Enhancing Security: Logging Out Users from Other Devices in Laravel
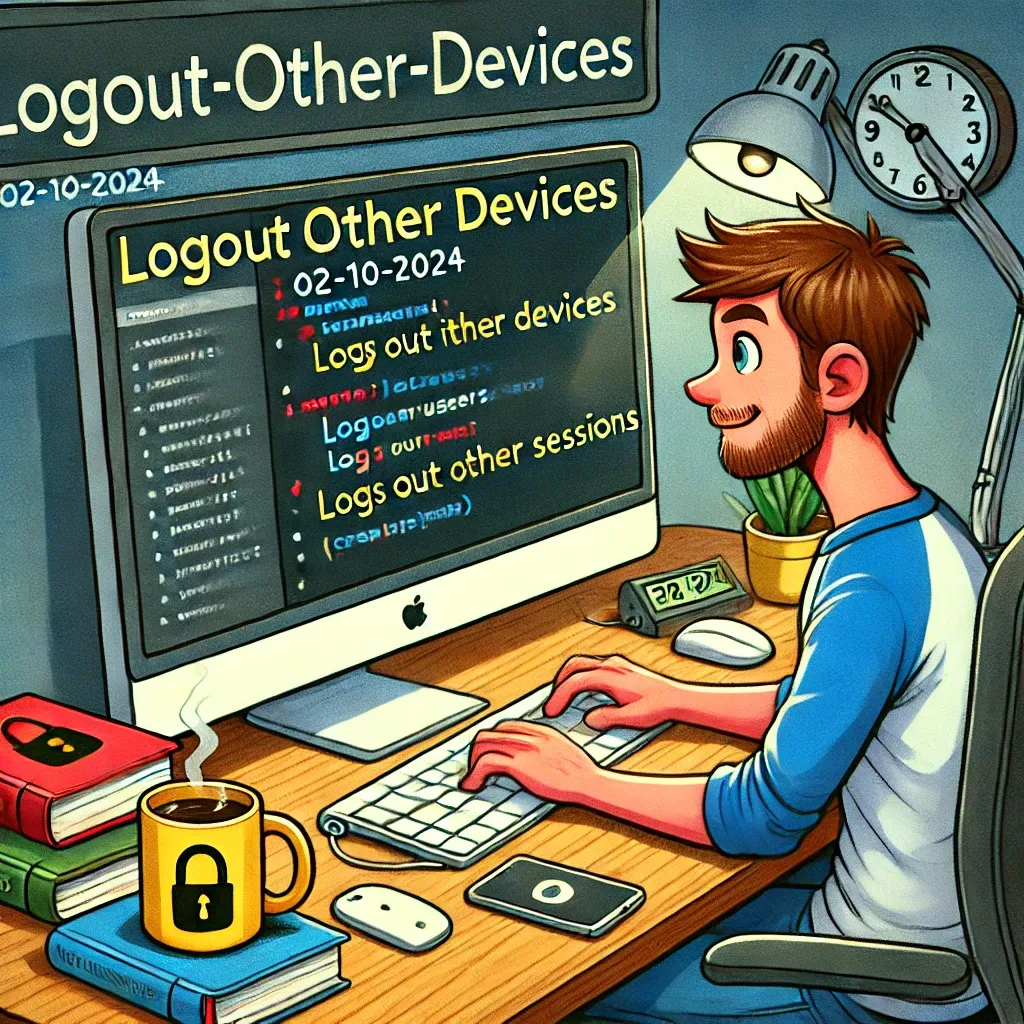
In today's multi-device world, users often access their accounts from various devices. While this convenience is great, it can pose security risks. Laravel provides a powerful feature to mitigate these risks: the ability to log out users from all other devices except the current one. Let's explore how to implement this feature using Auth::logoutOtherDevices()
.
Understanding the Feature
The logoutOtherDevices()
method allows you to invalidate and effectively "log out" a user's sessions on all devices except the one they're currently using. This is particularly useful when a user changes their password or when you suspect a security breach.
Setting Up the Middleware
Before implementing this feature, ensure that you have the AuthenticateSession
middleware in place. This middleware is crucial for maintaining session authentication across your application.
Add the following to your route group definitions:
Route::middleware(['auth', 'auth.session'])->group(function () {
// Your protected routes go here
});
Implementing the Logout Feature
Here's a basic implementation of the logout feature:
use Illuminate\Support\Facades\Auth;
Route::post('/update-password', function (Request $request) {
// Validate the new password
$request->validate([
'current_password' => 'required',
'new_password' => 'required|min:8|confirmed',
]);
// Check the current password
if (!Hash::check($request->current_password, Auth::user()->password)) {
return back()->withErrors(['current_password' => 'The provided password does not match our records.']);
}
// Log out other devices
Auth::logoutOtherDevices($request->current_password);
// Update the password
Auth::user()->update([
'password' => Hash::make($request->new_password)
]);
return redirect('/dashboard')->with('status', 'Password updated and other devices logged out.');
});
How It Works
- The user submits their current password along with the new password.
- We validate the input and check if the current password is correct.
- If everything checks out, we call
Auth::logoutOtherDevices()
with the current password. - Finally, we update the user's password.
Real-World Scenario
Imagine you're building a banking application where security is paramount. You might want to implement this feature in several scenarios:
User-Initiated Security Measure:
Route::post('/security/logout-all', function (Request $request) {
Auth::logoutOtherDevices($request->password);
return back()->with('status', 'You have been logged out from all other devices.');
});
Suspicious Activity Detection:
if (detectSuspiciousActivity()) {
Auth::logoutOtherDevices($request->password);
return redirect('/security-check')->with('warning', 'Suspicious activity detected. Other devices have been logged out.');
}
By implementing the logoutOtherDevices()
feature, you significantly enhance the security of your Laravel application. It provides users with greater control over their account access and helps mitigate risks associated with unauthorized access from forgotten logged-in sessions on other devices.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!