Excluding URIs from CSRF Protection in Laravel
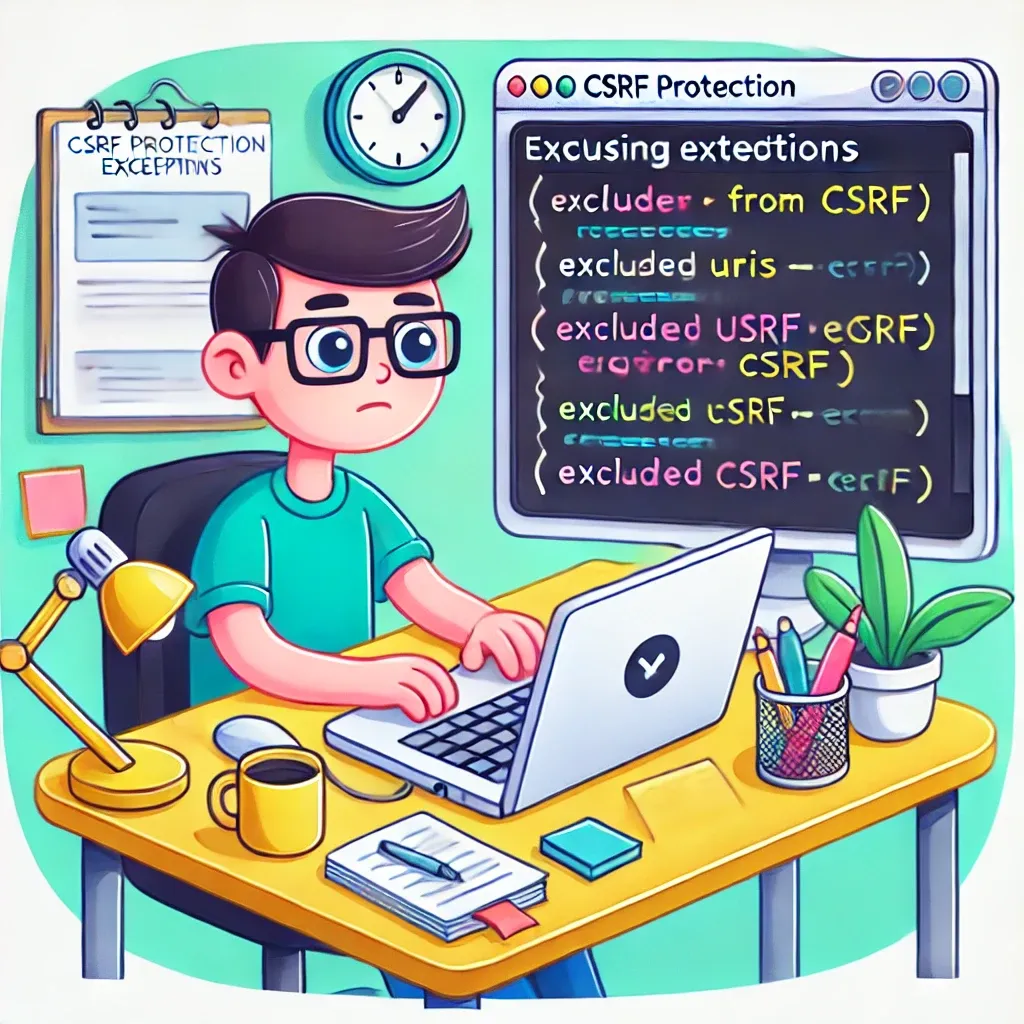
Laravel's built-in CSRF (Cross-Site Request Forgery) protection is a crucial security feature that helps safeguard your web applications. However, there are scenarios where you might need to exclude certain URIs from this protection, such as when handling webhooks or third-party callbacks. Let's explore how to effectively manage CSRF exceptions in Laravel while maintaining robust security.
Understanding CSRF Protection
Before diving into exclusions, it's important to understand why CSRF protection is vital:
- It prevents unauthorized commands from being transmitted from a user that the web application trusts.
- Laravel automatically generates CSRF tokens for each active user session.
- These tokens are validated on subsequent requests to protect against cross-site request forgeries.
The VerifyCsrfToken Middleware
Laravel uses the VerifyCsrfToken
middleware to handle CSRF protection. This middleware is where we'll define our exceptions.
Excluding URIs from CSRF Protection
To exclude specific URIs, you'll need to modify the $except
property in the VerifyCsrfToken
middleware:
<?php
namespace App\Http\Middleware;
use Illuminate\Foundation\Http\Middleware\VerifyCsrfToken as Middleware;
class VerifyCsrfToken extends Middleware
{
/**
* The URIs that should be excluded from CSRF verification.
*
* @var array
*/
protected $except = [
'stripe/*',
'webhook/*',
];
}
In this example, any URI that starts with stripe/
or webhook/
will be excluded from CSRF protection.
Real-World Example: Handling a Stripe Webhook
Let's consider a practical example of handling a Stripe webhook:
// In App\Http\Middleware\VerifyCsrfToken.php
protected $except = [
'stripe/webhook',
];
// In routes/web.php
Route::post('stripe/webhook', 'StripeWebhookController@handleWebhook');
// In App\Http\Controllers\StripeWebhookController.php
public function handleWebhook(Request $request)
{
// Verify the webhook signature
$payload = $request->getContent();
$sig_header = $request->header('Stripe-Signature');
$event = null;
try {
$event = \Stripe\Webhook::constructEvent(
$payload, $sig_header, config('services.stripe.webhook_secret')
);
} catch(\UnexpectedValueException $e) {
return response()->json(['error' => 'Invalid payload'], 400);
} catch(\Stripe\Exception\SignatureVerificationException $e) {
return response()->json(['error' => 'Invalid signature'], 400);
}
// Handle the event
switch ($event->type) {
case 'payment_intent.succeeded':
$paymentIntent = $event->data->object;
handleSuccessfulPayment($paymentIntent);
break;
// ... handle other event types
default:
return response()->json(['error' => 'Unexpected event type'], 400);
}
return response()->json(['status' => 'success']);
}
In this example, we've excluded the Stripe webhook URI from CSRF protection but implemented Stripe's signature verification as an alternative security measure.
Testing CSRF Exclusions
When testing routes that are excluded from CSRF protection, you don't need to include the CSRF token:
public function testStripeWebhook()
{
$payload = [
'type' => 'payment_intent.succeeded',
'data' => [
'object' => [
'id' => 'pi_123456',
'amount' => 1000,
'currency' => 'usd',
],
],
];
$response = $this->postJson('/stripe/webhook', $payload, [
'Stripe-Signature' => 'test_signature',
]);
$response->assertStatus(200);
// Additional assertions...
}
Conclusion
Excluding URIs from CSRF protection in Laravel is a powerful feature that allows for flexibility in handling specific scenarios like webhooks. However, it's crucial to use this feature judiciously and implement alternative security measures where necessary. By following best practices and understanding the implications of CSRF exclusions, you can maintain a secure and flexible Laravel application.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!