Enhancing Request Handling in Laravel with mergeIfMissing()
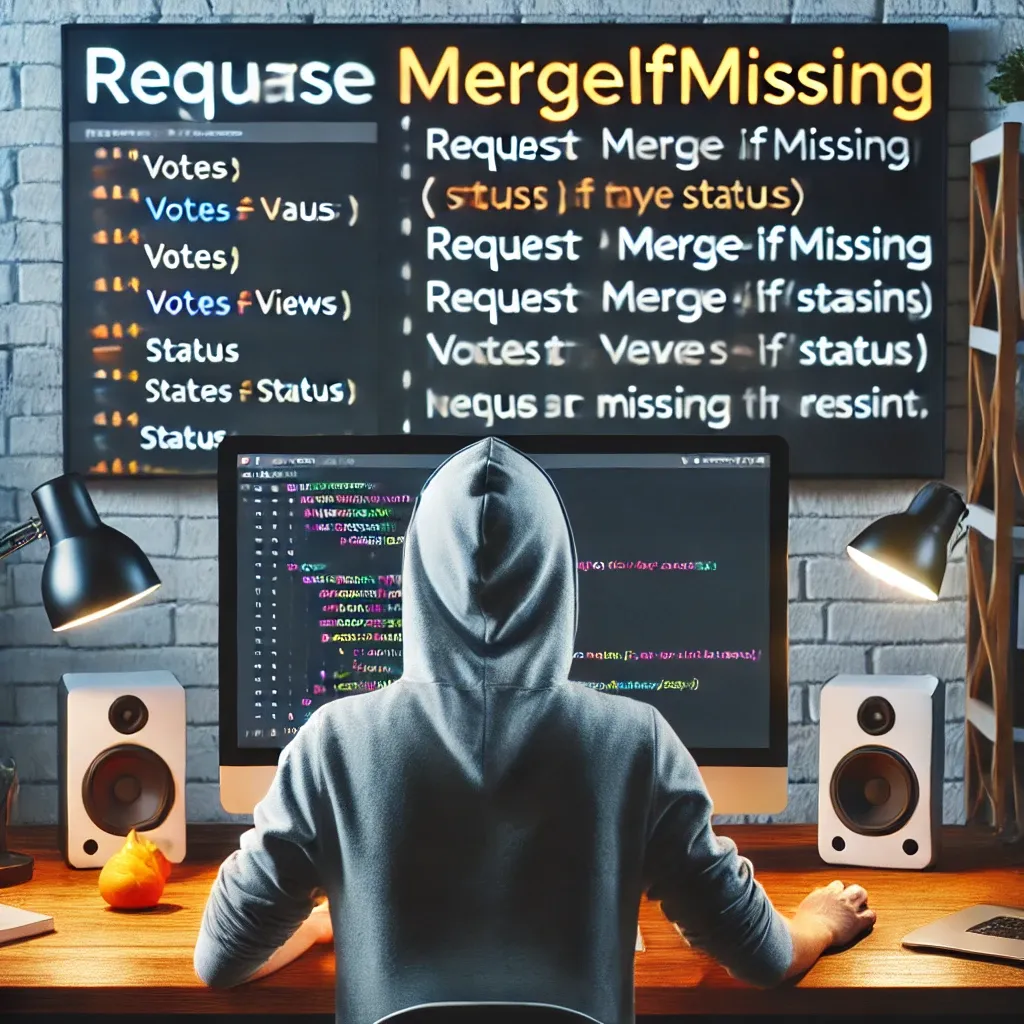
When building web applications, dealing with optional inputs and providing default values is a common task. Laravel, always striving to make developers' lives easier, offers the mergeIfMissing
method on the request object. This method allows you to seamlessly add default values to your request data without overwriting existing values. Let's explore how this feature can streamline your Laravel applications.
Understanding mergeIfMissing()
The mergeIfMissing
method merges the given array into the request's input data, but only for keys that don't already exist in the request. Here's the basic syntax:
$request->mergeIfMissing(['key' => 'default_value']);
Real-Life Example
Let's consider a scenario where we're creating a blog post. We want to ensure that certain fields always have a value, even if they're not provided in the request.
Here's how we might implement this using mergeIfMissing
:
<?php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
public function store(Request $request)
{
$request->mergeIfMissing([
'status' => 'draft',
'views' => 0,
'likes' => 0,
'published_at' => null,
]);
$post = Post::create($request->all());
return response()->json($post, 201);
}
}
In this example, we're using mergeIfMissing
to:
- Set a default status of 'draft' if not provided
- Initialize views and likes to 0 if not set
- Set published_at to null if not provided
Here's what the input and output might look like:
// POST /api/posts
// Input (minimal)
{
"title": "My First Blog Post",
"content": "This is the content of my first blog post."
}
// Output
{
"id": 1,
"title": "My First Blog Post",
"content": "This is the content of my first blog post.",
"status": "draft",
"views": 0,
"likes": 0,
"published_at": null,
"created_at": "2023-06-15T18:30:00.000000Z",
"updated_at": "2023-06-15T18:30:00.000000Z"
}
// Input (with some fields set)
{
"title": "My Second Post",
"content": "Content of the second post",
"status": "published",
"published_at": "2023-06-15T18:35:00.000000Z"
}
// Output
{
"id": 2,
"title": "My Second Post",
"content": "Content of the second post",
"status": "published",
"views": 0,
"likes": 0,
"published_at": "2023-06-15T18:35:00.000000Z",
"created_at": "2023-06-15T18:35:00.000000Z",
"updated_at": "2023-06-15T18:35:00.000000Z"
}
By leveraging mergeIfMissing()
, you can create more robust and flexible input handling in your Laravel applications. This method is particularly useful when dealing with forms or APIs where certain fields might be optional, but you want to ensure consistent data structure in your application.
If you found this tip helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel gems!