Enhancing User Experience with Custom Validation Messages in Laravel
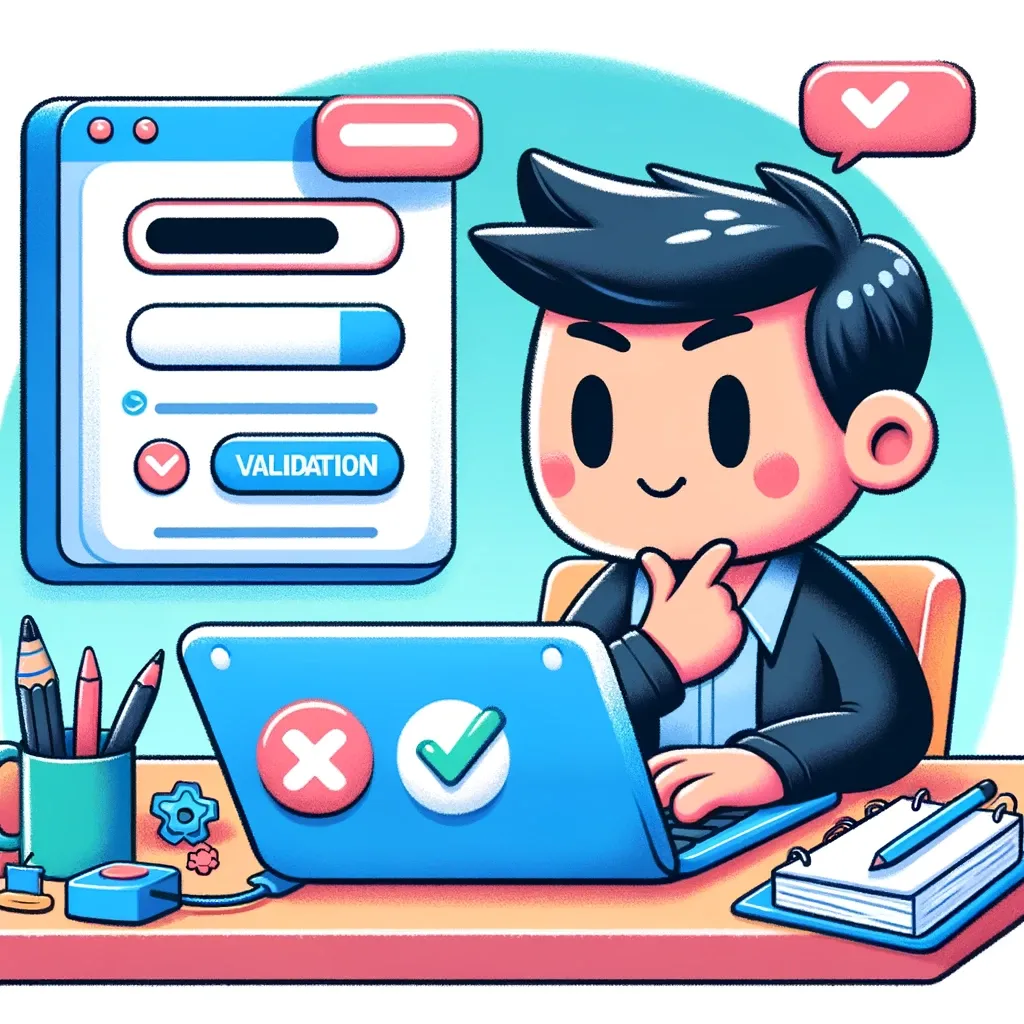
As Laravel developers, we strive to create applications that are not only functional but also user-friendly. One often overlooked aspect of user experience is the wording of validation error messages. Laravel provides powerful tools to customize these messages, allowing us to create more context-specific and user-friendly feedback. In this post, we'll explore how to leverage Laravel's localization features to create custom validation messages.
Why Custom Validation Messages Matter
Default validation messages are often technical and may not provide the best user experience. Custom messages allow you to:
- Use language that your users understand
- Provide more context-specific feedback
- Maintain a consistent tone across your application
- Support multiple languages easily
Basic Custom Messages
Let's start with a basic example of customizing messages:
public function messages()
{
return [
'email.required' => 'We need your email address to contact you.',
'password.min' => 'For your security, please use at least 8 characters in your password.',
];
}
This method can be used in form requests or directly in controllers when calling the validator.
Leveraging Localization
To take full advantage of Laravel's localization features, we can use the trans()
or __()
helpers:
public function messages()
{
return [
'email.required' => trans('validation.custom.email.required'),
'password.min' => __('validation.custom.password.min', ['min' => 8]),
];
}
Then, in your resources/lang/en/validation.php
file:
'custom' => [
'email' => [
'required' => 'We need your email address to contact you.',
],
'password' => [
'min' => 'For your security, please use at least :min characters in your password.',
],
],
This approach allows for easy localization and keeps all your messages organized in language files.
Advanced Techniques
Using Closures for Dynamic Messages
You can use closures to generate dynamic error messages:
public function messages()
{
return [
'username.unique' => function ($message, $attribute, $rule, $parameters) {
return 'The username "' . request('username') . '" is already taken. How about ' . request('username') . rand(1, 100) . '?';
},
];
}
Attribute-Specific Custom Messages
You can specify custom attribute names to make your messages even more friendly:
public function attributes()
{
return [
'email' => 'email address',
'phone' => 'phone number',
];
}
Now, a message like "The email is required" would become "The email address is required".
Real-World Example: Registration Form
Let's look at a more comprehensive example for a user registration form:
// app/Http/Requests/UserRegistrationRequest.php
class UserRegistrationRequest extends FormRequest
{
public function rules()
{
return [
'name' => 'required|string|max:255',
'email' => 'required|string|email|max:255|unique:users',
'password' => 'required|string|min:8|confirmed',
'terms' => 'accepted',
];
}
public function messages()
{
return [
'name.required' => trans('validation.custom.name.required'),
'email.required' => trans('validation.custom.email.required'),
'email.unique' => trans('validation.custom.email.unique'),
'password.min' => trans('validation.custom.password.min', ['min' => 8]),
'terms.accepted' => trans('validation.custom.terms.accepted'),
];
}
}
// resources/lang/en/validation.php
return [
'custom' => [
'name' => [
'required' => 'Please tell us your name so we can personalize your experience.',
],
'email' => [
'required' => 'We need your email address to create your account.',
'unique' => 'Looks like you already have an account. Would you like to log in instead?',
],
'password' => [
'min' => 'To keep your account secure, please use at least :min characters in your password.',
],
'terms' => [
'accepted' => 'Please review and accept our terms of service to continue.',
],
],
];
Best Practices
- Be Consistent: Maintain a consistent tone and style across all your custom messages.
- Be Specific: Provide clear instructions on how to correct the error.
- Be Positive: Frame messages in a positive, helpful tone rather than a negative one.
- Use Localization: Always use language files to make future updates and translations easier.
- Test with Users: Get feedback on your error messages to ensure they're clear and helpful.
Conclusion
Customizing validation messages is a small touch that can significantly improve the user experience of your Laravel application. By providing clear, friendly, and context-specific feedback, you can guide users through forms more effectively, reducing frustration and improving completion rates. Remember, good error messages are not just about pointing out what's wrong – they're about helping the user succeed.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!