Enhancing Your Views with Custom Blade Directives in Laravel
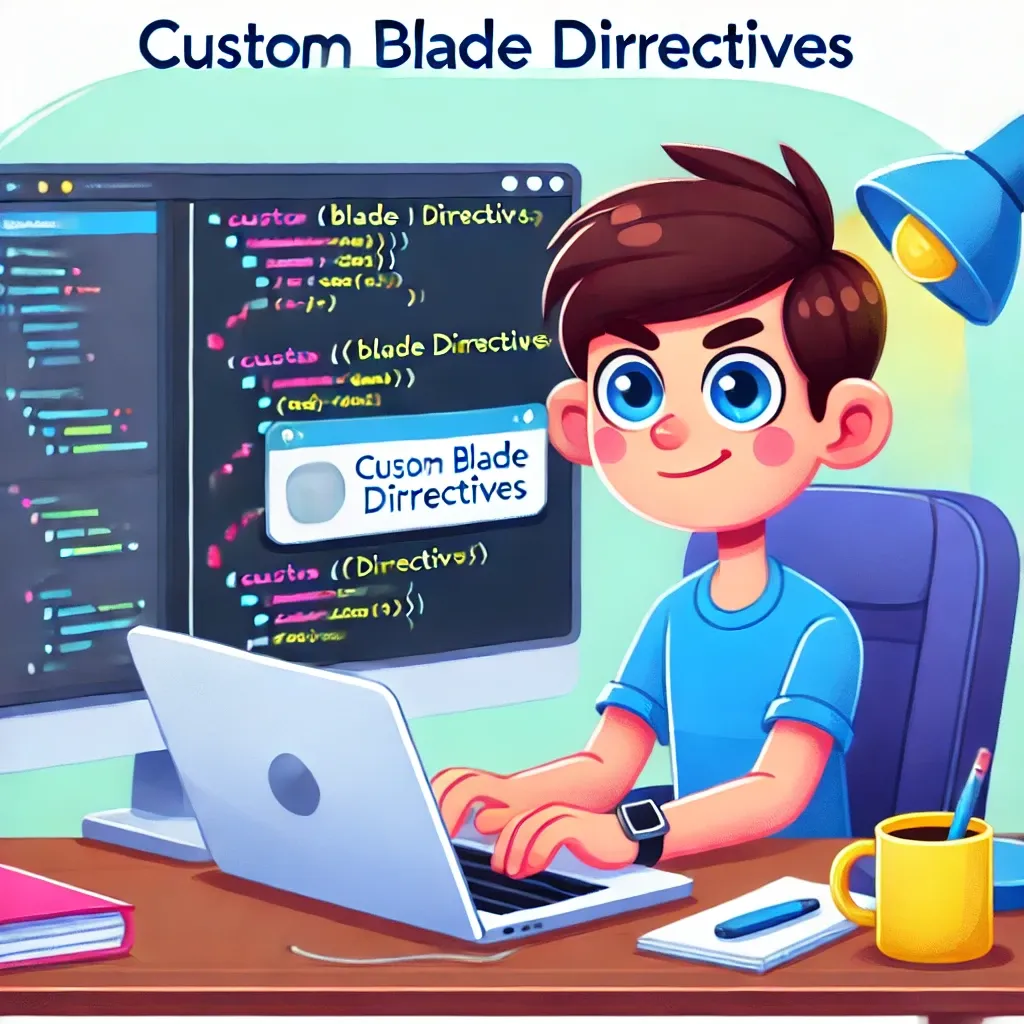
Blade, Laravel's powerful templating engine, offers a clean and expressive syntax for your views. While it comes with many built-in directives, you can extend its functionality by creating custom directives. Let's explore how to implement and use custom Blade directives for reusable view logic.
Creating a Custom Blade Directive
Custom Blade directives are defined in a service provider. You can use the AppServiceProvider or create a dedicated provider for your Blade extensions.
In your service provider's boot
method:
use Illuminate\Support\Facades\Blade;
public function boot()
{
Blade::directive('price', function ($expression) {
return "<?php echo '€' . number_format($expression, 2); ?>";
});
}
This creates a @price
directive that formats a number as a price with a Euro symbol and two decimal places.
Using the Custom Directive
In your Blade templates, you can now use the new directive:
<p>Product price: @price($product->price)</p>
If $product->price
is 29.99, this will output: "Product price: €29.99"
More Complex Directives
You can create more complex directives that accept multiple parameters:
Blade::directive('currency', function ($expression) {
list($amount, $currency) = explode(',', $expression);
return "<?php echo currency($amount, $currency); ?>";
});
Usage in a template:
<p>Price in USD: @currency($product->price, 'USD')</p>
Directives with Blocks
You can also create directives that work with blocks of content:
Blade::directive('ifadmin', function () {
return "<?php if(auth()->check() && auth()->user()->isAdmin()): ?>";
});
Blade::directive('endifadmin', function () {
return "<?php endif; ?>";
});
Usage in a template:
@ifadmin
<a href="/admin">Admin Panel</a>
@endifadmin
Reusable UI Components
Custom directives are great for creating reusable UI components:
Blade::directive('button', function ($expression) {
list($text, $type) = explode(',', $expression);
$type = trim($type, "' ");
return "<?php echo '<button class=\"btn btn-$type\">' . $text . '</button>'; ?>";
});
Usage:
@button('Submit', 'primary')
@button('Cancel', 'secondary')
Performance Considerations
Custom Blade directives are compiled and cached, so they don't add significant overhead to your application. However, for very complex logic, consider using view composers or dedicated classes instead.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!