Enhancing User Experience with Livewire's wire:transition.scale
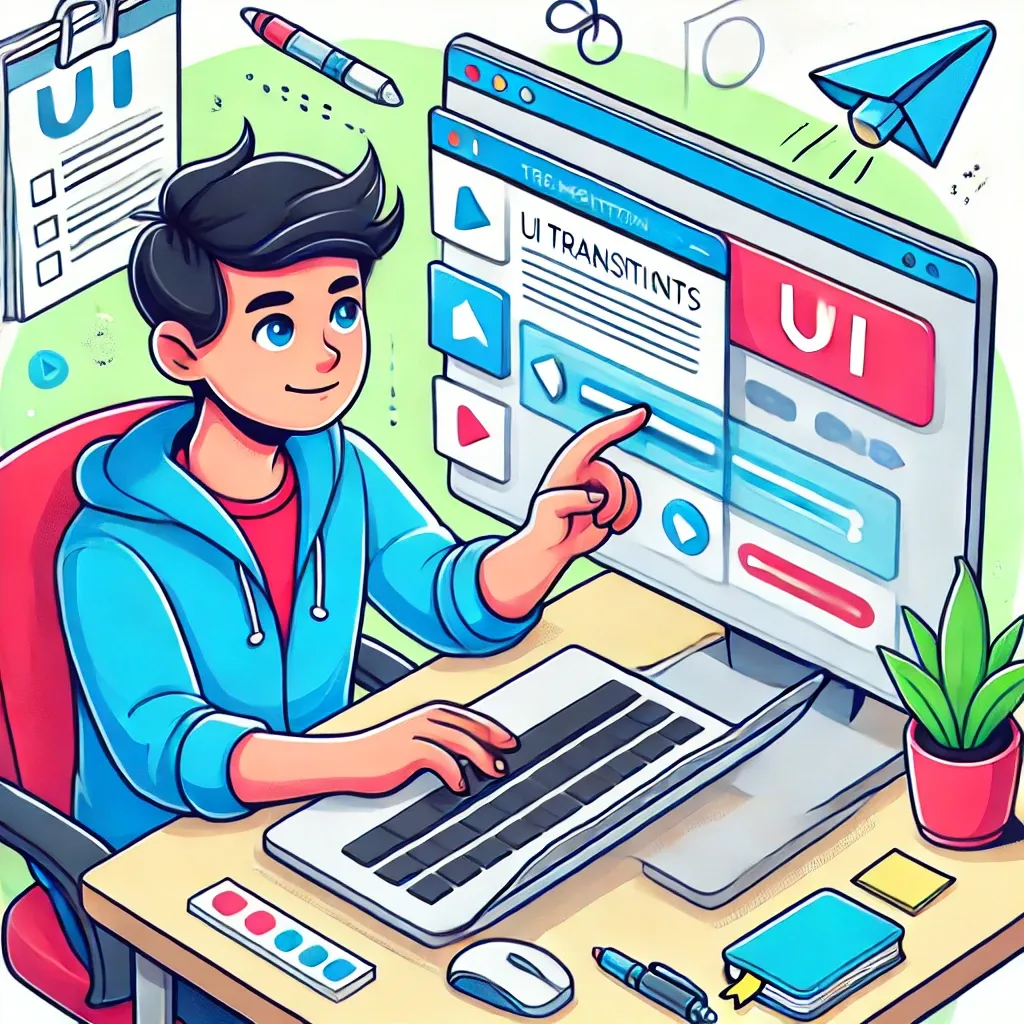
As web developers, we're constantly seeking ways to make our applications more engaging and visually appealing. Laravel Livewire, known for its ability to create dynamic interfaces, offers a powerful feature that can elevate your user interface to the next level: wire:transition.scale. In this post, we'll explore how to use this directive to add smooth scaling transitions to your Livewire components.
What is wire:transition.scale?
wire:transition.scale is a Livewire directive that allows you to add a scaling animation to elements as they enter or leave the DOM. This creates a visually pleasing effect that can significantly enhance the perceived responsiveness and polish of your application.
How to Use wire:transition.scale
Here's a basic example of how to use wire:transition.scale:
// resources/views/livewire/notification.blade.php
<div>
@if($show)
<div wire:transition.scale>
<p>This is an important notification!</p>
</div>
@endif
</div>
// app/Http/Livewire/Notification.php
class Notification extends Component
{
public $show = false;
public function toggleNotification()
{
$this->show = !$this->show;
}
public function render()
{
return view('livewire.notification');
}
}
Let's break this down:
- In the Blade template, we use wire:transition.scale on the div that we want to animate.
- The element will scale in when $show becomes true, and scale out when it becomes false.
- In the Livewire component, we have a simple toggle method to show/hide the notification.
Benefits of Using wire:transition.scale
- Improved User Experience: Smooth animations make your interface feel more responsive and polished.
- Easy Implementation: Add engaging animations without writing complex CSS or JavaScript.
- Consistency: Livewire ensures that the animations are consistent across different parts of your application.
- Performance: The transitions are optimized for performance, ensuring smooth animations even on less powerful devices.
Real-World Example: Dynamic Content Loading
Let's consider a more complex real-world scenario where wire:transition.scale can be incredibly useful: a dynamic content loading system for a blog or news site.
// resources/views/livewire/article-list.blade.php
<div>
@foreach($articles as $article)
<div wire:key="{{ $article->id }}" wire:transition.scale>
<h2>{{ $article->title }}</h2>
<p>{{ $article->excerpt }}</p>
</div>
@endforeach
<button wire:click="loadMore">Load More</button>
</div>
// app/Http/Livewire/ArticleList.php
class ArticleList extends Component
{
public $articles;
public $page = 1;
public function mount()
{
$this->loadArticles();
}
public function loadMore()
{
$this->page++;
$this->loadArticles();
}
private function loadArticles()
{
$newArticles = Article::latest()
->take(10 * $this->page)
->get();
$this->articles = $newArticles;
}
public function render()
{
return view('livewire.article-list');
}
}
In this example:
- We use wire:transition.scale on each article div to create a smooth scaling effect when new articles are loaded.
- The wire:key attribute ensures that Livewire can properly track each article for animation purposes.
- When the "Load More" button is clicked, new articles are fetched and added to the list with a pleasing scale-in animation.
This approach creates a visually engaging experience as users load more content, making the interaction feel smooth and responsive.
Advanced Usage: Customizing the Transition
Livewire also allows you to customize the scale transition. You can adjust the starting scale, duration, and timing function:
<div wire:transition.scale.75>
<!-- This will start at 75% scale -->
</div>
<div wire:transition.scale.duration.500ms>
<!-- This transition will last 500 milliseconds -->
</div>
<div wire:transition.scale.ease-in-out>
<!-- This will use an ease-in-out timing function -->
</div>
These customizations allow you to fine-tune the animation to fit the specific needs and feel of your application.
Conclusion
Livewire's wire:transition.scale directive is a powerful tool for creating engaging, animated user interfaces in your Laravel applications. By allowing you to easily add scaling transitions to your components, it enables you to build more dynamic and visually appealing interfaces with minimal effort.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!