function () {
$path = trim($this->getPath(), '/');
return $this->withHost('docs.' . $this->getHost())
->withPath($path);
});
// Create webhook URL
Uri::macro('webhook', function ($event) {
return $this->withPath('webhooks/' . $event);
});
}
}
// Usage in your application
$baseUri = new Uri('https://example.com/users');
// https://example.com/api/v1/users
$apiUri = $baseUri->v1();
// https://docs.example.com/users
$docsUri = $baseUri->apiDocs();
// https://example.com/webhooks/user.created
$webhookUri = $baseUri->webhook('user.created');
This approach allows you to create a domain-specific language for URL manipulation that perfectly fits your application's needs.
Stay up to date with more Laravel tips and tricks by following me: