Enhancing User Interactions in Laravel Livewire with wire:confirm
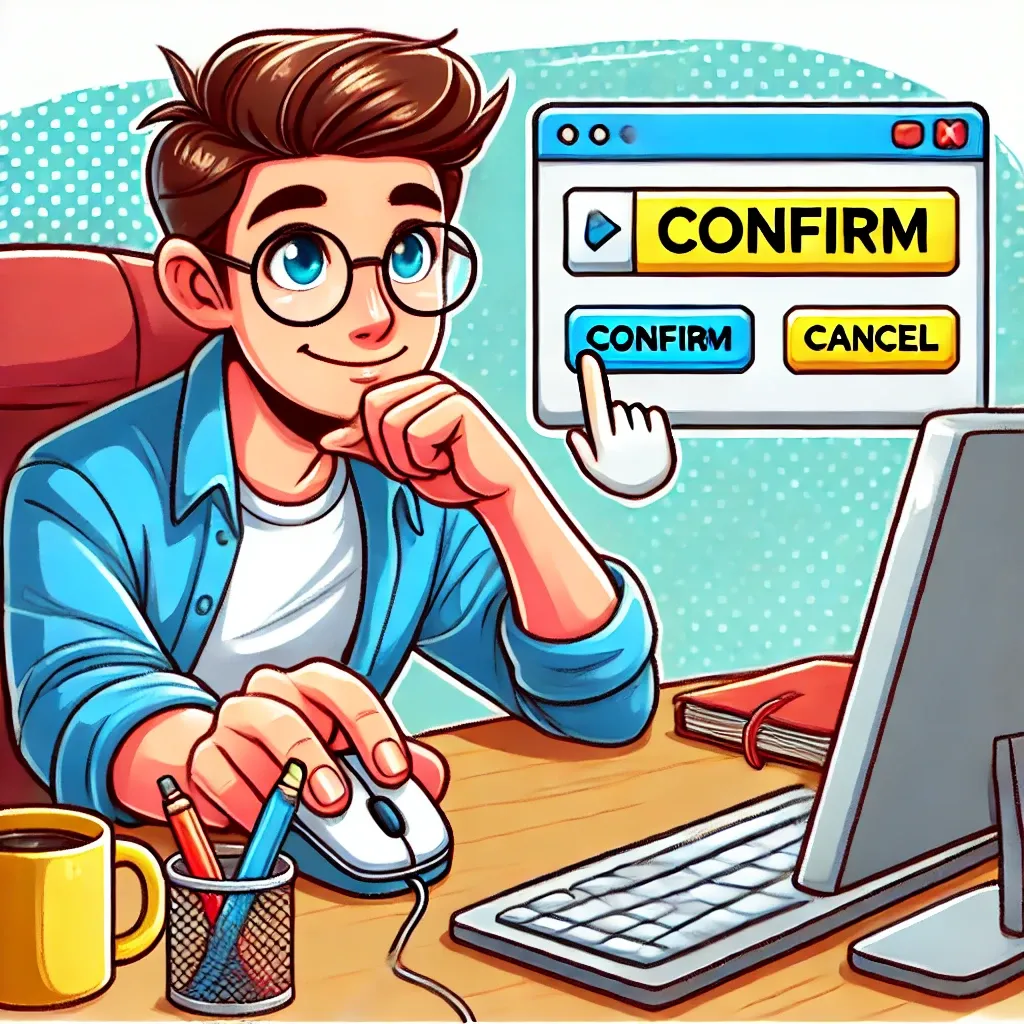
As Livewire developers, we often need to add an extra layer of confirmation before executing critical actions in our applications. Livewire's wire:confirm directive provides an elegant solution to this common requirement. In this post, we'll explore how to use this feature to create more user-friendly and safer interfaces.
Understanding wire:confirm
The wire:confirm directive in Livewire allows you to easily add a confirmation dialog before an action is performed. This is particularly useful for irreversible operations or actions with significant consequences.
Basic Usage
Let's start with a simple example:
<button wire:click="deletePost" wire:confirm="Are you sure you want to delete this post?">
Delete Post
</button>
In this case, when a user clicks the "Delete Post" button, they'll see a browser confirmation dialog with the specified message before the deletePost method is called.
Advanced Techniques
Dynamic Confirmation Messages
You can make your confirmation messages more dynamic by using component properties:
<button wire:click="deletePost({{ $post->id }})"
wire:confirm="Are you sure you want to delete '{{ $post->title }}'?">
Delete Post
</button>
Combining with Other Livewire Features
wire:confirm works well with other Livewire directives:
<button wire:click="submitForm"
wire:loading.attr="disabled"
wire:confirm="This action cannot be undone. Proceed?">
Submit
</button>
This example combines confirmation with loading states.
Real-World Example: User Management System
Let's consider a more comprehensive example of a user management system:
// app/Http/Livewire/UserManagement.php
class UserManagement extends Component
{
public $users;
public function mount()
{
$this->users = User::all();
}
public function deleteUser($userId)
{
$user = User::findOrFail($userId);
$user->delete();
$this->users = User::all();
session()->flash('message', 'User deleted successfully.');
}
public function suspendUser($userId)
{
$user = User::findOrFail($userId);
$user->suspended = true;
$user->save();
$this->users = User::all();
session()->flash('message', 'User suspended successfully.');
}
public function render()
{
return view('livewire.user-management');
}
}
// resources/views/livewire/user-management.blade.php
<div>
@foreach ($users as $user)
<div>
{{ $user->name }}
<button wire:click="deleteUser({{ $user->id }})"
wire:confirm="Are you sure you want to permanently delete {{ $user->name }}?">
Delete
</button>
<button wire:click="suspendUser({{ $user->id }})"
wire:confirm="Are you sure you want to suspend {{ $user->name }}?">
Suspend
</button>
</div>
@endforeach
</div>
In this example, we've added confirmation dialogs for both deleting and suspending users, providing an extra layer of safety for these important actions.
Best Practices
- Clear Messages: Write clear, concise confirmation messages that explain the consequences of the action.
- Context-Specific: Tailor your confirmation messages to the specific action and context.
- Consistent Use: Use confirmations consistently across your application for similar types of actions.
- Avoid Overuse: While confirmations are useful, overusing them can lead to "confirmation fatigue." Reserve them for important or irreversible actions.
- Accessibility: Ensure that your confirmation dialogs are accessible, including for users relying on screen readers.
Customizing the Confirmation Dialog
While wire:confirm uses the browser's default confirmation dialog, you can create custom confirmation modals for a more tailored user experience:
<div x-data="{ showConfirmModal: false }">
<button @click="showConfirmModal = true">Delete User</button>
<div x-show="showConfirmModal" class="modal">
<p>Are you sure you want to delete this user?</p>
<button wire:click="deleteUser" @click="showConfirmModal = false">Yes, Delete</button>
<button @click="showConfirmModal = false">Cancel</button>
</div>
</div>
This approach using Alpine.js allows for more styled and customizable confirmation dialogs.
Conclusion
Livewire's wire:confirm directive provides a simple yet powerful way to add confirmation dialogs to your application's critical actions. By implementing this feature, you can significantly improve the user experience and prevent accidental actions that could have serious consequences.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!