Mastering Laravel's Request Merge Feature
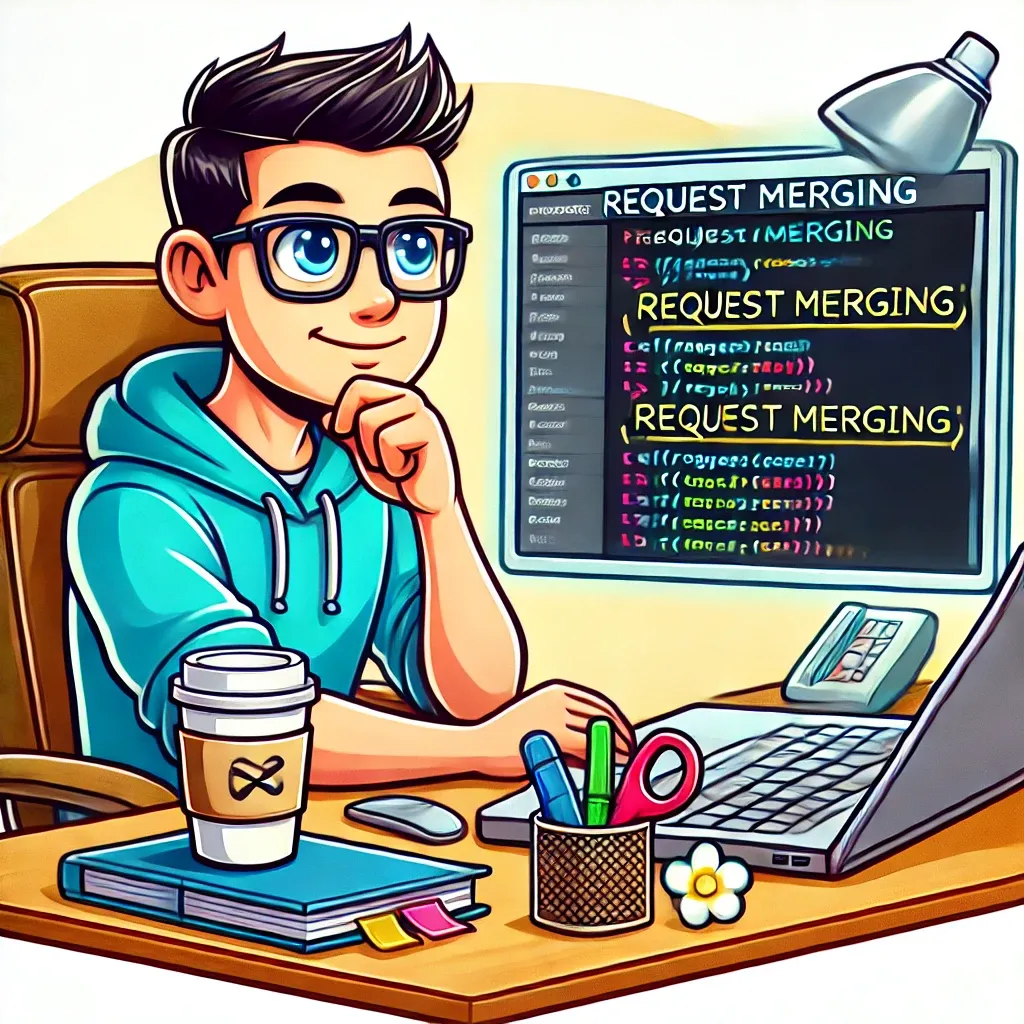
In Laravel applications, manipulating incoming request data is a common task. Whether you're adding user IDs, timestamps, or computed values, Laravel's request merge feature provides a clean and efficient way to enhance your request data. Let's explore how to leverage this powerful feature in your Laravel projects.
Understanding Request Merge
The merge()
method in Laravel allows you to add additional data to the current request. This can be incredibly useful for appending information that isn't directly provided by the client but is necessary for processing the request.
Basic Usage
Here's a simple example of how to use merge()
:
public function store(Request $request)
{
$request->merge(['user_id' => auth()->id()]);
// Now $request->user_id is available
$post = Post::create($request->all());
}
In this example, we're adding the authenticated user's ID to the request data before creating a new post.
Using Merge in Middleware
One of the most powerful applications of merge()
is in middleware, allowing you to consistently add data to requests across multiple routes:
namespace App\Http\Middleware;
use Closure;
class AddUserIdToRequest
{
public function handle($request, Closure $next)
{
if (auth()->check()) {
$request->merge(['user_id' => auth()->id()]);
}
return $next($request);
}
}
Register this middleware in your app/Http/Kernel.php
:
protected $routeMiddleware = [
// ...
'add.userid' => \App\Http\Middleware\AddUserIdToRequest::class,
];
Now you can use it on routes or in controllers:
Route::post('/posts', [PostController::class, 'store'])->middleware('add.userid');
Advanced Usage
Merging Computed Values
You can merge computed values based on other request data:
public function calculateDiscount(Request $request)
{
$subtotal = $request->input('subtotal');
$discountRate = $this->getDiscountRate($subtotal);
$request->merge([
'discount' => $subtotal * $discountRate,
'discount_rate' => $discountRate,
]);
// Process the order with the calculated discount
}
Conditional Merging
You can conditionally merge data based on certain criteria:
public function processPayment(Request $request)
{
if ($request->has('use_saved_card') && $request->use_saved_card) {
$savedCard = auth()->user()->defaultCard;
$request->merge([
'card_token' => $savedCard->token,
'card_last_four' => $savedCard->last_four,
]);
}
// Process payment
}
Real-World Example: E-commerce Order Processing
Let's look at a more comprehensive example in the context of an e-commerce application:
namespace App\Http\Controllers;
use App\Models\Order;
use Illuminate\Http\Request;
class OrderController extends Controller
{
public function store(Request $request)
{
// Add user ID and IP address
$request->merge([
'user_id' => auth()->id(),
'ip_address' => $request->ip(),
]);
// Calculate totals
$subtotal = $this->calculateSubtotal($request->items);
$tax = $this->calculateTax($subtotal);
$total = $subtotal + $tax;
$request->merge([
'subtotal' => $subtotal,
'tax' => $tax,
'total' => $total,
]);
// If using a promo code, add discount info
if ($request->has('promo_code')) {
$discount = $this->applyPromoCode($request->promo_code, $subtotal);
$request->merge([
'discount' => $discount,
'total' => $total - $discount,
]);
}
// Create the order
$order = Order::create($request->all());
return response()->json($order, 201);
}
// Helper methods (calculateSubtotal, calculateTax, applyPromoCode) would be defined here
}
In this example, we're enriching the request with various computed values before creating the order, ensuring all necessary data is present and correctly calculated.
Laravel's request merge feature provides a powerful way to enhance and manipulate incoming request data. Whether you're adding user context, calculating values, or preparing data for database operations, merge()
offers a clean and efficient solution. By leveraging this feature effectively, you can create more robust, secure, and efficient Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!